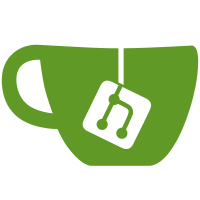
add: 词法分析器剩下数字、标识符的细节处理以及错误处理 Co-authored-by: duqoo <92306417+duqoo@users.noreply.github.com> Reviewed-on: PostGuard/Canon#15 Co-authored-by: Huaps <1183155719@qq.com> Co-committed-by: Huaps <1183155719@qq.com>
59 lines
2.5 KiB
C#
59 lines
2.5 KiB
C#
using Canon.Core.Enums;
|
|
using Canon.Core.LexicalParser;
|
|
using Canon.Core.Exceptions;
|
|
using Xunit.Abstractions;
|
|
|
|
namespace Canon.Tests.LexicalParserTests
|
|
{
|
|
|
|
public class NumberTests
|
|
{
|
|
private readonly ITestOutputHelper _testOutputHelper;
|
|
public NumberTests(ITestOutputHelper testOutputHelper)
|
|
{
|
|
_testOutputHelper = testOutputHelper;
|
|
}
|
|
|
|
[Theory]
|
|
[InlineData("123", "123", NumberType.Integer)]
|
|
[InlineData("0", "0", NumberType.Integer)]
|
|
[InlineData("1.23", "1.23", NumberType.Real)]
|
|
[InlineData("0.0", "0.0", NumberType.Real)]
|
|
[InlineData("1e7", "1e7", NumberType.Real)]
|
|
[InlineData("1E7", "1E7", NumberType.Real)]
|
|
[InlineData("1.23e-7", "1.23e-7", NumberType.Real)]
|
|
[InlineData("1.23E-7", "1.23E-7", NumberType.Real)]
|
|
[InlineData("1234567890", "1234567890", NumberType.Integer)]
|
|
[InlineData("1234567890.1234567890", "1234567890.1234567890", NumberType.Real)]
|
|
[InlineData("1e-7", "1e-7", NumberType.Real)]
|
|
[InlineData("1E-7", "1E-7", NumberType.Real)]
|
|
[InlineData(".67",".67", NumberType.Real)]
|
|
[InlineData("$123", "0x123", NumberType.Hex)]
|
|
public void TestParseNumber(string input, string expected, NumberType expectedNumberType)
|
|
{
|
|
Lexer lexer = new(input);
|
|
List<SemanticToken> tokens = lexer.Tokenize();
|
|
SemanticToken token = tokens[0];
|
|
Assert.Equal(SemanticTokenType.Number, token.TokenType);
|
|
NumberSemanticToken numberSemanticToken = (NumberSemanticToken)token;
|
|
Assert.Equal(expectedNumberType, numberSemanticToken.NumberType);
|
|
Assert.Equal(expected, numberSemanticToken.LiteralValue);
|
|
}
|
|
|
|
[Theory]
|
|
[InlineData("1E", 1, 3, LexemeErrorType.IllegalNumberFormat)]
|
|
[InlineData("123abc", 1, 4, LexemeErrorType.IllegalNumberFormat)]
|
|
[InlineData("123.45.67", 1, 7, LexemeErrorType.IllegalNumberFormat)]
|
|
[InlineData("123identifier", 1, 4, LexemeErrorType.IllegalNumberFormat)]
|
|
public void TestParseNumberError(string input, uint expectedLine, uint expectedCharPosition, LexemeErrorType expectedErrorType)
|
|
{
|
|
Lexer lexer = new(input);
|
|
var ex = Assert.Throws<LexemeException>(() => lexer.Tokenize());
|
|
_testOutputHelper.WriteLine(ex.ToString());
|
|
Assert.Equal(expectedErrorType, ex.ErrorType);
|
|
Assert.Equal(expectedLine, ex.Line);
|
|
Assert.Equal(expectedCharPosition, ex.CharPosition);
|
|
}
|
|
}
|
|
}
|