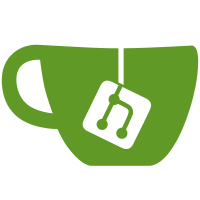
地址已绑定编译结果,支持历史记录切换功能 Co-authored-by: jackfiled <xcrenchangjun@outlook.com> Reviewed-on: PostGuard/Canon#51 Co-authored-by: Ichirinko <1621543655@qq.com> Co-committed-by: Ichirinko <1621543655@qq.com>
663 lines
578 KiB
C#
663 lines
578 KiB
C#
#nullable enable
|
|
using Canon.Core.Abstractions;
|
|
using Canon.Core.GrammarParser;
|
|
using Canon.Core.Enums;
|
|
namespace Canon.Core.GrammarParser;
|
|
|
|
public class GeneratedTransformer : ITransformer
|
|
{
|
|
private IDictionary<TerminatorBase, string> _shiftPointers;
|
|
|
|
public string Name { get; }
|
|
|
|
public IDictionary<Terminator, ReduceInformation> ReduceTable { get; }
|
|
|
|
public IDictionary<TerminatorBase, ITransformer> ShiftTable { get; }
|
|
|
|
public GeneratedTransformer(Dictionary<TerminatorBase, string> shiftTable,
|
|
Dictionary<Terminator, ReduceInformation> reduceTable, string name)
|
|
{
|
|
ReduceTable = reduceTable;
|
|
ShiftTable = new Dictionary<TerminatorBase, ITransformer>();
|
|
_shiftPointers = shiftTable;
|
|
Name = name;
|
|
}
|
|
|
|
public GeneratedTransformer()
|
|
{
|
|
ReduceTable = new Dictionary<Terminator, ReduceInformation>();
|
|
ShiftTable = new Dictionary<TerminatorBase, ITransformer>();
|
|
_shiftPointers = new Dictionary<TerminatorBase, string>();
|
|
Name = Guid.NewGuid().ToString();
|
|
}
|
|
|
|
public void ConstructShiftTable(Dictionary<string, GeneratedTransformer> transformers)
|
|
{
|
|
foreach (KeyValuePair<TerminatorBase,string> pair in _shiftPointers)
|
|
{
|
|
ShiftTable.Add(pair.Key, transformers[pair.Value]);
|
|
}
|
|
}
|
|
|
|
public override bool Equals(object? obj)
|
|
{
|
|
if (obj is not GeneratedTransformer other)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
return Name == other.Name;
|
|
}
|
|
|
|
public override int GetHashCode() => Name.GetHashCode();
|
|
}
|
|
public class GeneratedGrammarParser : IGrammarParser
|
|
{
|
|
private static readonly Dictionary<string, GeneratedTransformer> s_transformers = new()
|
|
{
|
|
{ "0ec30c00-e066-4e5e-a8b5-d0b86ce475ff", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ProgramStruct), "9795b29a-fcc6-4e7f-a720-aae07bdf7621"}, { new NonTerminator(NonTerminatorType.ProgramHead), "337185aa-d780-4d34-bfa1-9e9e97ae08d1"}, { new Terminator(KeywordType.Program), "5851bab3-2723-441a-a174-a9bd16cfa2c8"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0ec30c00-e066-4e5e-a8b5-d0b86ce475ff") },
|
|
{ "9795b29a-fcc6-4e7f-a720-aae07bdf7621", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.EndTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.StartNonTerminator))}, }, "9795b29a-fcc6-4e7f-a720-aae07bdf7621") },
|
|
{ "337185aa-d780-4d34-bfa1-9e9e97ae08d1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Semicolon), "4de6a540-2418-495d-81d0-93218c548f55"},}, new Dictionary<Terminator, ReduceInformation>{ }, "337185aa-d780-4d34-bfa1-9e9e97ae08d1") },
|
|
{ "5851bab3-2723-441a-a174-a9bd16cfa2c8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "43d4a173-9bfe-4556-b93f-bda2864df3d3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5851bab3-2723-441a-a174-a9bd16cfa2c8") },
|
|
{ "4de6a540-2418-495d-81d0-93218c548f55", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ProgramBody), "3d85ae4e-130a-4c9a-ad16-9c68fe9ee4f9"}, { new NonTerminator(NonTerminatorType.ConstDeclarations), "81e37fc8-8ebd-4d8d-a9a3-1256ada33bee"}, { new Terminator(KeywordType.Const), "ae34b16b-4829-44dd-b662-b35d2012b333"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Procedure), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Function), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Var), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ConstDeclarations))}, }, "4de6a540-2418-495d-81d0-93218c548f55") },
|
|
{ "43d4a173-9bfe-4556-b93f-bda2864df3d3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "e3f96aa7-a1f5-4bfa-87cd-2ff0270885b1"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ProgramHead))}, }, "43d4a173-9bfe-4556-b93f-bda2864df3d3") },
|
|
{ "3d85ae4e-130a-4c9a-ad16-9c68fe9ee4f9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Period), "9786cddc-9558-4fb4-8428-d6aed42c5a0d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "3d85ae4e-130a-4c9a-ad16-9c68fe9ee4f9") },
|
|
{ "81e37fc8-8ebd-4d8d-a9a3-1256ada33bee", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.VarDeclarations), "d1a1b822-ae15-42ca-a6b2-88270e26ca92"}, { new Terminator(KeywordType.Var), "9e1607f7-45af-4833-a5f8-867c3b650cdd"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(0, new NonTerminator(NonTerminatorType.VarDeclarations))}, { new Terminator(KeywordType.Procedure), new ReduceInformation(0, new NonTerminator(NonTerminatorType.VarDeclarations))}, { new Terminator(KeywordType.Function), new ReduceInformation(0, new NonTerminator(NonTerminatorType.VarDeclarations))}, }, "81e37fc8-8ebd-4d8d-a9a3-1256ada33bee") },
|
|
{ "ae34b16b-4829-44dd-b662-b35d2012b333", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ConstDeclaration), "52b20a41-14fb-4046-a340-9c65019e0106"}, { Terminator.IdentifierTerminator, "350f5711-3321-4fce-ad22-19bd472eae37"},}, new Dictionary<Terminator, ReduceInformation>{ }, "ae34b16b-4829-44dd-b662-b35d2012b333") },
|
|
{ "e3f96aa7-a1f5-4bfa-87cd-2ff0270885b1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.IdentifierList), "b709cf6e-42dd-4476-9f76-ae8fda9e9250"}, { Terminator.IdentifierTerminator, "b1baea6f-eb7e-48fa-9a23-b3b82272a36d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e3f96aa7-a1f5-4bfa-87cd-2ff0270885b1") },
|
|
{ "9786cddc-9558-4fb4-8428-d6aed42c5a0d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.EndTerminator, new ReduceInformation(4, new NonTerminator(NonTerminatorType.ProgramStruct))}, }, "9786cddc-9558-4fb4-8428-d6aed42c5a0d") },
|
|
{ "d1a1b822-ae15-42ca-a6b2-88270e26ca92", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SubprogramDeclarations), "64e85429-b687-4fea-858f-9b6bd1c92dfb"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(0, new NonTerminator(NonTerminatorType.SubprogramDeclarations))}, { new Terminator(KeywordType.Procedure), new ReduceInformation(0, new NonTerminator(NonTerminatorType.SubprogramDeclarations))}, { new Terminator(KeywordType.Function), new ReduceInformation(0, new NonTerminator(NonTerminatorType.SubprogramDeclarations))}, }, "d1a1b822-ae15-42ca-a6b2-88270e26ca92") },
|
|
{ "9e1607f7-45af-4833-a5f8-867c3b650cdd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.VarDeclaration), "21a2bd46-32e2-4a45-8c6d-a75efdce5c6b"}, { new NonTerminator(NonTerminatorType.IdentifierList), "153fdd10-03fc-4d0b-8b71-de6c4a119383"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ }, "9e1607f7-45af-4833-a5f8-867c3b650cdd") },
|
|
{ "52b20a41-14fb-4046-a340-9c65019e0106", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Semicolon), "cbf35baf-a1be-45eb-bf50-e2b314cb2131"},}, new Dictionary<Terminator, ReduceInformation>{ }, "52b20a41-14fb-4046-a340-9c65019e0106") },
|
|
{ "350f5711-3321-4fce-ad22-19bd472eae37", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Equal), "edbc9e45-8b9f-452b-9ae9-98e91f134f94"},}, new Dictionary<Terminator, ReduceInformation>{ }, "350f5711-3321-4fce-ad22-19bd472eae37") },
|
|
{ "b709cf6e-42dd-4476-9f76-ae8fda9e9250", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "6876a0f1-eb19-4109-8bfc-c5171039b872"}, { new Terminator(DelimiterType.Comma), "1939a028-1ce8-463b-b974-dcbe2c008359"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b709cf6e-42dd-4476-9f76-ae8fda9e9250") },
|
|
{ "b1baea6f-eb7e-48fa-9a23-b3b82272a36d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.IdentifierList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.IdentifierList))}, }, "b1baea6f-eb7e-48fa-9a23-b3b82272a36d") },
|
|
{ "64e85429-b687-4fea-858f-9b6bd1c92dfb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.CompoundStatement), "0a3899b4-dc42-4d86-96e3-0f2e27f2f8ac"}, { new Terminator(KeywordType.Begin), "3e8a151e-b996-437e-8586-1444c89a5e3d"}, { new NonTerminator(NonTerminatorType.Subprogram), "d1ea05d3-9b46-4939-852d-5542dad9b5e7"}, { new NonTerminator(NonTerminatorType.SubprogramHead), "b5a53feb-3b0a-49a2-ae21-36c57e8e74ed"}, { new Terminator(KeywordType.Procedure), "0220f031-d1ff-400c-9dfb-ea3581530740"}, { new Terminator(KeywordType.Function), "e83802c9-2612-4dab-b7c1-ee8774ea5801"},}, new Dictionary<Terminator, ReduceInformation>{ }, "64e85429-b687-4fea-858f-9b6bd1c92dfb") },
|
|
{ "21a2bd46-32e2-4a45-8c6d-a75efdce5c6b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Semicolon), "a1e6947e-90e9-4c44-8b6c-98492e400d3f"},}, new Dictionary<Terminator, ReduceInformation>{ }, "21a2bd46-32e2-4a45-8c6d-a75efdce5c6b") },
|
|
{ "153fdd10-03fc-4d0b-8b71-de6c4a119383", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Colon), "2ba6c335-667b-4644-a3ca-eec89c8ef328"}, { new Terminator(DelimiterType.Comma), "f780d1df-5e94-4b1b-ba7d-0107db38c3b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "153fdd10-03fc-4d0b-8b71-de6c4a119383") },
|
|
{ "1620bbb5-297a-47ec-97c7-4d1826507adf", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Colon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.IdentifierList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.IdentifierList))}, }, "1620bbb5-297a-47ec-97c7-4d1826507adf") },
|
|
{ "cbf35baf-a1be-45eb-bf50-e2b314cb2131", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "6f006c54-98dd-4024-a4cc-116e8e209e5c"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Procedure), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Function), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Var), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ConstDeclarations))}, }, "cbf35baf-a1be-45eb-bf50-e2b314cb2131") },
|
|
{ "edbc9e45-8b9f-452b-9ae9-98e91f134f94", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ConstValue), "5110cba0-c5b2-4df9-b8ce-5aba59cb716c"}, { new Terminator(OperatorType.Plus), "8b01dfd8-4b50-461b-b60a-c569310f3705"}, { new Terminator(OperatorType.Minus), "eb9e895c-18b0-4462-9bbd-899eb7ef592c"}, { Terminator.NumberTerminator, "ff5ed887-e52d-4807-a00a-50cf806f198a"}, { Terminator.CharacterTerminator, "1122485e-8861-4faf-bee9-de4048a0066d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "edbc9e45-8b9f-452b-9ae9-98e91f134f94") },
|
|
{ "6876a0f1-eb19-4109-8bfc-c5171039b872", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(5, new NonTerminator(NonTerminatorType.ProgramHead))}, }, "6876a0f1-eb19-4109-8bfc-c5171039b872") },
|
|
{ "1939a028-1ce8-463b-b974-dcbe2c008359", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "72c919c0-9bf2-4f9b-ac07-ba0e3f8222e9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1939a028-1ce8-463b-b974-dcbe2c008359") },
|
|
{ "0a3899b4-dc42-4d86-96e3-0f2e27f2f8ac", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Period), new ReduceInformation(4, new NonTerminator(NonTerminatorType.ProgramBody))}, }, "0a3899b4-dc42-4d86-96e3-0f2e27f2f8ac") },
|
|
{ "3e8a151e-b996-437e-8586-1444c89a5e3d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.StatementList), "1d03d0e7-df08-43b8-a706-eaad6ca9d700"}, { new NonTerminator(NonTerminatorType.Statement), "2dac9c20-107b-46e4-a18b-fdf58e625107"}, { new NonTerminator(NonTerminatorType.Variable), "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1"}, { Terminator.IdentifierTerminator, "2103d893-c079-4b22-81d8-4f9f358fe6ad"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "e53241b0-bb50-48d3-845a-063d9ecbbdeb"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a"}, { new Terminator(KeywordType.If), "c9145487-1b31-498c-9722-1f68bba31eab"}, { new Terminator(KeywordType.For), "d93a638a-474b-4cbd-93db-107fb792750f"}, { new Terminator(KeywordType.Begin), "bbe1d91e-d0c2-4be2-8184-4548587afa0e"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "3e8a151e-b996-437e-8586-1444c89a5e3d") },
|
|
{ "d1ea05d3-9b46-4939-852d-5542dad9b5e7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Semicolon), "d42c2be6-2a3f-4bf9-8ee8-79a37de8a5d2"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d1ea05d3-9b46-4939-852d-5542dad9b5e7") },
|
|
{ "b5a53feb-3b0a-49a2-ae21-36c57e8e74ed", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Semicolon), "42906d05-ffb1-4db1-83e4-5fb9b6bdc021"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b5a53feb-3b0a-49a2-ae21-36c57e8e74ed") },
|
|
{ "0220f031-d1ff-400c-9dfb-ea3581530740", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "78eb4c49-0782-42ba-bb9b-83db673ed092"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0220f031-d1ff-400c-9dfb-ea3581530740") },
|
|
{ "e83802c9-2612-4dab-b7c1-ee8774ea5801", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "58200c96-3cb6-440d-9e87-5cccf68dac85"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e83802c9-2612-4dab-b7c1-ee8774ea5801") },
|
|
{ "a1e6947e-90e9-4c44-8b6c-98492e400d3f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.IdentifierList), "14a84860-7a46-4421-bb73-a5dda0faf29b"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(3, new NonTerminator(NonTerminatorType.VarDeclarations))}, { new Terminator(KeywordType.Procedure), new ReduceInformation(3, new NonTerminator(NonTerminatorType.VarDeclarations))}, { new Terminator(KeywordType.Function), new ReduceInformation(3, new NonTerminator(NonTerminatorType.VarDeclarations))}, }, "a1e6947e-90e9-4c44-8b6c-98492e400d3f") },
|
|
{ "2ba6c335-667b-4644-a3ca-eec89c8ef328", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Type), "5bf65515-ca73-454a-8402-6c8d9ce74779"}, { new NonTerminator(NonTerminatorType.BasicType), "e587c3e6-05fc-4f92-913a-fb606c8f01a2"}, { new Terminator(KeywordType.Array), "a28b508d-ea6e-47b9-9173-9140b1c7c748"}, { new Terminator(KeywordType.Integer), "d649770f-216c-448b-9cbe-ba827526a2f5"}, { new Terminator(KeywordType.Real), "741f0bed-843f-4135-90f4-4fa0337a7126"}, { new Terminator(KeywordType.Boolean), "981256c3-20fb-44ce-b5ab-9e3a26a98417"}, { new Terminator(KeywordType.Character), "e12136f0-98e7-4894-abb2-8671ea2331c7"},}, new Dictionary<Terminator, ReduceInformation>{ }, "2ba6c335-667b-4644-a3ca-eec89c8ef328") },
|
|
{ "f780d1df-5e94-4b1b-ba7d-0107db38c3b3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "c7bd9d65-3568-48c1-a86c-e10927f82c3f"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f780d1df-5e94-4b1b-ba7d-0107db38c3b3") },
|
|
{ "6f006c54-98dd-4024-a4cc-116e8e209e5c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Equal), "8cfeb9a2-81b3-4c96-8635-6cd5c4092895"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6f006c54-98dd-4024-a4cc-116e8e209e5c") },
|
|
{ "5110cba0-c5b2-4df9-b8ce-5aba59cb716c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ConstDeclaration))}, }, "5110cba0-c5b2-4df9-b8ce-5aba59cb716c") },
|
|
{ "8b01dfd8-4b50-461b-b60a-c569310f3705", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.NumberTerminator, "21b40186-1871-4a41-853c-5d6d7863574b"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8b01dfd8-4b50-461b-b60a-c569310f3705") },
|
|
{ "eb9e895c-18b0-4462-9bbd-899eb7ef592c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.NumberTerminator, "b41c02e5-a0ff-44b9-b431-c2694c5715fc"},}, new Dictionary<Terminator, ReduceInformation>{ }, "eb9e895c-18b0-4462-9bbd-899eb7ef592c") },
|
|
{ "ff5ed887-e52d-4807-a00a-50cf806f198a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ConstValue))}, }, "ff5ed887-e52d-4807-a00a-50cf806f198a") },
|
|
{ "1122485e-8861-4faf-bee9-de4048a0066d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ConstValue))}, }, "1122485e-8861-4faf-bee9-de4048a0066d") },
|
|
{ "72c919c0-9bf2-4f9b-ac07-ba0e3f8222e9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdentifierList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdentifierList))}, }, "72c919c0-9bf2-4f9b-ac07-ba0e3f8222e9") },
|
|
{ "1d03d0e7-df08-43b8-a706-eaad6ca9d700", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.End), "7e451eed-0526-4778-be26-c9d6280a5d65"}, { new Terminator(DelimiterType.Semicolon), "ca1783b9-fb61-4fbb-886b-0d1de39ddf7d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1d03d0e7-df08-43b8-a706-eaad6ca9d700") },
|
|
{ "2dac9c20-107b-46e4-a18b-fdf58e625107", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.StatementList))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.StatementList))}, }, "2dac9c20-107b-46e4-a18b-fdf58e625107") },
|
|
{ "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Assign), "3c9ad433-96e5-4790-b170-c29c64a9bbec"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1") },
|
|
{ "2103d893-c079-4b22-81d8-4f9f358fe6ad", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Assign), "7598cb46-1c5b-4034-8cbd-9cb24dc6e409"}, { new NonTerminator(NonTerminatorType.IdVarPart), "2938411d-d60b-4928-935e-7341545b4c94"}, { new Terminator(DelimiterType.LeftSquareBracket), "b0d115ad-5000-4eed-b6ed-558743b60e74"}, { new Terminator(DelimiterType.LeftParenthesis), "6db3d982-af0e-485b-bb5d-93457809f8ce"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(OperatorType.Assign), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ProcedureCall))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ProcedureCall))}, }, "2103d893-c079-4b22-81d8-4f9f358fe6ad") },
|
|
{ "e53241b0-bb50-48d3-845a-063d9ecbbdeb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, }, "e53241b0-bb50-48d3-845a-063d9ecbbdeb") },
|
|
{ "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, }, "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a") },
|
|
{ "c9145487-1b31-498c-9722-1f68bba31eab", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "04ea29ff-ee71-4f41-bf51-2d170c53cfef"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "e1d03d71-8bbe-4e20-80bf-217f439b2253"}, { new NonTerminator(NonTerminatorType.Term), "cecc617c-0352-4618-b416-5bad0e13338d"}, { new NonTerminator(NonTerminatorType.Factor), "e94ac37d-28f2-4a98-b3c8-1c69a223c4de"}, { Terminator.NumberTerminator, "160264c5-eb9b-477e-9697-ddb4f34fc45f"}, { new NonTerminator(NonTerminatorType.Variable), "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f"}, { new Terminator(DelimiterType.LeftParenthesis), "64e0a597-aae0-4e32-a4aa-59c45f61fe83"}, { Terminator.IdentifierTerminator, "c1b31f89-e41b-4035-a282-0d4bd13e0c82"}, { new Terminator(KeywordType.Not), "000fa394-e859-4466-a821-0c9da688eb4a"}, { new Terminator(OperatorType.Minus), "c844c091-5a24-42c1-9660-9517d6664fa9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c9145487-1b31-498c-9722-1f68bba31eab") },
|
|
{ "d93a638a-474b-4cbd-93db-107fb792750f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "18431573-a0e7-4653-bcec-8616bbdc4e65"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d93a638a-474b-4cbd-93db-107fb792750f") },
|
|
{ "bbe1d91e-d0c2-4be2-8184-4548587afa0e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.StatementList), "4a89d600-1e9e-4be7-8913-d461810035a9"}, { new NonTerminator(NonTerminatorType.Statement), "2dac9c20-107b-46e4-a18b-fdf58e625107"}, { new NonTerminator(NonTerminatorType.Variable), "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1"}, { Terminator.IdentifierTerminator, "2103d893-c079-4b22-81d8-4f9f358fe6ad"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "e53241b0-bb50-48d3-845a-063d9ecbbdeb"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a"}, { new Terminator(KeywordType.If), "c9145487-1b31-498c-9722-1f68bba31eab"}, { new Terminator(KeywordType.For), "d93a638a-474b-4cbd-93db-107fb792750f"}, { new Terminator(KeywordType.Begin), "bbe1d91e-d0c2-4be2-8184-4548587afa0e"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "bbe1d91e-d0c2-4be2-8184-4548587afa0e") },
|
|
{ "d42c2be6-2a3f-4bf9-8ee8-79a37de8a5d2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SubprogramDeclarations))}, { new Terminator(KeywordType.Procedure), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SubprogramDeclarations))}, { new Terminator(KeywordType.Function), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SubprogramDeclarations))}, }, "d42c2be6-2a3f-4bf9-8ee8-79a37de8a5d2") },
|
|
{ "42906d05-ffb1-4db1-83e4-5fb9b6bdc021", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SubprogramBody), "0e1ee01e-4a5e-4e7f-8522-7997f7388c5e"}, { new NonTerminator(NonTerminatorType.ConstDeclarations), "6913293e-2acd-46e7-a0cd-af7c67791647"}, { new Terminator(KeywordType.Const), "e2eed85e-5218-4ed4-a565-6c36024d21b4"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Var), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ConstDeclarations))}, }, "42906d05-ffb1-4db1-83e4-5fb9b6bdc021") },
|
|
{ "78eb4c49-0782-42ba-bb9b-83db673ed092", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.FormalParameter), "239c34f1-88f7-45aa-b4cc-95e4d83f071e"}, { new Terminator(DelimiterType.LeftParenthesis), "4e57943c-1561-4ee1-a95f-3488069a42a6"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.FormalParameter))}, }, "78eb4c49-0782-42ba-bb9b-83db673ed092") },
|
|
{ "58200c96-3cb6-440d-9e87-5cccf68dac85", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.FormalParameter), "fe35e65a-a27e-43a2-b358-dbb2ba56f3b0"}, { new Terminator(DelimiterType.LeftParenthesis), "eed34ae6-e7a5-4ba9-bfea-04bcc0acde9c"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Colon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.FormalParameter))}, }, "58200c96-3cb6-440d-9e87-5cccf68dac85") },
|
|
{ "14a84860-7a46-4421-bb73-a5dda0faf29b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Colon), "8e2917f5-8b23-4cce-b1c4-e1876734f75f"}, { new Terminator(DelimiterType.Comma), "f780d1df-5e94-4b1b-ba7d-0107db38c3b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "14a84860-7a46-4421-bb73-a5dda0faf29b") },
|
|
{ "5bf65515-ca73-454a-8402-6c8d9ce74779", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.VarDeclaration))}, }, "5bf65515-ca73-454a-8402-6c8d9ce74779") },
|
|
{ "e587c3e6-05fc-4f92-913a-fb606c8f01a2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Type))}, }, "e587c3e6-05fc-4f92-913a-fb606c8f01a2") },
|
|
{ "a28b508d-ea6e-47b9-9173-9140b1c7c748", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftSquareBracket), "b16f1ce8-d147-4fad-977b-875f2d3fce4e"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a28b508d-ea6e-47b9-9173-9140b1c7c748") },
|
|
{ "d649770f-216c-448b-9cbe-ba827526a2f5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "d649770f-216c-448b-9cbe-ba827526a2f5") },
|
|
{ "741f0bed-843f-4135-90f4-4fa0337a7126", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "741f0bed-843f-4135-90f4-4fa0337a7126") },
|
|
{ "981256c3-20fb-44ce-b5ab-9e3a26a98417", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "981256c3-20fb-44ce-b5ab-9e3a26a98417") },
|
|
{ "e12136f0-98e7-4894-abb2-8671ea2331c7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "e12136f0-98e7-4894-abb2-8671ea2331c7") },
|
|
{ "c7bd9d65-3568-48c1-a86c-e10927f82c3f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Colon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdentifierList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdentifierList))}, }, "c7bd9d65-3568-48c1-a86c-e10927f82c3f") },
|
|
{ "8cfeb9a2-81b3-4c96-8635-6cd5c4092895", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ConstValue), "2865ba77-6470-42da-9209-f48055d19ed5"}, { new Terminator(OperatorType.Plus), "8b01dfd8-4b50-461b-b60a-c569310f3705"}, { new Terminator(OperatorType.Minus), "eb9e895c-18b0-4462-9bbd-899eb7ef592c"}, { Terminator.NumberTerminator, "ff5ed887-e52d-4807-a00a-50cf806f198a"}, { Terminator.CharacterTerminator, "1122485e-8861-4faf-bee9-de4048a0066d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8cfeb9a2-81b3-4c96-8635-6cd5c4092895") },
|
|
{ "21b40186-1871-4a41-853c-5d6d7863574b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ConstValue))}, }, "21b40186-1871-4a41-853c-5d6d7863574b") },
|
|
{ "b41c02e5-a0ff-44b9-b431-c2694c5715fc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ConstValue))}, }, "b41c02e5-a0ff-44b9-b431-c2694c5715fc") },
|
|
{ "7e451eed-0526-4778-be26-c9d6280a5d65", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Period), new ReduceInformation(3, new NonTerminator(NonTerminatorType.CompoundStatement))}, }, "7e451eed-0526-4778-be26-c9d6280a5d65") },
|
|
{ "ca1783b9-fb61-4fbb-886b-0d1de39ddf7d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Statement), "7a1b40a4-b0ab-401a-8499-4b4b4a572c5d"}, { new NonTerminator(NonTerminatorType.Variable), "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1"}, { Terminator.IdentifierTerminator, "2103d893-c079-4b22-81d8-4f9f358fe6ad"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "e53241b0-bb50-48d3-845a-063d9ecbbdeb"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a"}, { new Terminator(KeywordType.If), "c9145487-1b31-498c-9722-1f68bba31eab"}, { new Terminator(KeywordType.For), "d93a638a-474b-4cbd-93db-107fb792750f"}, { new Terminator(KeywordType.Begin), "bbe1d91e-d0c2-4be2-8184-4548587afa0e"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "ca1783b9-fb61-4fbb-886b-0d1de39ddf7d") },
|
|
{ "3c9ad433-96e5-4790-b170-c29c64a9bbec", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "56b179c5-68ea-4751-9165-d23a582340b0"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "ecd55a05-3e11-40f7-8343-eeecc2c78632"}, { new NonTerminator(NonTerminatorType.Term), "56f61e5b-58a2-485f-aea2-f73fa6da1536"}, { new NonTerminator(NonTerminatorType.Factor), "01061761-6bf0-4be4-b1a0-b74b19d7f1f0"}, { Terminator.NumberTerminator, "7f3d853a-b1d5-4fde-beea-6976c52d955f"}, { new NonTerminator(NonTerminatorType.Variable), "17d6dccc-6754-43c4-a39f-2d5d9ad28cff"}, { new Terminator(DelimiterType.LeftParenthesis), "b57a902f-a0ab-4887-a03b-65da4fb9c521"}, { Terminator.IdentifierTerminator, "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7"}, { new Terminator(KeywordType.Not), "0118ba89-ed50-418b-8262-8e54898e6b67"}, { new Terminator(OperatorType.Minus), "aa631dfb-dbe4-407b-a336-90e730bb4388"},}, new Dictionary<Terminator, ReduceInformation>{ }, "3c9ad433-96e5-4790-b170-c29c64a9bbec") },
|
|
{ "7598cb46-1c5b-4034-8cbd-9cb24dc6e409", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "84d4a81d-badc-46cf-9b28-250c07048f01"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "ecd55a05-3e11-40f7-8343-eeecc2c78632"}, { new NonTerminator(NonTerminatorType.Term), "56f61e5b-58a2-485f-aea2-f73fa6da1536"}, { new NonTerminator(NonTerminatorType.Factor), "01061761-6bf0-4be4-b1a0-b74b19d7f1f0"}, { Terminator.NumberTerminator, "7f3d853a-b1d5-4fde-beea-6976c52d955f"}, { new NonTerminator(NonTerminatorType.Variable), "17d6dccc-6754-43c4-a39f-2d5d9ad28cff"}, { new Terminator(DelimiterType.LeftParenthesis), "b57a902f-a0ab-4887-a03b-65da4fb9c521"}, { Terminator.IdentifierTerminator, "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7"}, { new Terminator(KeywordType.Not), "0118ba89-ed50-418b-8262-8e54898e6b67"}, { new Terminator(OperatorType.Minus), "aa631dfb-dbe4-407b-a336-90e730bb4388"},}, new Dictionary<Terminator, ReduceInformation>{ }, "7598cb46-1c5b-4034-8cbd-9cb24dc6e409") },
|
|
{ "2938411d-d60b-4928-935e-7341545b4c94", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(OperatorType.Assign), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "2938411d-d60b-4928-935e-7341545b4c94") },
|
|
{ "b0d115ad-5000-4eed-b6ed-558743b60e74", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "52d5a53d-3ca2-48e7-855c-c27abbdb2ade"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b0d115ad-5000-4eed-b6ed-558743b60e74") },
|
|
{ "6db3d982-af0e-485b-bb5d-93457809f8ce", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "cbfbe84a-a266-49ab-bbde-0b82c28e4468"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6db3d982-af0e-485b-bb5d-93457809f8ce") },
|
|
{ "04ea29ff-ee71-4f41-bf51-2d170c53cfef", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.Then), "07d41284-f043-4724-8399-05608904a4e5"},}, new Dictionary<Terminator, ReduceInformation>{ }, "04ea29ff-ee71-4f41-bf51-2d170c53cfef") },
|
|
{ "e1d03d71-8bbe-4e20-80bf-217f439b2253", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "1979f79c-b257-4c8e-a369-b53e8c670f1e"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "6dd0c576-4f37-44f5-b566-33df3f8a244f"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "e1d03d71-8bbe-4e20-80bf-217f439b2253") },
|
|
{ "cecc617c-0352-4618-b416-5bad0e13338d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "a368e0dd-a146-4d42-a835-cce8d8fe068a"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "cecc617c-0352-4618-b416-5bad0e13338d") },
|
|
{ "e94ac37d-28f2-4a98-b3c8-1c69a223c4de", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "e94ac37d-28f2-4a98-b3c8-1c69a223c4de") },
|
|
{ "160264c5-eb9b-477e-9697-ddb4f34fc45f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "160264c5-eb9b-477e-9697-ddb4f34fc45f") },
|
|
{ "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f") },
|
|
{ "64e0a597-aae0-4e32-a4aa-59c45f61fe83", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "d3d8a8dc-475f-4f70-9feb-9fee8f4fd8c1"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "64e0a597-aae0-4e32-a4aa-59c45f61fe83") },
|
|
{ "c1b31f89-e41b-4035-a282-0d4bd13e0c82", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "b50119cf-cb5b-44e2-a982-5d3dcc696a93"}, { new NonTerminator(NonTerminatorType.IdVarPart), "5a77c3c0-91e9-4244-b32b-76134a767a34"}, { new Terminator(DelimiterType.LeftSquareBracket), "b73b75d4-902d-4683-8a82-01d9ad550758"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "c1b31f89-e41b-4035-a282-0d4bd13e0c82") },
|
|
{ "000fa394-e859-4466-a821-0c9da688eb4a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "12db7dbe-c1c8-42ac-a506-d1f5850ad1c8"}, { Terminator.NumberTerminator, "160264c5-eb9b-477e-9697-ddb4f34fc45f"}, { new NonTerminator(NonTerminatorType.Variable), "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f"}, { new Terminator(DelimiterType.LeftParenthesis), "64e0a597-aae0-4e32-a4aa-59c45f61fe83"}, { Terminator.IdentifierTerminator, "c1b31f89-e41b-4035-a282-0d4bd13e0c82"}, { new Terminator(KeywordType.Not), "000fa394-e859-4466-a821-0c9da688eb4a"}, { new Terminator(OperatorType.Minus), "c844c091-5a24-42c1-9660-9517d6664fa9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "000fa394-e859-4466-a821-0c9da688eb4a") },
|
|
{ "c844c091-5a24-42c1-9660-9517d6664fa9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "ef1c82b8-fa0f-4e1f-9aa1-2f43f6b14334"}, { Terminator.NumberTerminator, "160264c5-eb9b-477e-9697-ddb4f34fc45f"}, { new NonTerminator(NonTerminatorType.Variable), "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f"}, { new Terminator(DelimiterType.LeftParenthesis), "64e0a597-aae0-4e32-a4aa-59c45f61fe83"}, { Terminator.IdentifierTerminator, "c1b31f89-e41b-4035-a282-0d4bd13e0c82"}, { new Terminator(KeywordType.Not), "000fa394-e859-4466-a821-0c9da688eb4a"}, { new Terminator(OperatorType.Minus), "c844c091-5a24-42c1-9660-9517d6664fa9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c844c091-5a24-42c1-9660-9517d6664fa9") },
|
|
{ "18431573-a0e7-4653-bcec-8616bbdc4e65", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Assign), "a4b4cf4b-21af-45a0-b204-72a6f987a8c8"},}, new Dictionary<Terminator, ReduceInformation>{ }, "18431573-a0e7-4653-bcec-8616bbdc4e65") },
|
|
{ "4a89d600-1e9e-4be7-8913-d461810035a9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.End), "ac12a348-d97b-4cd1-9f45-11a1f6920d0c"}, { new Terminator(DelimiterType.Semicolon), "ca1783b9-fb61-4fbb-886b-0d1de39ddf7d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "4a89d600-1e9e-4be7-8913-d461810035a9") },
|
|
{ "0e1ee01e-4a5e-4e7f-8522-7997f7388c5e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Subprogram))}, }, "0e1ee01e-4a5e-4e7f-8522-7997f7388c5e") },
|
|
{ "6913293e-2acd-46e7-a0cd-af7c67791647", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.VarDeclarations), "bebf75bf-3e28-484e-a003-6c094be8e2b3"}, { new Terminator(KeywordType.Var), "83c77bc6-ce15-4196-a57a-aa8edfbd7e2d"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(0, new NonTerminator(NonTerminatorType.VarDeclarations))}, }, "6913293e-2acd-46e7-a0cd-af7c67791647") },
|
|
{ "e2eed85e-5218-4ed4-a565-6c36024d21b4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ConstDeclaration), "8e58d8fa-ccad-4c5a-85af-6d6b3955cf9c"}, { Terminator.IdentifierTerminator, "350f5711-3321-4fce-ad22-19bd472eae37"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e2eed85e-5218-4ed4-a565-6c36024d21b4") },
|
|
{ "239c34f1-88f7-45aa-b4cc-95e4d83f071e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SubprogramHead))}, }, "239c34f1-88f7-45aa-b4cc-95e4d83f071e") },
|
|
{ "4e57943c-1561-4ee1-a95f-3488069a42a6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ParameterList), "099a005b-f8cd-4efa-8a55-52a47997e3a3"}, { new NonTerminator(NonTerminatorType.Parameter), "841041d3-d12c-4c81-949f-6db2c6bb71de"}, { new NonTerminator(NonTerminatorType.VarParameter), "1a42389c-c349-4c5e-bfa3-d40f719e4a78"}, { new NonTerminator(NonTerminatorType.ValueParameter), "c4477610-5e29-42d3-89fd-ffd5c57feb6a"}, { new Terminator(KeywordType.Var), "2e97b21a-2473-4b7c-9d01-b2493627a1a2"}, { new NonTerminator(NonTerminatorType.IdentifierList), "a0b9084f-7330-4dbd-9874-7c12714a5e00"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ }, "4e57943c-1561-4ee1-a95f-3488069a42a6") },
|
|
{ "fe35e65a-a27e-43a2-b358-dbb2ba56f3b0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Colon), "c4c85c24-1cd9-43c5-999c-380ce7efc37c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "fe35e65a-a27e-43a2-b358-dbb2ba56f3b0") },
|
|
{ "eed34ae6-e7a5-4ba9-bfea-04bcc0acde9c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ParameterList), "2a6466e2-82e5-48e8-8458-585945cd1e39"}, { new NonTerminator(NonTerminatorType.Parameter), "841041d3-d12c-4c81-949f-6db2c6bb71de"}, { new NonTerminator(NonTerminatorType.VarParameter), "1a42389c-c349-4c5e-bfa3-d40f719e4a78"}, { new NonTerminator(NonTerminatorType.ValueParameter), "c4477610-5e29-42d3-89fd-ffd5c57feb6a"}, { new Terminator(KeywordType.Var), "2e97b21a-2473-4b7c-9d01-b2493627a1a2"}, { new NonTerminator(NonTerminatorType.IdentifierList), "a0b9084f-7330-4dbd-9874-7c12714a5e00"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ }, "eed34ae6-e7a5-4ba9-bfea-04bcc0acde9c") },
|
|
{ "8e2917f5-8b23-4cce-b1c4-e1876734f75f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Type), "02f89177-b97f-437b-80c2-cfeee447e89b"}, { new NonTerminator(NonTerminatorType.BasicType), "e587c3e6-05fc-4f92-913a-fb606c8f01a2"}, { new Terminator(KeywordType.Array), "a28b508d-ea6e-47b9-9173-9140b1c7c748"}, { new Terminator(KeywordType.Integer), "d649770f-216c-448b-9cbe-ba827526a2f5"}, { new Terminator(KeywordType.Real), "741f0bed-843f-4135-90f4-4fa0337a7126"}, { new Terminator(KeywordType.Boolean), "981256c3-20fb-44ce-b5ab-9e3a26a98417"}, { new Terminator(KeywordType.Character), "e12136f0-98e7-4894-abb2-8671ea2331c7"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8e2917f5-8b23-4cce-b1c4-e1876734f75f") },
|
|
{ "b16f1ce8-d147-4fad-977b-875f2d3fce4e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Period), "8aa1ebee-9ea4-42ab-a84a-c1335f783be7"}, { Terminator.NumberTerminator, "293757c5-a93b-49d9-b77e-9a380d942ccf"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b16f1ce8-d147-4fad-977b-875f2d3fce4e") },
|
|
{ "2865ba77-6470-42da-9209-f48055d19ed5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(5, new NonTerminator(NonTerminatorType.ConstDeclaration))}, }, "2865ba77-6470-42da-9209-f48055d19ed5") },
|
|
{ "7a1b40a4-b0ab-401a-8499-4b4b4a572c5d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.StatementList))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.StatementList))}, }, "7a1b40a4-b0ab-401a-8499-4b4b4a572c5d") },
|
|
{ "56b179c5-68ea-4751-9165-d23a582340b0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, }, "56b179c5-68ea-4751-9165-d23a582340b0") },
|
|
{ "ecd55a05-3e11-40f7-8343-eeecc2c78632", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "b49a6e75-9814-4f7b-a569-6adbb55841cd"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "253bc019-1da2-40a0-90b3-7e1b21d75bcb"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "ecd55a05-3e11-40f7-8343-eeecc2c78632") },
|
|
{ "56f61e5b-58a2-485f-aea2-f73fa6da1536", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "cbb0931d-780c-46fa-a970-670acfbb3cac"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "56f61e5b-58a2-485f-aea2-f73fa6da1536") },
|
|
{ "01061761-6bf0-4be4-b1a0-b74b19d7f1f0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "01061761-6bf0-4be4-b1a0-b74b19d7f1f0") },
|
|
{ "7f3d853a-b1d5-4fde-beea-6976c52d955f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "7f3d853a-b1d5-4fde-beea-6976c52d955f") },
|
|
{ "17d6dccc-6754-43c4-a39f-2d5d9ad28cff", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "17d6dccc-6754-43c4-a39f-2d5d9ad28cff") },
|
|
{ "b57a902f-a0ab-4887-a03b-65da4fb9c521", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "eb8d30ab-ea2e-4710-bdee-4de1cd0cff0d"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b57a902f-a0ab-4887-a03b-65da4fb9c521") },
|
|
{ "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "73ec5436-9514-46ad-bbaa-de958e90b0de"}, { new NonTerminator(NonTerminatorType.IdVarPart), "ebb42c2b-c4c7-4239-b02a-da10c0caa4f3"}, { new Terminator(DelimiterType.LeftSquareBracket), "15ba3aca-9ac9-4a13-9d47-b183e36b3235"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7") },
|
|
{ "0118ba89-ed50-418b-8262-8e54898e6b67", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "a7e62a3f-9edc-490c-a59a-c41162a40be0"}, { Terminator.NumberTerminator, "7f3d853a-b1d5-4fde-beea-6976c52d955f"}, { new NonTerminator(NonTerminatorType.Variable), "17d6dccc-6754-43c4-a39f-2d5d9ad28cff"}, { new Terminator(DelimiterType.LeftParenthesis), "b57a902f-a0ab-4887-a03b-65da4fb9c521"}, { Terminator.IdentifierTerminator, "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7"}, { new Terminator(KeywordType.Not), "0118ba89-ed50-418b-8262-8e54898e6b67"}, { new Terminator(OperatorType.Minus), "aa631dfb-dbe4-407b-a336-90e730bb4388"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0118ba89-ed50-418b-8262-8e54898e6b67") },
|
|
{ "aa631dfb-dbe4-407b-a336-90e730bb4388", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "27162b06-ccc5-4e5c-bf35-6a0cb1db0fa0"}, { Terminator.NumberTerminator, "7f3d853a-b1d5-4fde-beea-6976c52d955f"}, { new NonTerminator(NonTerminatorType.Variable), "17d6dccc-6754-43c4-a39f-2d5d9ad28cff"}, { new Terminator(DelimiterType.LeftParenthesis), "b57a902f-a0ab-4887-a03b-65da4fb9c521"}, { Terminator.IdentifierTerminator, "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7"}, { new Terminator(KeywordType.Not), "0118ba89-ed50-418b-8262-8e54898e6b67"}, { new Terminator(OperatorType.Minus), "aa631dfb-dbe4-407b-a336-90e730bb4388"},}, new Dictionary<Terminator, ReduceInformation>{ }, "aa631dfb-dbe4-407b-a336-90e730bb4388") },
|
|
{ "84d4a81d-badc-46cf-9b28-250c07048f01", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, }, "84d4a81d-badc-46cf-9b28-250c07048f01") },
|
|
{ "52d5a53d-3ca2-48e7-855c-c27abbdb2ade", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "d9f775bf-aa56-4427-b0b6-91082e2ecaa0"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "52d5a53d-3ca2-48e7-855c-c27abbdb2ade") },
|
|
{ "443eb0a5-40d9-4b8e-b206-363e91ed8aae", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ExpressionList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ExpressionList))}, }, "443eb0a5-40d9-4b8e-b206-363e91ed8aae") },
|
|
{ "af315387-70e8-4940-9a8f-7f894721bee7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "bb71a39f-02cd-4965-9b88-3db31e60bab1"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "1e8fa2c3-6fb9-4370-bfc9-4e8e2cd2d7b4"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "af315387-70e8-4940-9a8f-7f894721bee7") },
|
|
{ "b2337dbe-963f-4559-a9a7-901b2f1496dc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "c254eae9-8d04-4e4a-8df3-458b09013efe"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "b2337dbe-963f-4559-a9a7-901b2f1496dc") },
|
|
{ "972ac3b8-6dc3-4503-8923-1acd997078da", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "972ac3b8-6dc3-4503-8923-1acd997078da") },
|
|
{ "13afc88b-d09a-42c0-be5b-926f3299342e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "13afc88b-d09a-42c0-be5b-926f3299342e") },
|
|
{ "e990e4c7-637f-4348-8ca7-ae6641c98f5f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "e990e4c7-637f-4348-8ca7-ae6641c98f5f") },
|
|
{ "bf0093f2-da22-4e47-9df5-3502975ed106", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "09ab1401-7bea-4b34-880c-388f91d280fd"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "bf0093f2-da22-4e47-9df5-3502975ed106") },
|
|
{ "55349752-e804-4542-b1e6-db73c260479d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "9533615a-a319-40a5-9987-fc2cb3960b06"}, { new NonTerminator(NonTerminatorType.IdVarPart), "b8a32b57-2bc0-4f4f-99bd-f59a1ccd9d11"}, { new Terminator(DelimiterType.LeftSquareBracket), "f833834b-f732-4d24-852f-6b8b3ad48211"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "55349752-e804-4542-b1e6-db73c260479d") },
|
|
{ "f01f63f7-64d9-4726-8917-634575d1bd27", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "6d46a46b-3965-44f4-b163-7c2e6b70e84e"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f01f63f7-64d9-4726-8917-634575d1bd27") },
|
|
{ "d0b41fc4-34c3-4b9e-a939-477279006d43", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "ca432529-3a30-456c-829b-2995ab5e6649"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d0b41fc4-34c3-4b9e-a939-477279006d43") },
|
|
{ "cbfbe84a-a266-49ab-bbde-0b82c28e4468", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "49868f67-0d68-475b-9a01-29105a951d90"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "cbfbe84a-a266-49ab-bbde-0b82c28e4468") },
|
|
{ "09556f9b-3c83-477b-8464-0cab6d8e1bee", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ExpressionList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ExpressionList))}, }, "09556f9b-3c83-477b-8464-0cab6d8e1bee") },
|
|
{ "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "8f009ccb-5fe1-4ea5-aaae-62b0e7e9ff1f"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "8eceb0e2-2e1f-499b-858b-15be318f4e9a"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba") },
|
|
{ "f2a35504-1720-4849-9ae3-8ec0c5b49854", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "5e0fd40a-6707-48b6-8187-e404dc744a7b"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "f2a35504-1720-4849-9ae3-8ec0c5b49854") },
|
|
{ "d2d61ae7-84e4-4609-96e1-336bc474312f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "d2d61ae7-84e4-4609-96e1-336bc474312f") },
|
|
{ "aa92ed9e-dde5-4295-84e5-f047a7118d25", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "aa92ed9e-dde5-4295-84e5-f047a7118d25") },
|
|
{ "372bc844-539e-4c2f-b103-bedf27599ff9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "372bc844-539e-4c2f-b103-bedf27599ff9") },
|
|
{ "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "72115296-b2d4-4cf5-b4db-b5863bc51468"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b") },
|
|
{ "2fda7058-df05-491d-a157-6b562f943743", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "57651180-5cc5-4de8-b41f-adad15688420"}, { new NonTerminator(NonTerminatorType.IdVarPart), "93b02c93-034b-4d27-a5d7-9c7847cfcb75"}, { new Terminator(DelimiterType.LeftSquareBracket), "52d2a6d1-d832-4518-afa6-0e9177726cd3"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "2fda7058-df05-491d-a157-6b562f943743") },
|
|
{ "cfda5c15-06e0-47a7-a1db-8a5062493970", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "8e0afe7c-4e12-433b-a19d-58c5406ea92c"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "cfda5c15-06e0-47a7-a1db-8a5062493970") },
|
|
{ "b39e46cf-fb12-40fa-84eb-da91399dd049", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "c80a7a16-36e9-4d3f-ad90-1e929dd3c8ab"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b39e46cf-fb12-40fa-84eb-da91399dd049") },
|
|
{ "07d41284-f043-4724-8399-05608904a4e5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Statement), "b82136ea-c714-4539-8f07-a9cf837cd734"}, { new NonTerminator(NonTerminatorType.Variable), "95ec03de-0feb-40ef-8d99-6e17f1af43bc"}, { Terminator.IdentifierTerminator, "d5afeb9a-86c4-4038-b0cc-cddf89462d0d"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "c99a68a9-af82-472a-b1a8-40f6398859c5"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "d2c7c905-6a01-48f9-a191-8b6e18062cce"}, { new Terminator(KeywordType.If), "197d696b-bb93-47c1-a2d6-32f6f58a2966"}, { new Terminator(KeywordType.For), "954dbde8-c20c-421b-b9af-3f71898d6f01"}, { new Terminator(KeywordType.Begin), "df0cac11-1ad1-4f31-87ee-0350ca6051f4"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "07d41284-f043-4724-8399-05608904a4e5") },
|
|
{ "1979f79c-b257-4c8e-a369-b53e8c670f1e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "176d52b9-5765-4f4f-976b-ad6772b9ff8f"}, { new NonTerminator(NonTerminatorType.Term), "70fc898f-6a01-438c-a311-dff3d2c8b22a"}, { new NonTerminator(NonTerminatorType.Factor), "86726642-31b6-468b-97d8-aa0bd78b5290"}, { Terminator.NumberTerminator, "b5045e69-5b08-443a-a4dd-bcfc531ec151"}, { new NonTerminator(NonTerminatorType.Variable), "e667abdf-5bac-4bd4-ba6d-30a41ebda6b1"}, { new Terminator(DelimiterType.LeftParenthesis), "fac69cb2-c9e2-489c-a5f6-aeacd11a1701"}, { Terminator.IdentifierTerminator, "6980c787-4656-4f35-adcd-321bfabbbd05"}, { new Terminator(KeywordType.Not), "75de4e67-0e89-435b-8b07-17bc55e72853"}, { new Terminator(OperatorType.Minus), "1c9d4136-0793-411a-9895-8f3ce17193a3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1979f79c-b257-4c8e-a369-b53e8c670f1e") },
|
|
{ "79a105dd-26a8-48e1-a1e5-574c75949b4d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, }, "79a105dd-26a8-48e1-a1e5-574c75949b4d") },
|
|
{ "6afacd0d-b338-4375-8311-1af9477b2050", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, }, "6afacd0d-b338-4375-8311-1af9477b2050") },
|
|
{ "b19156b3-e778-413d-b25a-ae00fc21ab09", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, }, "b19156b3-e778-413d-b25a-ae00fc21ab09") },
|
|
{ "583c85b9-f1c6-454a-9d8a-9954789412ec", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, }, "583c85b9-f1c6-454a-9d8a-9954789412ec") },
|
|
{ "74e622b4-8fc3-49a2-992b-87c0d048f09a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, }, "74e622b4-8fc3-49a2-992b-87c0d048f09a") },
|
|
{ "5db54d7e-231b-43b7-be0f-bb3021e1915a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.RelationOperator))}, }, "5db54d7e-231b-43b7-be0f-bb3021e1915a") },
|
|
{ "6dd0c576-4f37-44f5-b566-33df3f8a244f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "237238a0-37d2-40b1-b85b-481da02c50b9"}, { new NonTerminator(NonTerminatorType.Factor), "e94ac37d-28f2-4a98-b3c8-1c69a223c4de"}, { Terminator.NumberTerminator, "160264c5-eb9b-477e-9697-ddb4f34fc45f"}, { new NonTerminator(NonTerminatorType.Variable), "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f"}, { new Terminator(DelimiterType.LeftParenthesis), "64e0a597-aae0-4e32-a4aa-59c45f61fe83"}, { Terminator.IdentifierTerminator, "c1b31f89-e41b-4035-a282-0d4bd13e0c82"}, { new Terminator(KeywordType.Not), "000fa394-e859-4466-a821-0c9da688eb4a"}, { new Terminator(OperatorType.Minus), "c844c091-5a24-42c1-9660-9517d6664fa9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6dd0c576-4f37-44f5-b566-33df3f8a244f") },
|
|
{ "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, }, "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981") },
|
|
{ "521c4a92-4030-40b7-a6af-9b8ec1f4358f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, }, "521c4a92-4030-40b7-a6af-9b8ec1f4358f") },
|
|
{ "273a6a36-3c91-4795-b9b6-8884d837b5a8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.AddOperator))}, }, "273a6a36-3c91-4795-b9b6-8884d837b5a8") },
|
|
{ "a368e0dd-a146-4d42-a835-cce8d8fe068a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "ad08eb42-95d9-40c5-80d2-c91eee28ac62"}, { Terminator.NumberTerminator, "160264c5-eb9b-477e-9697-ddb4f34fc45f"}, { new NonTerminator(NonTerminatorType.Variable), "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f"}, { new Terminator(DelimiterType.LeftParenthesis), "64e0a597-aae0-4e32-a4aa-59c45f61fe83"}, { Terminator.IdentifierTerminator, "c1b31f89-e41b-4035-a282-0d4bd13e0c82"}, { new Terminator(KeywordType.Not), "000fa394-e859-4466-a821-0c9da688eb4a"}, { new Terminator(OperatorType.Minus), "c844c091-5a24-42c1-9660-9517d6664fa9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a368e0dd-a146-4d42-a835-cce8d8fe068a") },
|
|
{ "7896e50c-802f-4079-bc51-521da71c5940", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, }, "7896e50c-802f-4079-bc51-521da71c5940") },
|
|
{ "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, }, "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4") },
|
|
{ "8c25ace4-2cdf-4493-8fc3-24c8110c9e53", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, }, "8c25ace4-2cdf-4493-8fc3-24c8110c9e53") },
|
|
{ "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, }, "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28") },
|
|
{ "19e2b148-c63e-4b68-9299-549db9054923", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { Terminator.NumberTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { Terminator.IdentifierTerminator, new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(DelimiterType.LeftParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(KeywordType.Not), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.MultiplyOperator))}, }, "19e2b148-c63e-4b68-9299-549db9054923") },
|
|
{ "d3d8a8dc-475f-4f70-9feb-9fee8f4fd8c1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "2d3427fb-2c1f-411a-902b-fff3c4774ebe"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d3d8a8dc-475f-4f70-9feb-9fee8f4fd8c1") },
|
|
{ "0f6db587-6cd7-4ecc-8651-c6d50006f10a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "5417883a-4e03-4e45-a1c9-897b98a50c9c"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "623f1f11-53c3-4917-8373-d9448dadbf72"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "0f6db587-6cd7-4ecc-8651-c6d50006f10a") },
|
|
{ "29ba3a00-e922-4557-afb9-6f5d3609d6ee", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "1efdc93c-1cf5-42ba-b76e-4913b093fe1e"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "29ba3a00-e922-4557-afb9-6f5d3609d6ee") },
|
|
{ "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2") },
|
|
{ "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749") },
|
|
{ "27067d65-a3e8-4670-b353-2f4a7abd2ab7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "27067d65-a3e8-4670-b353-2f4a7abd2ab7") },
|
|
{ "cb7a9eca-2c00-4aa1-b40d-d9c025922749", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "bbc42cc9-4082-4219-9bd8-62c653270c33"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "cb7a9eca-2c00-4aa1-b40d-d9c025922749") },
|
|
{ "5022f026-8866-40ab-8bd7-91eade276b7d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "3aacd451-1def-4cf1-be9a-977567f01d08"}, { new NonTerminator(NonTerminatorType.IdVarPart), "bef05338-3156-4599-9a62-f4491d7c83be"}, { new Terminator(DelimiterType.LeftSquareBracket), "2c8d4e5a-c721-473a-a25b-a9457d94715c"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "5022f026-8866-40ab-8bd7-91eade276b7d") },
|
|
{ "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "07375a4c-6fb3-4a10-9446-56ade98b9f97"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3") },
|
|
{ "8c33c722-e4b7-43e5-b416-eac9ae0200b3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "d43d3c4f-71e8-4d92-b606-d7448fbe4fac"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8c33c722-e4b7-43e5-b416-eac9ae0200b3") },
|
|
{ "b50119cf-cb5b-44e2-a982-5d3dcc696a93", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "a35c1189-edfa-4093-bf69-3124685c5f26"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b50119cf-cb5b-44e2-a982-5d3dcc696a93") },
|
|
{ "5a77c3c0-91e9-4244-b32b-76134a767a34", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "5a77c3c0-91e9-4244-b32b-76134a767a34") },
|
|
{ "b73b75d4-902d-4683-8a82-01d9ad550758", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "ad37ddff-25f5-4011-bf88-5ac4bd0cb2f1"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b73b75d4-902d-4683-8a82-01d9ad550758") },
|
|
{ "12db7dbe-c1c8-42ac-a506-d1f5850ad1c8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "12db7dbe-c1c8-42ac-a506-d1f5850ad1c8") },
|
|
{ "ef1c82b8-fa0f-4e1f-9aa1-2f43f6b14334", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "ef1c82b8-fa0f-4e1f-9aa1-2f43f6b14334") },
|
|
{ "a4b4cf4b-21af-45a0-b204-72a6f987a8c8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "5de3330d-a6c6-4099-8af9-cfb659d47b00"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bbdbc5e4-202b-4d40-a862-c9092cfb6c67"}, { new NonTerminator(NonTerminatorType.Term), "fe68835b-da88-4eda-940c-19f73c430df7"}, { new NonTerminator(NonTerminatorType.Factor), "3b214e0e-230c-481b-8c15-f304cc856f86"}, { Terminator.NumberTerminator, "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4"}, { new NonTerminator(NonTerminatorType.Variable), "f56343e4-f729-4764-b769-0e133ee3a2f2"}, { new Terminator(DelimiterType.LeftParenthesis), "cdf73a03-7454-4da9-942e-e01adf2d6592"}, { Terminator.IdentifierTerminator, "38247e93-f150-453a-b02a-809c7b22ef1d"}, { new Terminator(KeywordType.Not), "16b14840-8c2b-4856-a232-ebcc29c0f7a5"}, { new Terminator(OperatorType.Minus), "f8243a3e-9016-47fc-88a3-453d416dc854"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a4b4cf4b-21af-45a0-b204-72a6f987a8c8") },
|
|
{ "ac12a348-d97b-4cd1-9f45-11a1f6920d0c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.CompoundStatement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.CompoundStatement))}, }, "ac12a348-d97b-4cd1-9f45-11a1f6920d0c") },
|
|
{ "bebf75bf-3e28-484e-a003-6c094be8e2b3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.CompoundStatement), "b33610ed-b390-486f-923a-714bf7147197"}, { new Terminator(KeywordType.Begin), "91fa9734-aa7f-4b34-a139-cfa85c396cbc"},}, new Dictionary<Terminator, ReduceInformation>{ }, "bebf75bf-3e28-484e-a003-6c094be8e2b3") },
|
|
{ "83c77bc6-ce15-4196-a57a-aa8edfbd7e2d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.VarDeclaration), "c10cd1be-21ef-4e0d-9722-d6c895dfbf79"}, { new NonTerminator(NonTerminatorType.IdentifierList), "153fdd10-03fc-4d0b-8b71-de6c4a119383"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ }, "83c77bc6-ce15-4196-a57a-aa8edfbd7e2d") },
|
|
{ "8e58d8fa-ccad-4c5a-85af-6d6b3955cf9c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Semicolon), "520f9af3-ed17-4e0e-aa24-a461c3d448a5"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8e58d8fa-ccad-4c5a-85af-6d6b3955cf9c") },
|
|
{ "099a005b-f8cd-4efa-8a55-52a47997e3a3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "a50aa3cb-351c-4216-a2ee-5fa1496ba01f"}, { new Terminator(DelimiterType.Semicolon), "24c2af8e-f211-40ca-b06e-af64dabc2315"},}, new Dictionary<Terminator, ReduceInformation>{ }, "099a005b-f8cd-4efa-8a55-52a47997e3a3") },
|
|
{ "841041d3-d12c-4c81-949f-6db2c6bb71de", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ParameterList))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ParameterList))}, }, "841041d3-d12c-4c81-949f-6db2c6bb71de") },
|
|
{ "1a42389c-c349-4c5e-bfa3-d40f719e4a78", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Parameter))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Parameter))}, }, "1a42389c-c349-4c5e-bfa3-d40f719e4a78") },
|
|
{ "c4477610-5e29-42d3-89fd-ffd5c57feb6a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Parameter))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Parameter))}, }, "c4477610-5e29-42d3-89fd-ffd5c57feb6a") },
|
|
{ "2e97b21a-2473-4b7c-9d01-b2493627a1a2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ValueParameter), "fa52306a-d38a-47a5-8a7f-23e2895334f5"}, { new NonTerminator(NonTerminatorType.IdentifierList), "a0b9084f-7330-4dbd-9874-7c12714a5e00"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ }, "2e97b21a-2473-4b7c-9d01-b2493627a1a2") },
|
|
{ "a0b9084f-7330-4dbd-9874-7c12714a5e00", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Colon), "f10e618d-59ed-4a23-b6dc-02f2d0f6dd3e"}, { new Terminator(DelimiterType.Comma), "f780d1df-5e94-4b1b-ba7d-0107db38c3b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a0b9084f-7330-4dbd-9874-7c12714a5e00") },
|
|
{ "c4c85c24-1cd9-43c5-999c-380ce7efc37c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.BasicType), "1c605559-8a9b-45b5-a03c-29c01028439c"}, { new Terminator(KeywordType.Integer), "d649770f-216c-448b-9cbe-ba827526a2f5"}, { new Terminator(KeywordType.Real), "741f0bed-843f-4135-90f4-4fa0337a7126"}, { new Terminator(KeywordType.Boolean), "981256c3-20fb-44ce-b5ab-9e3a26a98417"}, { new Terminator(KeywordType.Character), "e12136f0-98e7-4894-abb2-8671ea2331c7"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c4c85c24-1cd9-43c5-999c-380ce7efc37c") },
|
|
{ "2a6466e2-82e5-48e8-8458-585945cd1e39", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "4a95c5d4-3386-4dd6-84eb-c010dd493b55"}, { new Terminator(DelimiterType.Semicolon), "24c2af8e-f211-40ca-b06e-af64dabc2315"},}, new Dictionary<Terminator, ReduceInformation>{ }, "2a6466e2-82e5-48e8-8458-585945cd1e39") },
|
|
{ "02f89177-b97f-437b-80c2-cfeee447e89b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(5, new NonTerminator(NonTerminatorType.VarDeclaration))}, }, "02f89177-b97f-437b-80c2-cfeee447e89b") },
|
|
{ "8aa1ebee-9ea4-42ab-a84a-c1335f783be7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "b2c7444b-c362-4975-8612-431738df31e6"}, { new Terminator(DelimiterType.Comma), "36b4ab41-2d5d-40cb-9add-0a4ccd140262"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8aa1ebee-9ea4-42ab-a84a-c1335f783be7") },
|
|
{ "293757c5-a93b-49d9-b77e-9a380d942ccf", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.DoubleDots), "313f579d-7a53-4d48-bbcc-41609243ae15"},}, new Dictionary<Terminator, ReduceInformation>{ }, "293757c5-a93b-49d9-b77e-9a380d942ccf") },
|
|
{ "b49a6e75-9814-4f7b-a569-6adbb55841cd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "ccf04ef5-d768-4039-9177-7c358b075e35"}, { new NonTerminator(NonTerminatorType.Term), "709c6303-5083-4422-a7e5-cb3066d1aefd"}, { new NonTerminator(NonTerminatorType.Factor), "840498d9-7584-4b94-9182-f56adfb0a56d"}, { Terminator.NumberTerminator, "a94e0a8a-3c31-4b45-b757-8a1e6b19e511"}, { new NonTerminator(NonTerminatorType.Variable), "ca7cefdc-b17f-4e84-8b42-829590b1110e"}, { new Terminator(DelimiterType.LeftParenthesis), "9019af5d-8a33-44d4-954c-d8f218b3ec5e"}, { Terminator.IdentifierTerminator, "f0064b59-541c-4e17-b719-937423bb36c5"}, { new Terminator(KeywordType.Not), "6196e383-c5cd-4279-9cbb-85bd6bdc1de4"}, { new Terminator(OperatorType.Minus), "323aa85f-eb14-4398-a5a6-e62e621aa1c2"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b49a6e75-9814-4f7b-a569-6adbb55841cd") },
|
|
{ "253bc019-1da2-40a0-90b3-7e1b21d75bcb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "c4307f56-7e81-4055-8987-ed9826ee70e3"}, { new NonTerminator(NonTerminatorType.Factor), "01061761-6bf0-4be4-b1a0-b74b19d7f1f0"}, { Terminator.NumberTerminator, "7f3d853a-b1d5-4fde-beea-6976c52d955f"}, { new NonTerminator(NonTerminatorType.Variable), "17d6dccc-6754-43c4-a39f-2d5d9ad28cff"}, { new Terminator(DelimiterType.LeftParenthesis), "b57a902f-a0ab-4887-a03b-65da4fb9c521"}, { Terminator.IdentifierTerminator, "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7"}, { new Terminator(KeywordType.Not), "0118ba89-ed50-418b-8262-8e54898e6b67"}, { new Terminator(OperatorType.Minus), "aa631dfb-dbe4-407b-a336-90e730bb4388"},}, new Dictionary<Terminator, ReduceInformation>{ }, "253bc019-1da2-40a0-90b3-7e1b21d75bcb") },
|
|
{ "cbb0931d-780c-46fa-a970-670acfbb3cac", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "3a86cef5-67a4-4910-ae34-6ddd3e209ac6"}, { Terminator.NumberTerminator, "7f3d853a-b1d5-4fde-beea-6976c52d955f"}, { new NonTerminator(NonTerminatorType.Variable), "17d6dccc-6754-43c4-a39f-2d5d9ad28cff"}, { new Terminator(DelimiterType.LeftParenthesis), "b57a902f-a0ab-4887-a03b-65da4fb9c521"}, { Terminator.IdentifierTerminator, "0d46bc0d-9b3c-43f2-90f8-bc3f1f74dbf7"}, { new Terminator(KeywordType.Not), "0118ba89-ed50-418b-8262-8e54898e6b67"}, { new Terminator(OperatorType.Minus), "aa631dfb-dbe4-407b-a336-90e730bb4388"},}, new Dictionary<Terminator, ReduceInformation>{ }, "cbb0931d-780c-46fa-a970-670acfbb3cac") },
|
|
{ "eb8d30ab-ea2e-4710-bdee-4de1cd0cff0d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "6492230d-2e12-4609-bc2a-cbc4f91c8820"},}, new Dictionary<Terminator, ReduceInformation>{ }, "eb8d30ab-ea2e-4710-bdee-4de1cd0cff0d") },
|
|
{ "73ec5436-9514-46ad-bbaa-de958e90b0de", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "37939423-f4db-4ab7-9e15-d8e30c811a8d"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "73ec5436-9514-46ad-bbaa-de958e90b0de") },
|
|
{ "ebb42c2b-c4c7-4239-b02a-da10c0caa4f3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "ebb42c2b-c4c7-4239-b02a-da10c0caa4f3") },
|
|
{ "15ba3aca-9ac9-4a13-9d47-b183e36b3235", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "d04a50f5-c163-4c1e-b9db-cefaf42fd995"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "15ba3aca-9ac9-4a13-9d47-b183e36b3235") },
|
|
{ "a7e62a3f-9edc-490c-a59a-c41162a40be0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "a7e62a3f-9edc-490c-a59a-c41162a40be0") },
|
|
{ "27162b06-ccc5-4e5c-bf35-6a0cb1db0fa0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "27162b06-ccc5-4e5c-bf35-6a0cb1db0fa0") },
|
|
{ "d9f775bf-aa56-4427-b0b6-91082e2ecaa0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(OperatorType.Assign), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "d9f775bf-aa56-4427-b0b6-91082e2ecaa0") },
|
|
{ "b9ff9839-3a26-4a8c-9679-3f5636b81d03", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "ec00a7c3-8437-4547-a444-1d97e6260c39"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b9ff9839-3a26-4a8c-9679-3f5636b81d03") },
|
|
{ "bb71a39f-02cd-4965-9b88-3db31e60bab1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "12f85add-4cb2-4579-9dec-602ba96c0474"}, { new NonTerminator(NonTerminatorType.Term), "ca87ba4b-609f-4b45-9779-7a40f73c239b"}, { new NonTerminator(NonTerminatorType.Factor), "51f72fb0-d985-4bc6-b47a-9ea0753d7907"}, { Terminator.NumberTerminator, "13ca9c1b-760f-4da0-878b-72b23c8a6a55"}, { new NonTerminator(NonTerminatorType.Variable), "35529643-d0ce-4527-ad73-841e6868903b"}, { new Terminator(DelimiterType.LeftParenthesis), "9b07659a-c168-4c5b-8781-b6c11ea204a0"}, { Terminator.IdentifierTerminator, "df0def68-2aa5-49b6-8869-9e2d30b4c48d"}, { new Terminator(KeywordType.Not), "fec38012-07b5-4ba8-ae88-a68a714f1cbc"}, { new Terminator(OperatorType.Minus), "727581eb-84d2-411d-9872-e367a0ccc78c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "bb71a39f-02cd-4965-9b88-3db31e60bab1") },
|
|
{ "1e8fa2c3-6fb9-4370-bfc9-4e8e2cd2d7b4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "e5f440de-e88c-4b32-8fd0-9e244b30d5fc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1e8fa2c3-6fb9-4370-bfc9-4e8e2cd2d7b4") },
|
|
{ "c254eae9-8d04-4e4a-8df3-458b09013efe", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "7bcbb8fd-e886-4575-8412-00b4a9da4191"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c254eae9-8d04-4e4a-8df3-458b09013efe") },
|
|
{ "09ab1401-7bea-4b34-880c-388f91d280fd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "4cb65c7a-7ebe-4ee8-aa87-bddbd75caeb9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "09ab1401-7bea-4b34-880c-388f91d280fd") },
|
|
{ "9533615a-a319-40a5-9987-fc2cb3960b06", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "5ae12e51-d94e-425b-b223-5a12afaeda8b"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "9533615a-a319-40a5-9987-fc2cb3960b06") },
|
|
{ "b8a32b57-2bc0-4f4f-99bd-f59a1ccd9d11", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "b8a32b57-2bc0-4f4f-99bd-f59a1ccd9d11") },
|
|
{ "f833834b-f732-4d24-852f-6b8b3ad48211", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "03b1c5aa-6683-4422-9316-0f3f3d213c54"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f833834b-f732-4d24-852f-6b8b3ad48211") },
|
|
{ "6d46a46b-3965-44f4-b163-7c2e6b70e84e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "6d46a46b-3965-44f4-b163-7c2e6b70e84e") },
|
|
{ "ca432529-3a30-456c-829b-2995ab5e6649", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "ca432529-3a30-456c-829b-2995ab5e6649") },
|
|
{ "49868f67-0d68-475b-9a01-29105a951d90", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(4, new NonTerminator(NonTerminatorType.ProcedureCall))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(4, new NonTerminator(NonTerminatorType.ProcedureCall))}, }, "49868f67-0d68-475b-9a01-29105a951d90") },
|
|
{ "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "7e357ece-1708-4c9d-8a54-84b4256bcfdd"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef") },
|
|
{ "8f009ccb-5fe1-4ea5-aaae-62b0e7e9ff1f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "61d911f0-a8fc-4bc9-87d9-b58ce3f9f5cb"}, { new NonTerminator(NonTerminatorType.Term), "6c17a0f8-afa7-4e7a-8934-0216c55df6c7"}, { new NonTerminator(NonTerminatorType.Factor), "f0d0fcd0-1d8a-405f-9354-a994b496f73d"}, { Terminator.NumberTerminator, "3ffc269a-694c-4697-9e31-73f752ab9d1f"}, { new NonTerminator(NonTerminatorType.Variable), "188a892f-e2b1-465e-85e1-67451cbfe493"}, { new Terminator(DelimiterType.LeftParenthesis), "29f01b0d-b7fb-46f9-9522-5c1dbec0b06c"}, { Terminator.IdentifierTerminator, "8306e662-fca1-48d9-bedf-bd4bc289518c"}, { new Terminator(KeywordType.Not), "393677af-7726-490c-9f16-7587445de9f6"}, { new Terminator(OperatorType.Minus), "653e16fa-8ba0-4f39-8e14-063ba0fcc359"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8f009ccb-5fe1-4ea5-aaae-62b0e7e9ff1f") },
|
|
{ "8eceb0e2-2e1f-499b-858b-15be318f4e9a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "0f34877d-5028-4e35-bae5-f71fab556329"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8eceb0e2-2e1f-499b-858b-15be318f4e9a") },
|
|
{ "5e0fd40a-6707-48b6-8187-e404dc744a7b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "e04d7719-7961-41ef-877f-76c3f1e9c07d"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5e0fd40a-6707-48b6-8187-e404dc744a7b") },
|
|
{ "72115296-b2d4-4cf5-b4db-b5863bc51468", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "ee04eb34-e49b-4496-80b9-837ace95dfb7"},}, new Dictionary<Terminator, ReduceInformation>{ }, "72115296-b2d4-4cf5-b4db-b5863bc51468") },
|
|
{ "57651180-5cc5-4de8-b41f-adad15688420", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "e00991ec-e31d-459e-924f-3dd992323cd8"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "57651180-5cc5-4de8-b41f-adad15688420") },
|
|
{ "93b02c93-034b-4d27-a5d7-9c7847cfcb75", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "93b02c93-034b-4d27-a5d7-9c7847cfcb75") },
|
|
{ "52d2a6d1-d832-4518-afa6-0e9177726cd3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "c7f27ef7-8244-4eb9-8d74-75b0a048adcb"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "52d2a6d1-d832-4518-afa6-0e9177726cd3") },
|
|
{ "8e0afe7c-4e12-433b-a19d-58c5406ea92c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "8e0afe7c-4e12-433b-a19d-58c5406ea92c") },
|
|
{ "c80a7a16-36e9-4d3f-ad90-1e929dd3c8ab", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "c80a7a16-36e9-4d3f-ad90-1e929dd3c8ab") },
|
|
{ "b82136ea-c714-4539-8f07-a9cf837cd734", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ElsePart), "99ec1fc2-97e4-4a1e-9624-2c7a38b38093"}, { new Terminator(KeywordType.Else), "7d267d53-3ce0-4d70-9b6c-0621875ae971"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ElsePart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ElsePart))}, }, "b82136ea-c714-4539-8f07-a9cf837cd734") },
|
|
{ "95ec03de-0feb-40ef-8d99-6e17f1af43bc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Assign), "e7cca867-263f-4ac7-a7a8-33bff1f48284"},}, new Dictionary<Terminator, ReduceInformation>{ }, "95ec03de-0feb-40ef-8d99-6e17f1af43bc") },
|
|
{ "d5afeb9a-86c4-4038-b0cc-cddf89462d0d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Assign), "cd8c7020-b7cb-4d2c-89e2-43a1e972dfb9"}, { new NonTerminator(NonTerminatorType.IdVarPart), "2938411d-d60b-4928-935e-7341545b4c94"}, { new Terminator(DelimiterType.LeftSquareBracket), "b0d115ad-5000-4eed-b6ed-558743b60e74"}, { new Terminator(DelimiterType.LeftParenthesis), "8cba8140-28f5-4468-a66e-3e1c1907d8e6"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(OperatorType.Assign), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ProcedureCall))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ProcedureCall))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.ProcedureCall))}, }, "d5afeb9a-86c4-4038-b0cc-cddf89462d0d") },
|
|
{ "c99a68a9-af82-472a-b1a8-40f6398859c5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, }, "c99a68a9-af82-472a-b1a8-40f6398859c5") },
|
|
{ "d2c7c905-6a01-48f9-a191-8b6e18062cce", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Statement))}, }, "d2c7c905-6a01-48f9-a191-8b6e18062cce") },
|
|
{ "197d696b-bb93-47c1-a2d6-32f6f58a2966", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "e4f1500c-cfbc-48c9-bcff-0c33500e01c7"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "e1d03d71-8bbe-4e20-80bf-217f439b2253"}, { new NonTerminator(NonTerminatorType.Term), "cecc617c-0352-4618-b416-5bad0e13338d"}, { new NonTerminator(NonTerminatorType.Factor), "e94ac37d-28f2-4a98-b3c8-1c69a223c4de"}, { Terminator.NumberTerminator, "160264c5-eb9b-477e-9697-ddb4f34fc45f"}, { new NonTerminator(NonTerminatorType.Variable), "aa8f146c-ae0f-49b6-968d-2e0f2c5f641f"}, { new Terminator(DelimiterType.LeftParenthesis), "64e0a597-aae0-4e32-a4aa-59c45f61fe83"}, { Terminator.IdentifierTerminator, "c1b31f89-e41b-4035-a282-0d4bd13e0c82"}, { new Terminator(KeywordType.Not), "000fa394-e859-4466-a821-0c9da688eb4a"}, { new Terminator(OperatorType.Minus), "c844c091-5a24-42c1-9660-9517d6664fa9"},}, new Dictionary<Terminator, ReduceInformation>{ }, "197d696b-bb93-47c1-a2d6-32f6f58a2966") },
|
|
{ "954dbde8-c20c-421b-b9af-3f71898d6f01", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "664bbdbe-f6d7-465e-9391-dbcd925093e8"},}, new Dictionary<Terminator, ReduceInformation>{ }, "954dbde8-c20c-421b-b9af-3f71898d6f01") },
|
|
{ "df0cac11-1ad1-4f31-87ee-0350ca6051f4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.StatementList), "88d16383-f68f-4824-87d9-bd2134c94983"}, { new NonTerminator(NonTerminatorType.Statement), "2dac9c20-107b-46e4-a18b-fdf58e625107"}, { new NonTerminator(NonTerminatorType.Variable), "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1"}, { Terminator.IdentifierTerminator, "2103d893-c079-4b22-81d8-4f9f358fe6ad"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "e53241b0-bb50-48d3-845a-063d9ecbbdeb"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a"}, { new Terminator(KeywordType.If), "c9145487-1b31-498c-9722-1f68bba31eab"}, { new Terminator(KeywordType.For), "d93a638a-474b-4cbd-93db-107fb792750f"}, { new Terminator(KeywordType.Begin), "bbe1d91e-d0c2-4be2-8184-4548587afa0e"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "df0cac11-1ad1-4f31-87ee-0350ca6051f4") },
|
|
{ "176d52b9-5765-4f4f-976b-ad6772b9ff8f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "f2998caf-7e35-441c-ade5-0e046846bae4"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "176d52b9-5765-4f4f-976b-ad6772b9ff8f") },
|
|
{ "70fc898f-6a01-438c-a311-dff3d2c8b22a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "2c40c3e6-9e5d-4a04-9a03-d54fa03e9fd6"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "70fc898f-6a01-438c-a311-dff3d2c8b22a") },
|
|
{ "86726642-31b6-468b-97d8-aa0bd78b5290", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "86726642-31b6-468b-97d8-aa0bd78b5290") },
|
|
{ "b5045e69-5b08-443a-a4dd-bcfc531ec151", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "b5045e69-5b08-443a-a4dd-bcfc531ec151") },
|
|
{ "e667abdf-5bac-4bd4-ba6d-30a41ebda6b1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "e667abdf-5bac-4bd4-ba6d-30a41ebda6b1") },
|
|
{ "fac69cb2-c9e2-489c-a5f6-aeacd11a1701", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "6a6374aa-3719-4edb-b619-44b5b94f59f0"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "fac69cb2-c9e2-489c-a5f6-aeacd11a1701") },
|
|
{ "6980c787-4656-4f35-adcd-321bfabbbd05", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "5cd1041b-f882-46c0-90fb-99adcd19f617"}, { new NonTerminator(NonTerminatorType.IdVarPart), "3e572cc3-8db3-4bd0-83a1-c5006f6e8c02"}, { new Terminator(DelimiterType.LeftSquareBracket), "8bedebe5-33b9-43e8-b0b5-cad41bcccd6f"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "6980c787-4656-4f35-adcd-321bfabbbd05") },
|
|
{ "75de4e67-0e89-435b-8b07-17bc55e72853", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "0cd606f3-0bf2-45f7-9ebf-da3722a4a606"}, { Terminator.NumberTerminator, "b5045e69-5b08-443a-a4dd-bcfc531ec151"}, { new NonTerminator(NonTerminatorType.Variable), "e667abdf-5bac-4bd4-ba6d-30a41ebda6b1"}, { new Terminator(DelimiterType.LeftParenthesis), "fac69cb2-c9e2-489c-a5f6-aeacd11a1701"}, { Terminator.IdentifierTerminator, "6980c787-4656-4f35-adcd-321bfabbbd05"}, { new Terminator(KeywordType.Not), "75de4e67-0e89-435b-8b07-17bc55e72853"}, { new Terminator(OperatorType.Minus), "1c9d4136-0793-411a-9895-8f3ce17193a3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "75de4e67-0e89-435b-8b07-17bc55e72853") },
|
|
{ "1c9d4136-0793-411a-9895-8f3ce17193a3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "e303b300-36f4-4cd7-ab84-5f25c89bef58"}, { Terminator.NumberTerminator, "b5045e69-5b08-443a-a4dd-bcfc531ec151"}, { new NonTerminator(NonTerminatorType.Variable), "e667abdf-5bac-4bd4-ba6d-30a41ebda6b1"}, { new Terminator(DelimiterType.LeftParenthesis), "fac69cb2-c9e2-489c-a5f6-aeacd11a1701"}, { Terminator.IdentifierTerminator, "6980c787-4656-4f35-adcd-321bfabbbd05"}, { new Terminator(KeywordType.Not), "75de4e67-0e89-435b-8b07-17bc55e72853"}, { new Terminator(OperatorType.Minus), "1c9d4136-0793-411a-9895-8f3ce17193a3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1c9d4136-0793-411a-9895-8f3ce17193a3") },
|
|
{ "237238a0-37d2-40b1-b85b-481da02c50b9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "a368e0dd-a146-4d42-a835-cce8d8fe068a"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "237238a0-37d2-40b1-b85b-481da02c50b9") },
|
|
{ "ad08eb42-95d9-40c5-80d2-c91eee28ac62", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "ad08eb42-95d9-40c5-80d2-c91eee28ac62") },
|
|
{ "2d3427fb-2c1f-411a-902b-fff3c4774ebe", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "2d3427fb-2c1f-411a-902b-fff3c4774ebe") },
|
|
{ "5417883a-4e03-4e45-a1c9-897b98a50c9c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "33990854-fada-4294-86be-eb1bee27d247"}, { new NonTerminator(NonTerminatorType.Term), "9fb34318-5a61-4087-9c5f-2382ce6fefbb"}, { new NonTerminator(NonTerminatorType.Factor), "41c2af4d-09f5-4b9b-9451-8cc42419d432"}, { Terminator.NumberTerminator, "2a86e144-9c92-4853-81a5-12888293d62f"}, { new NonTerminator(NonTerminatorType.Variable), "a7f71a4b-2095-4f79-a06f-6cdee4b7d59e"}, { new Terminator(DelimiterType.LeftParenthesis), "f881ba2a-5a64-4a41-beaa-22cb3030308a"}, { Terminator.IdentifierTerminator, "0f896225-a2c2-40c8-ba03-f80886ec77eb"}, { new Terminator(KeywordType.Not), "7dd7e043-b608-405e-99f9-7a031816ff16"}, { new Terminator(OperatorType.Minus), "c46d6d12-9c82-4d65-ae5a-b973857c1a90"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5417883a-4e03-4e45-a1c9-897b98a50c9c") },
|
|
{ "623f1f11-53c3-4917-8373-d9448dadbf72", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "4c9c1423-1228-420e-9e2b-182ec7720e2d"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "623f1f11-53c3-4917-8373-d9448dadbf72") },
|
|
{ "1efdc93c-1cf5-42ba-b76e-4913b093fe1e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "9740516a-f934-4433-865f-6c6f48bfcd06"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1efdc93c-1cf5-42ba-b76e-4913b093fe1e") },
|
|
{ "bbc42cc9-4082-4219-9bd8-62c653270c33", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "00709b79-a2ae-448e-a285-6c36bc3721df"},}, new Dictionary<Terminator, ReduceInformation>{ }, "bbc42cc9-4082-4219-9bd8-62c653270c33") },
|
|
{ "3aacd451-1def-4cf1-be9a-977567f01d08", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "f187fcd2-60cf-4c9d-af98-c9170910d212"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "3aacd451-1def-4cf1-be9a-977567f01d08") },
|
|
{ "bef05338-3156-4599-9a62-f4491d7c83be", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "bef05338-3156-4599-9a62-f4491d7c83be") },
|
|
{ "2c8d4e5a-c721-473a-a25b-a9457d94715c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "433446e7-f515-436d-ade7-77bb308b0d60"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "2c8d4e5a-c721-473a-a25b-a9457d94715c") },
|
|
{ "07375a4c-6fb3-4a10-9446-56ade98b9f97", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "07375a4c-6fb3-4a10-9446-56ade98b9f97") },
|
|
{ "d43d3c4f-71e8-4d92-b606-d7448fbe4fac", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "d43d3c4f-71e8-4d92-b606-d7448fbe4fac") },
|
|
{ "a35c1189-edfa-4093-bf69-3124685c5f26", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "5aa88840-b58f-4bef-a19e-6d9faf2f9f5f"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a35c1189-edfa-4093-bf69-3124685c5f26") },
|
|
{ "ad37ddff-25f5-4011-bf88-5ac4bd0cb2f1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "37d7c079-8c1a-402a-b6b3-2b4723abe4cb"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "ad37ddff-25f5-4011-bf88-5ac4bd0cb2f1") },
|
|
{ "5de3330d-a6c6-4099-8af9-cfb659d47b00", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.To), "03add803-2858-4eef-8330-b0cd874bf1c6"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5de3330d-a6c6-4099-8af9-cfb659d47b00") },
|
|
{ "bbdbc5e4-202b-4d40-a862-c9092cfb6c67", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "c2494b20-9ed6-4176-a127-ce126ef89513"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "6cefc87c-b2c3-430d-aea4-8239664aec25"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "bbdbc5e4-202b-4d40-a862-c9092cfb6c67") },
|
|
{ "fe68835b-da88-4eda-940c-19f73c430df7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "4415fae3-3f18-49e9-a5cd-26c4a00bcaf2"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "fe68835b-da88-4eda-940c-19f73c430df7") },
|
|
{ "3b214e0e-230c-481b-8c15-f304cc856f86", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "3b214e0e-230c-481b-8c15-f304cc856f86") },
|
|
{ "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4") },
|
|
{ "f56343e4-f729-4764-b769-0e133ee3a2f2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "f56343e4-f729-4764-b769-0e133ee3a2f2") },
|
|
{ "cdf73a03-7454-4da9-942e-e01adf2d6592", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "615f959c-98af-4f2d-93c8-9061f3713ce9"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "cdf73a03-7454-4da9-942e-e01adf2d6592") },
|
|
{ "38247e93-f150-453a-b02a-809c7b22ef1d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "3a80750a-ca4a-43b8-a145-0bc059ece8d0"}, { new NonTerminator(NonTerminatorType.IdVarPart), "b0b4d0a8-c647-4f2c-834d-9019f888f528"}, { new Terminator(DelimiterType.LeftSquareBracket), "7f857463-72a1-441a-b6e9-f8830df0a461"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "38247e93-f150-453a-b02a-809c7b22ef1d") },
|
|
{ "16b14840-8c2b-4856-a232-ebcc29c0f7a5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "15925aad-03ce-4409-96ef-ecc1c4adad25"}, { Terminator.NumberTerminator, "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4"}, { new NonTerminator(NonTerminatorType.Variable), "f56343e4-f729-4764-b769-0e133ee3a2f2"}, { new Terminator(DelimiterType.LeftParenthesis), "cdf73a03-7454-4da9-942e-e01adf2d6592"}, { Terminator.IdentifierTerminator, "38247e93-f150-453a-b02a-809c7b22ef1d"}, { new Terminator(KeywordType.Not), "16b14840-8c2b-4856-a232-ebcc29c0f7a5"}, { new Terminator(OperatorType.Minus), "f8243a3e-9016-47fc-88a3-453d416dc854"},}, new Dictionary<Terminator, ReduceInformation>{ }, "16b14840-8c2b-4856-a232-ebcc29c0f7a5") },
|
|
{ "f8243a3e-9016-47fc-88a3-453d416dc854", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "7b4e1505-ebb1-4bf4-a59f-fab3f1825c96"}, { Terminator.NumberTerminator, "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4"}, { new NonTerminator(NonTerminatorType.Variable), "f56343e4-f729-4764-b769-0e133ee3a2f2"}, { new Terminator(DelimiterType.LeftParenthesis), "cdf73a03-7454-4da9-942e-e01adf2d6592"}, { Terminator.IdentifierTerminator, "38247e93-f150-453a-b02a-809c7b22ef1d"}, { new Terminator(KeywordType.Not), "16b14840-8c2b-4856-a232-ebcc29c0f7a5"}, { new Terminator(OperatorType.Minus), "f8243a3e-9016-47fc-88a3-453d416dc854"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f8243a3e-9016-47fc-88a3-453d416dc854") },
|
|
{ "b33610ed-b390-486f-923a-714bf7147197", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SubprogramBody))}, }, "b33610ed-b390-486f-923a-714bf7147197") },
|
|
{ "91fa9734-aa7f-4b34-a139-cfa85c396cbc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.StatementList), "ca99f015-0575-4805-8126-12f9e488837b"}, { new NonTerminator(NonTerminatorType.Statement), "2dac9c20-107b-46e4-a18b-fdf58e625107"}, { new NonTerminator(NonTerminatorType.Variable), "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1"}, { Terminator.IdentifierTerminator, "2103d893-c079-4b22-81d8-4f9f358fe6ad"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "e53241b0-bb50-48d3-845a-063d9ecbbdeb"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a"}, { new Terminator(KeywordType.If), "c9145487-1b31-498c-9722-1f68bba31eab"}, { new Terminator(KeywordType.For), "d93a638a-474b-4cbd-93db-107fb792750f"}, { new Terminator(KeywordType.Begin), "bbe1d91e-d0c2-4be2-8184-4548587afa0e"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "91fa9734-aa7f-4b34-a139-cfa85c396cbc") },
|
|
{ "c10cd1be-21ef-4e0d-9722-d6c895dfbf79", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.Semicolon), "cd72dcad-a42e-4e0a-bfe4-e5db4099051f"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c10cd1be-21ef-4e0d-9722-d6c895dfbf79") },
|
|
{ "520f9af3-ed17-4e0e-aa24-a461c3d448a5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.IdentifierTerminator, "6f006c54-98dd-4024-a4cc-116e8e209e5c"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ConstDeclarations))}, { new Terminator(KeywordType.Var), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ConstDeclarations))}, }, "520f9af3-ed17-4e0e-aa24-a461c3d448a5") },
|
|
{ "a50aa3cb-351c-4216-a2ee-5fa1496ba01f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.FormalParameter))}, }, "a50aa3cb-351c-4216-a2ee-5fa1496ba01f") },
|
|
{ "24c2af8e-f211-40ca-b06e-af64dabc2315", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Parameter), "33745ff9-98fb-480d-be11-6da3074f5afe"}, { new NonTerminator(NonTerminatorType.VarParameter), "1a42389c-c349-4c5e-bfa3-d40f719e4a78"}, { new NonTerminator(NonTerminatorType.ValueParameter), "c4477610-5e29-42d3-89fd-ffd5c57feb6a"}, { new Terminator(KeywordType.Var), "2e97b21a-2473-4b7c-9d01-b2493627a1a2"}, { new NonTerminator(NonTerminatorType.IdentifierList), "a0b9084f-7330-4dbd-9874-7c12714a5e00"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ }, "24c2af8e-f211-40ca-b06e-af64dabc2315") },
|
|
{ "fa52306a-d38a-47a5-8a7f-23e2895334f5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.VarParameter))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.VarParameter))}, }, "fa52306a-d38a-47a5-8a7f-23e2895334f5") },
|
|
{ "f10e618d-59ed-4a23-b6dc-02f2d0f6dd3e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.BasicType), "4e55c2b0-2317-40f2-a25b-d6832b55a801"}, { new Terminator(KeywordType.Integer), "899d09a4-360d-40f9-8d3d-54cf9ea5cbaf"}, { new Terminator(KeywordType.Real), "7841c200-6b8f-472d-bb62-465fe55427c7"}, { new Terminator(KeywordType.Boolean), "76219417-fc31-4a52-ad31-38f963df5a69"}, { new Terminator(KeywordType.Character), "b52a8d40-72d7-4552-82ee-7a3d91d61771"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f10e618d-59ed-4a23-b6dc-02f2d0f6dd3e") },
|
|
{ "1c605559-8a9b-45b5-a03c-29c01028439c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(5, new NonTerminator(NonTerminatorType.SubprogramHead))}, }, "1c605559-8a9b-45b5-a03c-29c01028439c") },
|
|
{ "4a95c5d4-3386-4dd6-84eb-c010dd493b55", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Colon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.FormalParameter))}, }, "4a95c5d4-3386-4dd6-84eb-c010dd493b55") },
|
|
{ "b2c7444b-c362-4975-8612-431738df31e6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.Of), "0ae666a3-545c-40a1-8f83-7b9f9d0d8bbc"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b2c7444b-c362-4975-8612-431738df31e6") },
|
|
{ "36b4ab41-2d5d-40cb-9add-0a4ccd140262", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.NumberTerminator, "9f944ebf-f3fc-4842-8686-772d29fe8f7c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "36b4ab41-2d5d-40cb-9add-0a4ccd140262") },
|
|
{ "313f579d-7a53-4d48-bbcc-41609243ae15", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.NumberTerminator, "37dd900d-c913-4ce5-a8c5-40e88a68f4da"},}, new Dictionary<Terminator, ReduceInformation>{ }, "313f579d-7a53-4d48-bbcc-41609243ae15") },
|
|
{ "ccf04ef5-d768-4039-9177-7c358b075e35", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "76d184c8-f3c5-4f2c-ad43-0fd59d75a2a7"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "ccf04ef5-d768-4039-9177-7c358b075e35") },
|
|
{ "709c6303-5083-4422-a7e5-cb3066d1aefd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "2661dbe7-08ca-4463-8892-ffdc1673793c"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "709c6303-5083-4422-a7e5-cb3066d1aefd") },
|
|
{ "840498d9-7584-4b94-9182-f56adfb0a56d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "840498d9-7584-4b94-9182-f56adfb0a56d") },
|
|
{ "a94e0a8a-3c31-4b45-b757-8a1e6b19e511", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "a94e0a8a-3c31-4b45-b757-8a1e6b19e511") },
|
|
{ "ca7cefdc-b17f-4e84-8b42-829590b1110e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "ca7cefdc-b17f-4e84-8b42-829590b1110e") },
|
|
{ "9019af5d-8a33-44d4-954c-d8f218b3ec5e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "0874f0b7-49ef-427a-b0c4-d12a0d5c1a9b"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "9019af5d-8a33-44d4-954c-d8f218b3ec5e") },
|
|
{ "f0064b59-541c-4e17-b719-937423bb36c5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "c4ff5ec8-5acf-4a99-9762-32cf249cbadc"}, { new NonTerminator(NonTerminatorType.IdVarPart), "c26a9885-06bd-4ffe-87dd-c8872257b2dc"}, { new Terminator(DelimiterType.LeftSquareBracket), "38ed7061-3592-4788-b5da-1f6869b6d716"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "f0064b59-541c-4e17-b719-937423bb36c5") },
|
|
{ "6196e383-c5cd-4279-9cbb-85bd6bdc1de4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "3ff56b4c-9b39-447d-b705-f69f52b22398"}, { Terminator.NumberTerminator, "a94e0a8a-3c31-4b45-b757-8a1e6b19e511"}, { new NonTerminator(NonTerminatorType.Variable), "ca7cefdc-b17f-4e84-8b42-829590b1110e"}, { new Terminator(DelimiterType.LeftParenthesis), "9019af5d-8a33-44d4-954c-d8f218b3ec5e"}, { Terminator.IdentifierTerminator, "f0064b59-541c-4e17-b719-937423bb36c5"}, { new Terminator(KeywordType.Not), "6196e383-c5cd-4279-9cbb-85bd6bdc1de4"}, { new Terminator(OperatorType.Minus), "323aa85f-eb14-4398-a5a6-e62e621aa1c2"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6196e383-c5cd-4279-9cbb-85bd6bdc1de4") },
|
|
{ "323aa85f-eb14-4398-a5a6-e62e621aa1c2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "43857d72-45ac-4b06-9cd5-da183e56897f"}, { Terminator.NumberTerminator, "a94e0a8a-3c31-4b45-b757-8a1e6b19e511"}, { new NonTerminator(NonTerminatorType.Variable), "ca7cefdc-b17f-4e84-8b42-829590b1110e"}, { new Terminator(DelimiterType.LeftParenthesis), "9019af5d-8a33-44d4-954c-d8f218b3ec5e"}, { Terminator.IdentifierTerminator, "f0064b59-541c-4e17-b719-937423bb36c5"}, { new Terminator(KeywordType.Not), "6196e383-c5cd-4279-9cbb-85bd6bdc1de4"}, { new Terminator(OperatorType.Minus), "323aa85f-eb14-4398-a5a6-e62e621aa1c2"},}, new Dictionary<Terminator, ReduceInformation>{ }, "323aa85f-eb14-4398-a5a6-e62e621aa1c2") },
|
|
{ "c4307f56-7e81-4055-8987-ed9826ee70e3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "cbb0931d-780c-46fa-a970-670acfbb3cac"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "c4307f56-7e81-4055-8987-ed9826ee70e3") },
|
|
{ "3a86cef5-67a4-4910-ae34-6ddd3e209ac6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "3a86cef5-67a4-4910-ae34-6ddd3e209ac6") },
|
|
{ "6492230d-2e12-4609-bc2a-cbc4f91c8820", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "6492230d-2e12-4609-bc2a-cbc4f91c8820") },
|
|
{ "37939423-f4db-4ab7-9e15-d8e30c811a8d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "f445b0cf-2c90-4e6e-8da7-63d6423333f4"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "37939423-f4db-4ab7-9e15-d8e30c811a8d") },
|
|
{ "d04a50f5-c163-4c1e-b9db-cefaf42fd995", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "50138a80-e45e-4501-a646-80513240b0c0"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d04a50f5-c163-4c1e-b9db-cefaf42fd995") },
|
|
{ "ec00a7c3-8437-4547-a444-1d97e6260c39", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ExpressionList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ExpressionList))}, }, "ec00a7c3-8437-4547-a444-1d97e6260c39") },
|
|
{ "12f85add-4cb2-4579-9dec-602ba96c0474", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "e46147c9-003b-46cb-9997-24feeb2ca815"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "12f85add-4cb2-4579-9dec-602ba96c0474") },
|
|
{ "ca87ba4b-609f-4b45-9779-7a40f73c239b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "49ff32c8-99bc-4459-9f3f-33612a86266a"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "ca87ba4b-609f-4b45-9779-7a40f73c239b") },
|
|
{ "51f72fb0-d985-4bc6-b47a-9ea0753d7907", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "51f72fb0-d985-4bc6-b47a-9ea0753d7907") },
|
|
{ "13ca9c1b-760f-4da0-878b-72b23c8a6a55", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "13ca9c1b-760f-4da0-878b-72b23c8a6a55") },
|
|
{ "35529643-d0ce-4527-ad73-841e6868903b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "35529643-d0ce-4527-ad73-841e6868903b") },
|
|
{ "9b07659a-c168-4c5b-8781-b6c11ea204a0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "d67fe0fd-82e0-4693-a7bd-35d11394462b"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "9b07659a-c168-4c5b-8781-b6c11ea204a0") },
|
|
{ "df0def68-2aa5-49b6-8869-9e2d30b4c48d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "d3aecd1f-ce29-4754-a1ad-7d3e19ed6753"}, { new NonTerminator(NonTerminatorType.IdVarPart), "beea9760-bdb9-4e65-86ed-3a90de4c9b65"}, { new Terminator(DelimiterType.LeftSquareBracket), "840c3d0e-b902-4cb8-a5e2-109ea93e1775"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "df0def68-2aa5-49b6-8869-9e2d30b4c48d") },
|
|
{ "fec38012-07b5-4ba8-ae88-a68a714f1cbc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "e0e375a3-617f-459c-a805-f9079e5003a8"}, { Terminator.NumberTerminator, "13ca9c1b-760f-4da0-878b-72b23c8a6a55"}, { new NonTerminator(NonTerminatorType.Variable), "35529643-d0ce-4527-ad73-841e6868903b"}, { new Terminator(DelimiterType.LeftParenthesis), "9b07659a-c168-4c5b-8781-b6c11ea204a0"}, { Terminator.IdentifierTerminator, "df0def68-2aa5-49b6-8869-9e2d30b4c48d"}, { new Terminator(KeywordType.Not), "fec38012-07b5-4ba8-ae88-a68a714f1cbc"}, { new Terminator(OperatorType.Minus), "727581eb-84d2-411d-9872-e367a0ccc78c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "fec38012-07b5-4ba8-ae88-a68a714f1cbc") },
|
|
{ "727581eb-84d2-411d-9872-e367a0ccc78c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "c0520c10-db88-4872-ab61-6920951067b9"}, { Terminator.NumberTerminator, "13ca9c1b-760f-4da0-878b-72b23c8a6a55"}, { new NonTerminator(NonTerminatorType.Variable), "35529643-d0ce-4527-ad73-841e6868903b"}, { new Terminator(DelimiterType.LeftParenthesis), "9b07659a-c168-4c5b-8781-b6c11ea204a0"}, { Terminator.IdentifierTerminator, "df0def68-2aa5-49b6-8869-9e2d30b4c48d"}, { new Terminator(KeywordType.Not), "fec38012-07b5-4ba8-ae88-a68a714f1cbc"}, { new Terminator(OperatorType.Minus), "727581eb-84d2-411d-9872-e367a0ccc78c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "727581eb-84d2-411d-9872-e367a0ccc78c") },
|
|
{ "e5f440de-e88c-4b32-8fd0-9e244b30d5fc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "c254eae9-8d04-4e4a-8df3-458b09013efe"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "e5f440de-e88c-4b32-8fd0-9e244b30d5fc") },
|
|
{ "7bcbb8fd-e886-4575-8412-00b4a9da4191", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "7bcbb8fd-e886-4575-8412-00b4a9da4191") },
|
|
{ "4cb65c7a-7ebe-4ee8-aa87-bddbd75caeb9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "4cb65c7a-7ebe-4ee8-aa87-bddbd75caeb9") },
|
|
{ "5ae12e51-d94e-425b-b223-5a12afaeda8b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "730ac0f0-9ffb-4894-92f0-459e7a0488be"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5ae12e51-d94e-425b-b223-5a12afaeda8b") },
|
|
{ "03b1c5aa-6683-4422-9316-0f3f3d213c54", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "cfb76852-2969-4a72-b98f-22405390bc96"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "03b1c5aa-6683-4422-9316-0f3f3d213c54") },
|
|
{ "7e357ece-1708-4c9d-8a54-84b4256bcfdd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ExpressionList))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ExpressionList))}, }, "7e357ece-1708-4c9d-8a54-84b4256bcfdd") },
|
|
{ "61d911f0-a8fc-4bc9-87d9-b58ce3f9f5cb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "a309e6b0-3089-48de-b8c3-168b663f3c14"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "61d911f0-a8fc-4bc9-87d9-b58ce3f9f5cb") },
|
|
{ "6c17a0f8-afa7-4e7a-8934-0216c55df6c7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "73a2f83c-7090-409b-92cf-40f354be432c"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "6c17a0f8-afa7-4e7a-8934-0216c55df6c7") },
|
|
{ "f0d0fcd0-1d8a-405f-9354-a994b496f73d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "f0d0fcd0-1d8a-405f-9354-a994b496f73d") },
|
|
{ "3ffc269a-694c-4697-9e31-73f752ab9d1f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "3ffc269a-694c-4697-9e31-73f752ab9d1f") },
|
|
{ "188a892f-e2b1-465e-85e1-67451cbfe493", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "188a892f-e2b1-465e-85e1-67451cbfe493") },
|
|
{ "29f01b0d-b7fb-46f9-9522-5c1dbec0b06c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "fd1b66b4-6a27-4c1e-92d0-02331e7332c5"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "29f01b0d-b7fb-46f9-9522-5c1dbec0b06c") },
|
|
{ "8306e662-fca1-48d9-bedf-bd4bc289518c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "5e5cb6ae-e0d0-44e8-9309-8111917a2ca4"}, { new NonTerminator(NonTerminatorType.IdVarPart), "6668df6d-36ea-4f84-831f-60257550db2d"}, { new Terminator(DelimiterType.LeftSquareBracket), "842797a0-655c-4fd4-8300-fe1f30f1842c"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "8306e662-fca1-48d9-bedf-bd4bc289518c") },
|
|
{ "393677af-7726-490c-9f16-7587445de9f6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "1b2e99a6-f206-4af6-a9f5-13c590b6f3a6"}, { Terminator.NumberTerminator, "3ffc269a-694c-4697-9e31-73f752ab9d1f"}, { new NonTerminator(NonTerminatorType.Variable), "188a892f-e2b1-465e-85e1-67451cbfe493"}, { new Terminator(DelimiterType.LeftParenthesis), "29f01b0d-b7fb-46f9-9522-5c1dbec0b06c"}, { Terminator.IdentifierTerminator, "8306e662-fca1-48d9-bedf-bd4bc289518c"}, { new Terminator(KeywordType.Not), "393677af-7726-490c-9f16-7587445de9f6"}, { new Terminator(OperatorType.Minus), "653e16fa-8ba0-4f39-8e14-063ba0fcc359"},}, new Dictionary<Terminator, ReduceInformation>{ }, "393677af-7726-490c-9f16-7587445de9f6") },
|
|
{ "653e16fa-8ba0-4f39-8e14-063ba0fcc359", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "afdf0327-a331-4543-8f0b-b5f54f38505c"}, { Terminator.NumberTerminator, "3ffc269a-694c-4697-9e31-73f752ab9d1f"}, { new NonTerminator(NonTerminatorType.Variable), "188a892f-e2b1-465e-85e1-67451cbfe493"}, { new Terminator(DelimiterType.LeftParenthesis), "29f01b0d-b7fb-46f9-9522-5c1dbec0b06c"}, { Terminator.IdentifierTerminator, "8306e662-fca1-48d9-bedf-bd4bc289518c"}, { new Terminator(KeywordType.Not), "393677af-7726-490c-9f16-7587445de9f6"}, { new Terminator(OperatorType.Minus), "653e16fa-8ba0-4f39-8e14-063ba0fcc359"},}, new Dictionary<Terminator, ReduceInformation>{ }, "653e16fa-8ba0-4f39-8e14-063ba0fcc359") },
|
|
{ "0f34877d-5028-4e35-bae5-f71fab556329", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "5e0fd40a-6707-48b6-8187-e404dc744a7b"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "0f34877d-5028-4e35-bae5-f71fab556329") },
|
|
{ "e04d7719-7961-41ef-877f-76c3f1e9c07d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "e04d7719-7961-41ef-877f-76c3f1e9c07d") },
|
|
{ "ee04eb34-e49b-4496-80b9-837ace95dfb7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "ee04eb34-e49b-4496-80b9-837ace95dfb7") },
|
|
{ "e00991ec-e31d-459e-924f-3dd992323cd8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "5e50fd88-93bf-4757-9ef1-4b8269eb2172"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e00991ec-e31d-459e-924f-3dd992323cd8") },
|
|
{ "c7f27ef7-8244-4eb9-8d74-75b0a048adcb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "045382bc-9dfb-4b0d-a6e2-325681ac646a"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c7f27ef7-8244-4eb9-8d74-75b0a048adcb") },
|
|
{ "99ec1fc2-97e4-4a1e-9624-2c7a38b38093", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(5, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(5, new NonTerminator(NonTerminatorType.Statement))}, }, "99ec1fc2-97e4-4a1e-9624-2c7a38b38093") },
|
|
{ "7d267d53-3ce0-4d70-9b6c-0621875ae971", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Statement), "3a004d15-8f52-4adf-8832-53d0ed1c8429"}, { new NonTerminator(NonTerminatorType.Variable), "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1"}, { Terminator.IdentifierTerminator, "2103d893-c079-4b22-81d8-4f9f358fe6ad"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "e53241b0-bb50-48d3-845a-063d9ecbbdeb"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a"}, { new Terminator(KeywordType.If), "c9145487-1b31-498c-9722-1f68bba31eab"}, { new Terminator(KeywordType.For), "d93a638a-474b-4cbd-93db-107fb792750f"}, { new Terminator(KeywordType.Begin), "bbe1d91e-d0c2-4be2-8184-4548587afa0e"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "7d267d53-3ce0-4d70-9b6c-0621875ae971") },
|
|
{ "e7cca867-263f-4ac7-a7a8-33bff1f48284", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "fa653631-6014-4fad-a34d-ae04caa6e03b"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "f64f7d06-6e83-4b4a-afa4-12d820344a4a"}, { new NonTerminator(NonTerminatorType.Term), "140aa1c3-e73d-4c29-802a-e241fda55485"}, { new NonTerminator(NonTerminatorType.Factor), "5f286aeb-87b1-4cd2-8227-dacae01f5851"}, { Terminator.NumberTerminator, "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4"}, { new NonTerminator(NonTerminatorType.Variable), "d8bb860c-d107-4a56-96fb-7e7e70063536"}, { new Terminator(DelimiterType.LeftParenthesis), "33fd746c-385d-4bb0-9e51-902f3d247131"}, { Terminator.IdentifierTerminator, "152863ec-9b47-42ca-8fee-391ace4b9f5e"}, { new Terminator(KeywordType.Not), "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae"}, { new Terminator(OperatorType.Minus), "3db16667-f163-45df-a53a-a2b4c37906fb"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e7cca867-263f-4ac7-a7a8-33bff1f48284") },
|
|
{ "cd8c7020-b7cb-4d2c-89e2-43a1e972dfb9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "3065f96f-b964-4da0-a1e2-051c00df4238"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "f64f7d06-6e83-4b4a-afa4-12d820344a4a"}, { new NonTerminator(NonTerminatorType.Term), "140aa1c3-e73d-4c29-802a-e241fda55485"}, { new NonTerminator(NonTerminatorType.Factor), "5f286aeb-87b1-4cd2-8227-dacae01f5851"}, { Terminator.NumberTerminator, "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4"}, { new NonTerminator(NonTerminatorType.Variable), "d8bb860c-d107-4a56-96fb-7e7e70063536"}, { new Terminator(DelimiterType.LeftParenthesis), "33fd746c-385d-4bb0-9e51-902f3d247131"}, { Terminator.IdentifierTerminator, "152863ec-9b47-42ca-8fee-391ace4b9f5e"}, { new Terminator(KeywordType.Not), "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae"}, { new Terminator(OperatorType.Minus), "3db16667-f163-45df-a53a-a2b4c37906fb"},}, new Dictionary<Terminator, ReduceInformation>{ }, "cd8c7020-b7cb-4d2c-89e2-43a1e972dfb9") },
|
|
{ "8cba8140-28f5-4468-a66e-3e1c1907d8e6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "1aac137b-8eba-4ca3-8652-3d230112e2a0"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8cba8140-28f5-4468-a66e-3e1c1907d8e6") },
|
|
{ "e4f1500c-cfbc-48c9-bcff-0c33500e01c7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.Then), "b25d1052-dd35-4688-9a47-0507f51cfd6f"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e4f1500c-cfbc-48c9-bcff-0c33500e01c7") },
|
|
{ "664bbdbe-f6d7-465e-9391-dbcd925093e8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(OperatorType.Assign), "c6a9f364-68dd-4012-ab77-379f7603f641"},}, new Dictionary<Terminator, ReduceInformation>{ }, "664bbdbe-f6d7-465e-9391-dbcd925093e8") },
|
|
{ "88d16383-f68f-4824-87d9-bd2134c94983", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.End), "49a7e4fa-6e01-4023-907b-60286af9256a"}, { new Terminator(DelimiterType.Semicolon), "ca1783b9-fb61-4fbb-886b-0d1de39ddf7d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "88d16383-f68f-4824-87d9-bd2134c94983") },
|
|
{ "f2998caf-7e35-441c-ade5-0e046846bae4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "bd6b6663-2cce-483e-9d19-c61be1d729f3"}, { new NonTerminator(NonTerminatorType.Factor), "86726642-31b6-468b-97d8-aa0bd78b5290"}, { Terminator.NumberTerminator, "b5045e69-5b08-443a-a4dd-bcfc531ec151"}, { new NonTerminator(NonTerminatorType.Variable), "e667abdf-5bac-4bd4-ba6d-30a41ebda6b1"}, { new Terminator(DelimiterType.LeftParenthesis), "fac69cb2-c9e2-489c-a5f6-aeacd11a1701"}, { Terminator.IdentifierTerminator, "6980c787-4656-4f35-adcd-321bfabbbd05"}, { new Terminator(KeywordType.Not), "75de4e67-0e89-435b-8b07-17bc55e72853"}, { new Terminator(OperatorType.Minus), "1c9d4136-0793-411a-9895-8f3ce17193a3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f2998caf-7e35-441c-ade5-0e046846bae4") },
|
|
{ "2c40c3e6-9e5d-4a04-9a03-d54fa03e9fd6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "bb7afe54-cf30-4686-bcb5-9cc28aea6f05"}, { Terminator.NumberTerminator, "b5045e69-5b08-443a-a4dd-bcfc531ec151"}, { new NonTerminator(NonTerminatorType.Variable), "e667abdf-5bac-4bd4-ba6d-30a41ebda6b1"}, { new Terminator(DelimiterType.LeftParenthesis), "fac69cb2-c9e2-489c-a5f6-aeacd11a1701"}, { Terminator.IdentifierTerminator, "6980c787-4656-4f35-adcd-321bfabbbd05"}, { new Terminator(KeywordType.Not), "75de4e67-0e89-435b-8b07-17bc55e72853"}, { new Terminator(OperatorType.Minus), "1c9d4136-0793-411a-9895-8f3ce17193a3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "2c40c3e6-9e5d-4a04-9a03-d54fa03e9fd6") },
|
|
{ "6a6374aa-3719-4edb-b619-44b5b94f59f0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "8c7fb4d8-adbc-4a32-88e8-fa21d0091d18"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6a6374aa-3719-4edb-b619-44b5b94f59f0") },
|
|
{ "5cd1041b-f882-46c0-90fb-99adcd19f617", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "a8bed40a-3e8e-4852-81b4-203f2c0f31f4"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5cd1041b-f882-46c0-90fb-99adcd19f617") },
|
|
{ "3e572cc3-8db3-4bd0-83a1-c5006f6e8c02", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "3e572cc3-8db3-4bd0-83a1-c5006f6e8c02") },
|
|
{ "8bedebe5-33b9-43e8-b0b5-cad41bcccd6f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "392887a7-7346-422b-842c-6b02c94db7aa"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8bedebe5-33b9-43e8-b0b5-cad41bcccd6f") },
|
|
{ "0cd606f3-0bf2-45f7-9ebf-da3722a4a606", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "0cd606f3-0bf2-45f7-9ebf-da3722a4a606") },
|
|
{ "e303b300-36f4-4cd7-ab84-5f25c89bef58", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "e303b300-36f4-4cd7-ab84-5f25c89bef58") },
|
|
{ "33990854-fada-4294-86be-eb1bee27d247", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "0d952e95-ce57-44c2-846a-170950662e9a"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "33990854-fada-4294-86be-eb1bee27d247") },
|
|
{ "9fb34318-5a61-4087-9c5f-2382ce6fefbb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "7b21201b-8255-4261-9742-587d3c0a4bc6"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "9fb34318-5a61-4087-9c5f-2382ce6fefbb") },
|
|
{ "41c2af4d-09f5-4b9b-9451-8cc42419d432", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "41c2af4d-09f5-4b9b-9451-8cc42419d432") },
|
|
{ "2a86e144-9c92-4853-81a5-12888293d62f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "2a86e144-9c92-4853-81a5-12888293d62f") },
|
|
{ "a7f71a4b-2095-4f79-a06f-6cdee4b7d59e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "a7f71a4b-2095-4f79-a06f-6cdee4b7d59e") },
|
|
{ "f881ba2a-5a64-4a41-beaa-22cb3030308a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "27768c95-ff12-4fac-aa55-9c5b9e361de8"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f881ba2a-5a64-4a41-beaa-22cb3030308a") },
|
|
{ "0f896225-a2c2-40c8-ba03-f80886ec77eb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "e8a5d434-72a4-46e9-9bd9-d78af731ef73"}, { new NonTerminator(NonTerminatorType.IdVarPart), "49e62c47-cb9e-44ca-9eef-b43dea6723d4"}, { new Terminator(DelimiterType.LeftSquareBracket), "d46259bb-80c9-4ffd-9c24-9e7bae78add5"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "0f896225-a2c2-40c8-ba03-f80886ec77eb") },
|
|
{ "7dd7e043-b608-405e-99f9-7a031816ff16", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "39e72570-0e67-4611-bd9a-0470e815b89e"}, { Terminator.NumberTerminator, "2a86e144-9c92-4853-81a5-12888293d62f"}, { new NonTerminator(NonTerminatorType.Variable), "a7f71a4b-2095-4f79-a06f-6cdee4b7d59e"}, { new Terminator(DelimiterType.LeftParenthesis), "f881ba2a-5a64-4a41-beaa-22cb3030308a"}, { Terminator.IdentifierTerminator, "0f896225-a2c2-40c8-ba03-f80886ec77eb"}, { new Terminator(KeywordType.Not), "7dd7e043-b608-405e-99f9-7a031816ff16"}, { new Terminator(OperatorType.Minus), "c46d6d12-9c82-4d65-ae5a-b973857c1a90"},}, new Dictionary<Terminator, ReduceInformation>{ }, "7dd7e043-b608-405e-99f9-7a031816ff16") },
|
|
{ "c46d6d12-9c82-4d65-ae5a-b973857c1a90", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "8a01dda9-1cc2-4878-95c9-df49efe5b0b4"}, { Terminator.NumberTerminator, "2a86e144-9c92-4853-81a5-12888293d62f"}, { new NonTerminator(NonTerminatorType.Variable), "a7f71a4b-2095-4f79-a06f-6cdee4b7d59e"}, { new Terminator(DelimiterType.LeftParenthesis), "f881ba2a-5a64-4a41-beaa-22cb3030308a"}, { Terminator.IdentifierTerminator, "0f896225-a2c2-40c8-ba03-f80886ec77eb"}, { new Terminator(KeywordType.Not), "7dd7e043-b608-405e-99f9-7a031816ff16"}, { new Terminator(OperatorType.Minus), "c46d6d12-9c82-4d65-ae5a-b973857c1a90"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c46d6d12-9c82-4d65-ae5a-b973857c1a90") },
|
|
{ "4c9c1423-1228-420e-9e2b-182ec7720e2d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "1efdc93c-1cf5-42ba-b76e-4913b093fe1e"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "4c9c1423-1228-420e-9e2b-182ec7720e2d") },
|
|
{ "9740516a-f934-4433-865f-6c6f48bfcd06", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "9740516a-f934-4433-865f-6c6f48bfcd06") },
|
|
{ "00709b79-a2ae-448e-a285-6c36bc3721df", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "00709b79-a2ae-448e-a285-6c36bc3721df") },
|
|
{ "f187fcd2-60cf-4c9d-af98-c9170910d212", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "fdbb53de-4335-4d3e-b784-3b7c1b262625"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f187fcd2-60cf-4c9d-af98-c9170910d212") },
|
|
{ "433446e7-f515-436d-ade7-77bb308b0d60", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "1491bbfe-601a-4191-b45a-1bb10d7d18d5"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "433446e7-f515-436d-ade7-77bb308b0d60") },
|
|
{ "5aa88840-b58f-4bef-a19e-6d9faf2f9f5f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "5aa88840-b58f-4bef-a19e-6d9faf2f9f5f") },
|
|
{ "37d7c079-8c1a-402a-b6b3-2b4723abe4cb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "37d7c079-8c1a-402a-b6b3-2b4723abe4cb") },
|
|
{ "03add803-2858-4eef-8330-b0cd874bf1c6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "4f2176aa-ff6e-477c-a812-a0b2c3a1d64c"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "80dcc69e-4b6c-47a7-806d-2d6042789558"}, { new NonTerminator(NonTerminatorType.Term), "b6f0cf51-dc0f-42b9-b4be-bf32b9a5a91a"}, { new NonTerminator(NonTerminatorType.Factor), "fb30bf0b-a6da-4520-bbf4-f7bde327e205"}, { Terminator.NumberTerminator, "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927"}, { new NonTerminator(NonTerminatorType.Variable), "3e0807fb-f778-41ce-bc91-57646ba8a4fd"}, { new Terminator(DelimiterType.LeftParenthesis), "92c717a5-062e-4ee9-8b6b-83979d1b8f55"}, { Terminator.IdentifierTerminator, "c0348957-d7d9-46c4-8066-60e86de6a120"}, { new Terminator(KeywordType.Not), "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81"}, { new Terminator(OperatorType.Minus), "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4"},}, new Dictionary<Terminator, ReduceInformation>{ }, "03add803-2858-4eef-8330-b0cd874bf1c6") },
|
|
{ "c2494b20-9ed6-4176-a127-ce126ef89513", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "5bf62974-385a-46c4-ab7f-efef13e98440"}, { new NonTerminator(NonTerminatorType.Term), "750206ac-afc5-4a52-bba3-ac748d93cf32"}, { new NonTerminator(NonTerminatorType.Factor), "a69cd9ad-c893-4b4f-be74-0fc3cd420ddb"}, { Terminator.NumberTerminator, "c1cac270-eb37-4944-a5f2-4fbf46250915"}, { new NonTerminator(NonTerminatorType.Variable), "7f8b9161-eadb-41c1-bdf3-449db72c2486"}, { new Terminator(DelimiterType.LeftParenthesis), "8858a3cf-c573-42ff-9f6f-1c14e693c759"}, { Terminator.IdentifierTerminator, "8f80a93b-1f9c-4827-851d-25e5bce2798b"}, { new Terminator(KeywordType.Not), "c8e9b2a6-afee-4f2e-9646-74cbba6b2f65"}, { new Terminator(OperatorType.Minus), "b1eb6db3-a55a-4399-a287-469148a5eef3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c2494b20-9ed6-4176-a127-ce126ef89513") },
|
|
{ "6cefc87c-b2c3-430d-aea4-8239664aec25", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "02e11df1-da73-441a-92b5-8057844e68a3"}, { new NonTerminator(NonTerminatorType.Factor), "3b214e0e-230c-481b-8c15-f304cc856f86"}, { Terminator.NumberTerminator, "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4"}, { new NonTerminator(NonTerminatorType.Variable), "f56343e4-f729-4764-b769-0e133ee3a2f2"}, { new Terminator(DelimiterType.LeftParenthesis), "cdf73a03-7454-4da9-942e-e01adf2d6592"}, { Terminator.IdentifierTerminator, "38247e93-f150-453a-b02a-809c7b22ef1d"}, { new Terminator(KeywordType.Not), "16b14840-8c2b-4856-a232-ebcc29c0f7a5"}, { new Terminator(OperatorType.Minus), "f8243a3e-9016-47fc-88a3-453d416dc854"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6cefc87c-b2c3-430d-aea4-8239664aec25") },
|
|
{ "4415fae3-3f18-49e9-a5cd-26c4a00bcaf2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "7f26b8c0-ea67-477b-84e6-343ffd2923dd"}, { Terminator.NumberTerminator, "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4"}, { new NonTerminator(NonTerminatorType.Variable), "f56343e4-f729-4764-b769-0e133ee3a2f2"}, { new Terminator(DelimiterType.LeftParenthesis), "cdf73a03-7454-4da9-942e-e01adf2d6592"}, { Terminator.IdentifierTerminator, "38247e93-f150-453a-b02a-809c7b22ef1d"}, { new Terminator(KeywordType.Not), "16b14840-8c2b-4856-a232-ebcc29c0f7a5"}, { new Terminator(OperatorType.Minus), "f8243a3e-9016-47fc-88a3-453d416dc854"},}, new Dictionary<Terminator, ReduceInformation>{ }, "4415fae3-3f18-49e9-a5cd-26c4a00bcaf2") },
|
|
{ "615f959c-98af-4f2d-93c8-9061f3713ce9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "0c17baef-2b4b-450e-b245-5cd843ef405f"},}, new Dictionary<Terminator, ReduceInformation>{ }, "615f959c-98af-4f2d-93c8-9061f3713ce9") },
|
|
{ "3a80750a-ca4a-43b8-a145-0bc059ece8d0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "77fc79a0-c31e-48df-bd18-0ea14a034ba1"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "3a80750a-ca4a-43b8-a145-0bc059ece8d0") },
|
|
{ "b0b4d0a8-c647-4f2c-834d-9019f888f528", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "b0b4d0a8-c647-4f2c-834d-9019f888f528") },
|
|
{ "7f857463-72a1-441a-b6e9-f8830df0a461", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "fe879b03-0748-4f76-b081-9196cf3a5684"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "7f857463-72a1-441a-b6e9-f8830df0a461") },
|
|
{ "15925aad-03ce-4409-96ef-ecc1c4adad25", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "15925aad-03ce-4409-96ef-ecc1c4adad25") },
|
|
{ "7b4e1505-ebb1-4bf4-a59f-fab3f1825c96", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "7b4e1505-ebb1-4bf4-a59f-fab3f1825c96") },
|
|
{ "ca99f015-0575-4805-8126-12f9e488837b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.End), "359234a1-0f34-4d0f-b65b-e6b4b5aa7a8a"}, { new Terminator(DelimiterType.Semicolon), "ca1783b9-fb61-4fbb-886b-0d1de39ddf7d"},}, new Dictionary<Terminator, ReduceInformation>{ }, "ca99f015-0575-4805-8126-12f9e488837b") },
|
|
{ "cd72dcad-a42e-4e0a-bfe4-e5db4099051f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.IdentifierList), "14a84860-7a46-4421-bb73-a5dda0faf29b"}, { Terminator.IdentifierTerminator, "1620bbb5-297a-47ec-97c7-4d1826507adf"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Begin), new ReduceInformation(3, new NonTerminator(NonTerminatorType.VarDeclarations))}, }, "cd72dcad-a42e-4e0a-bfe4-e5db4099051f") },
|
|
{ "33745ff9-98fb-480d-be11-6da3074f5afe", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ParameterList))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ParameterList))}, }, "33745ff9-98fb-480d-be11-6da3074f5afe") },
|
|
{ "4e55c2b0-2317-40f2-a25b-d6832b55a801", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ValueParameter))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.ValueParameter))}, }, "4e55c2b0-2317-40f2-a25b-d6832b55a801") },
|
|
{ "899d09a4-360d-40f9-8d3d-54cf9ea5cbaf", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "899d09a4-360d-40f9-8d3d-54cf9ea5cbaf") },
|
|
{ "7841c200-6b8f-472d-bb62-465fe55427c7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "7841c200-6b8f-472d-bb62-465fe55427c7") },
|
|
{ "76219417-fc31-4a52-ad31-38f963df5a69", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "76219417-fc31-4a52-ad31-38f963df5a69") },
|
|
{ "b52a8d40-72d7-4552-82ee-7a3d91d61771", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.BasicType))}, }, "b52a8d40-72d7-4552-82ee-7a3d91d61771") },
|
|
{ "0ae666a3-545c-40a1-8f83-7b9f9d0d8bbc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.BasicType), "52d8892a-4e66-443a-90c7-e78d08bc6940"}, { new Terminator(KeywordType.Integer), "d649770f-216c-448b-9cbe-ba827526a2f5"}, { new Terminator(KeywordType.Real), "741f0bed-843f-4135-90f4-4fa0337a7126"}, { new Terminator(KeywordType.Boolean), "981256c3-20fb-44ce-b5ab-9e3a26a98417"}, { new Terminator(KeywordType.Character), "e12136f0-98e7-4894-abb2-8671ea2331c7"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0ae666a3-545c-40a1-8f83-7b9f9d0d8bbc") },
|
|
{ "9f944ebf-f3fc-4842-8686-772d29fe8f7c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.DoubleDots), "5019768d-6250-47ae-a7b6-d22b6ee1fbea"},}, new Dictionary<Terminator, ReduceInformation>{ }, "9f944ebf-f3fc-4842-8686-772d29fe8f7c") },
|
|
{ "37dd900d-c913-4ce5-a8c5-40e88a68f4da", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Period))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Period))}, }, "37dd900d-c913-4ce5-a8c5-40e88a68f4da") },
|
|
{ "76d184c8-f3c5-4f2c-ad43-0fd59d75a2a7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "cd3c2c4c-b44a-47e2-b2af-183065962f24"}, { new NonTerminator(NonTerminatorType.Factor), "840498d9-7584-4b94-9182-f56adfb0a56d"}, { Terminator.NumberTerminator, "a94e0a8a-3c31-4b45-b757-8a1e6b19e511"}, { new NonTerminator(NonTerminatorType.Variable), "ca7cefdc-b17f-4e84-8b42-829590b1110e"}, { new Terminator(DelimiterType.LeftParenthesis), "9019af5d-8a33-44d4-954c-d8f218b3ec5e"}, { Terminator.IdentifierTerminator, "f0064b59-541c-4e17-b719-937423bb36c5"}, { new Terminator(KeywordType.Not), "6196e383-c5cd-4279-9cbb-85bd6bdc1de4"}, { new Terminator(OperatorType.Minus), "323aa85f-eb14-4398-a5a6-e62e621aa1c2"},}, new Dictionary<Terminator, ReduceInformation>{ }, "76d184c8-f3c5-4f2c-ad43-0fd59d75a2a7") },
|
|
{ "2661dbe7-08ca-4463-8892-ffdc1673793c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "875f3b07-14ea-4cc5-87c8-94ee7d2d0eeb"}, { Terminator.NumberTerminator, "a94e0a8a-3c31-4b45-b757-8a1e6b19e511"}, { new NonTerminator(NonTerminatorType.Variable), "ca7cefdc-b17f-4e84-8b42-829590b1110e"}, { new Terminator(DelimiterType.LeftParenthesis), "9019af5d-8a33-44d4-954c-d8f218b3ec5e"}, { Terminator.IdentifierTerminator, "f0064b59-541c-4e17-b719-937423bb36c5"}, { new Terminator(KeywordType.Not), "6196e383-c5cd-4279-9cbb-85bd6bdc1de4"}, { new Terminator(OperatorType.Minus), "323aa85f-eb14-4398-a5a6-e62e621aa1c2"},}, new Dictionary<Terminator, ReduceInformation>{ }, "2661dbe7-08ca-4463-8892-ffdc1673793c") },
|
|
{ "0874f0b7-49ef-427a-b0c4-d12a0d5c1a9b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "5b4b078e-5e44-4eca-9ef0-43450b2c2277"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0874f0b7-49ef-427a-b0c4-d12a0d5c1a9b") },
|
|
{ "c4ff5ec8-5acf-4a99-9762-32cf249cbadc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "90399067-fa55-4c60-9310-0ae6d71a9cc3"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c4ff5ec8-5acf-4a99-9762-32cf249cbadc") },
|
|
{ "c26a9885-06bd-4ffe-87dd-c8872257b2dc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "c26a9885-06bd-4ffe-87dd-c8872257b2dc") },
|
|
{ "38ed7061-3592-4788-b5da-1f6869b6d716", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "73fc5922-076e-459a-95c7-40c6e6a5eae8"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "38ed7061-3592-4788-b5da-1f6869b6d716") },
|
|
{ "3ff56b4c-9b39-447d-b705-f69f52b22398", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "3ff56b4c-9b39-447d-b705-f69f52b22398") },
|
|
{ "43857d72-45ac-4b06-9cd5-da183e56897f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "43857d72-45ac-4b06-9cd5-da183e56897f") },
|
|
{ "f445b0cf-2c90-4e6e-8da7-63d6423333f4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "f445b0cf-2c90-4e6e-8da7-63d6423333f4") },
|
|
{ "50138a80-e45e-4501-a646-80513240b0c0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "50138a80-e45e-4501-a646-80513240b0c0") },
|
|
{ "e46147c9-003b-46cb-9997-24feeb2ca815", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "c03b755e-3abd-46b4-a7e4-29ccec010909"}, { new NonTerminator(NonTerminatorType.Factor), "51f72fb0-d985-4bc6-b47a-9ea0753d7907"}, { Terminator.NumberTerminator, "13ca9c1b-760f-4da0-878b-72b23c8a6a55"}, { new NonTerminator(NonTerminatorType.Variable), "35529643-d0ce-4527-ad73-841e6868903b"}, { new Terminator(DelimiterType.LeftParenthesis), "9b07659a-c168-4c5b-8781-b6c11ea204a0"}, { Terminator.IdentifierTerminator, "df0def68-2aa5-49b6-8869-9e2d30b4c48d"}, { new Terminator(KeywordType.Not), "fec38012-07b5-4ba8-ae88-a68a714f1cbc"}, { new Terminator(OperatorType.Minus), "727581eb-84d2-411d-9872-e367a0ccc78c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e46147c9-003b-46cb-9997-24feeb2ca815") },
|
|
{ "49ff32c8-99bc-4459-9f3f-33612a86266a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "375f7217-1f72-45b4-82ac-711f5615da6b"}, { Terminator.NumberTerminator, "13ca9c1b-760f-4da0-878b-72b23c8a6a55"}, { new NonTerminator(NonTerminatorType.Variable), "35529643-d0ce-4527-ad73-841e6868903b"}, { new Terminator(DelimiterType.LeftParenthesis), "9b07659a-c168-4c5b-8781-b6c11ea204a0"}, { Terminator.IdentifierTerminator, "df0def68-2aa5-49b6-8869-9e2d30b4c48d"}, { new Terminator(KeywordType.Not), "fec38012-07b5-4ba8-ae88-a68a714f1cbc"}, { new Terminator(OperatorType.Minus), "727581eb-84d2-411d-9872-e367a0ccc78c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "49ff32c8-99bc-4459-9f3f-33612a86266a") },
|
|
{ "d67fe0fd-82e0-4693-a7bd-35d11394462b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "a43f13cd-fa8e-44cf-99f7-6ab78917c5cc"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d67fe0fd-82e0-4693-a7bd-35d11394462b") },
|
|
{ "d3aecd1f-ce29-4754-a1ad-7d3e19ed6753", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "e94fff91-a23d-4230-9b0e-2d2a5df7e56c"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d3aecd1f-ce29-4754-a1ad-7d3e19ed6753") },
|
|
{ "beea9760-bdb9-4e65-86ed-3a90de4c9b65", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "beea9760-bdb9-4e65-86ed-3a90de4c9b65") },
|
|
{ "840c3d0e-b902-4cb8-a5e2-109ea93e1775", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "addd1a42-5cb6-4af5-9c34-75702e727c61"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "840c3d0e-b902-4cb8-a5e2-109ea93e1775") },
|
|
{ "e0e375a3-617f-459c-a805-f9079e5003a8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "e0e375a3-617f-459c-a805-f9079e5003a8") },
|
|
{ "c0520c10-db88-4872-ab61-6920951067b9", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "c0520c10-db88-4872-ab61-6920951067b9") },
|
|
{ "730ac0f0-9ffb-4894-92f0-459e7a0488be", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "730ac0f0-9ffb-4894-92f0-459e7a0488be") },
|
|
{ "cfb76852-2969-4a72-b98f-22405390bc96", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "cfb76852-2969-4a72-b98f-22405390bc96") },
|
|
{ "a309e6b0-3089-48de-b8c3-168b663f3c14", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "12996497-f8f0-487e-a986-4faf26a412fc"}, { new NonTerminator(NonTerminatorType.Factor), "f0d0fcd0-1d8a-405f-9354-a994b496f73d"}, { Terminator.NumberTerminator, "3ffc269a-694c-4697-9e31-73f752ab9d1f"}, { new NonTerminator(NonTerminatorType.Variable), "188a892f-e2b1-465e-85e1-67451cbfe493"}, { new Terminator(DelimiterType.LeftParenthesis), "29f01b0d-b7fb-46f9-9522-5c1dbec0b06c"}, { Terminator.IdentifierTerminator, "8306e662-fca1-48d9-bedf-bd4bc289518c"}, { new Terminator(KeywordType.Not), "393677af-7726-490c-9f16-7587445de9f6"}, { new Terminator(OperatorType.Minus), "653e16fa-8ba0-4f39-8e14-063ba0fcc359"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a309e6b0-3089-48de-b8c3-168b663f3c14") },
|
|
{ "73a2f83c-7090-409b-92cf-40f354be432c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "54b6b697-8095-4963-a92e-979bdd69f240"}, { Terminator.NumberTerminator, "3ffc269a-694c-4697-9e31-73f752ab9d1f"}, { new NonTerminator(NonTerminatorType.Variable), "188a892f-e2b1-465e-85e1-67451cbfe493"}, { new Terminator(DelimiterType.LeftParenthesis), "29f01b0d-b7fb-46f9-9522-5c1dbec0b06c"}, { Terminator.IdentifierTerminator, "8306e662-fca1-48d9-bedf-bd4bc289518c"}, { new Terminator(KeywordType.Not), "393677af-7726-490c-9f16-7587445de9f6"}, { new Terminator(OperatorType.Minus), "653e16fa-8ba0-4f39-8e14-063ba0fcc359"},}, new Dictionary<Terminator, ReduceInformation>{ }, "73a2f83c-7090-409b-92cf-40f354be432c") },
|
|
{ "fd1b66b4-6a27-4c1e-92d0-02331e7332c5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "48411b02-dd58-43cd-ba96-8e43edc14d9f"},}, new Dictionary<Terminator, ReduceInformation>{ }, "fd1b66b4-6a27-4c1e-92d0-02331e7332c5") },
|
|
{ "5e5cb6ae-e0d0-44e8-9309-8111917a2ca4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "912cba3e-b8ae-44fd-8555-09649067280d"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5e5cb6ae-e0d0-44e8-9309-8111917a2ca4") },
|
|
{ "6668df6d-36ea-4f84-831f-60257550db2d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "6668df6d-36ea-4f84-831f-60257550db2d") },
|
|
{ "842797a0-655c-4fd4-8300-fe1f30f1842c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "4c990798-e543-4628-8c4f-e4fd5e8fac82"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "842797a0-655c-4fd4-8300-fe1f30f1842c") },
|
|
{ "1b2e99a6-f206-4af6-a9f5-13c590b6f3a6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "1b2e99a6-f206-4af6-a9f5-13c590b6f3a6") },
|
|
{ "afdf0327-a331-4543-8f0b-b5f54f38505c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "afdf0327-a331-4543-8f0b-b5f54f38505c") },
|
|
{ "5e50fd88-93bf-4757-9ef1-4b8269eb2172", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "5e50fd88-93bf-4757-9ef1-4b8269eb2172") },
|
|
{ "045382bc-9dfb-4b0d-a6e2-325681ac646a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "045382bc-9dfb-4b0d-a6e2-325681ac646a") },
|
|
{ "3a004d15-8f52-4adf-8832-53d0ed1c8429", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ElsePart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ElsePart))}, }, "3a004d15-8f52-4adf-8832-53d0ed1c8429") },
|
|
{ "fa653631-6014-4fad-a34d-ae04caa6e03b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, }, "fa653631-6014-4fad-a34d-ae04caa6e03b") },
|
|
{ "f64f7d06-6e83-4b4a-afa4-12d820344a4a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "8b26efb2-6dc6-40ab-9dd9-69cce78e87dc"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "bc175d2d-ffb8-43d8-a25d-9e7a8e8f7de4"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "f64f7d06-6e83-4b4a-afa4-12d820344a4a") },
|
|
{ "140aa1c3-e73d-4c29-802a-e241fda55485", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "5049da86-b0f5-4f86-9380-7a1e03c0e85a"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "140aa1c3-e73d-4c29-802a-e241fda55485") },
|
|
{ "5f286aeb-87b1-4cd2-8227-dacae01f5851", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "5f286aeb-87b1-4cd2-8227-dacae01f5851") },
|
|
{ "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4") },
|
|
{ "d8bb860c-d107-4a56-96fb-7e7e70063536", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "d8bb860c-d107-4a56-96fb-7e7e70063536") },
|
|
{ "33fd746c-385d-4bb0-9e51-902f3d247131", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "a6422eec-d723-42b5-9893-d24495d886eb"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "33fd746c-385d-4bb0-9e51-902f3d247131") },
|
|
{ "152863ec-9b47-42ca-8fee-391ace4b9f5e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "a61591c6-7c29-4357-8982-9368097cb288"}, { new NonTerminator(NonTerminatorType.IdVarPart), "33a4241e-4918-4f4f-8572-aaa1dd967254"}, { new Terminator(DelimiterType.LeftSquareBracket), "b9a5dc96-4f6b-44d7-924e-e1a373f3bc01"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Else), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "152863ec-9b47-42ca-8fee-391ace4b9f5e") },
|
|
{ "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "3c9368ec-9988-4be8-a072-9e88afaea56d"}, { Terminator.NumberTerminator, "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4"}, { new NonTerminator(NonTerminatorType.Variable), "d8bb860c-d107-4a56-96fb-7e7e70063536"}, { new Terminator(DelimiterType.LeftParenthesis), "33fd746c-385d-4bb0-9e51-902f3d247131"}, { Terminator.IdentifierTerminator, "152863ec-9b47-42ca-8fee-391ace4b9f5e"}, { new Terminator(KeywordType.Not), "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae"}, { new Terminator(OperatorType.Minus), "3db16667-f163-45df-a53a-a2b4c37906fb"},}, new Dictionary<Terminator, ReduceInformation>{ }, "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae") },
|
|
{ "3db16667-f163-45df-a53a-a2b4c37906fb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "3d0a1402-9860-48e4-87ea-ca3f32bbc322"}, { Terminator.NumberTerminator, "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4"}, { new NonTerminator(NonTerminatorType.Variable), "d8bb860c-d107-4a56-96fb-7e7e70063536"}, { new Terminator(DelimiterType.LeftParenthesis), "33fd746c-385d-4bb0-9e51-902f3d247131"}, { Terminator.IdentifierTerminator, "152863ec-9b47-42ca-8fee-391ace4b9f5e"}, { new Terminator(KeywordType.Not), "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae"}, { new Terminator(OperatorType.Minus), "3db16667-f163-45df-a53a-a2b4c37906fb"},}, new Dictionary<Terminator, ReduceInformation>{ }, "3db16667-f163-45df-a53a-a2b4c37906fb") },
|
|
{ "3065f96f-b964-4da0-a1e2-051c00df4238", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Statement))}, }, "3065f96f-b964-4da0-a1e2-051c00df4238") },
|
|
{ "1aac137b-8eba-4ca3-8652-3d230112e2a0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "5ea72771-54ad-48d9-b4fa-bf8f15adb2b8"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1aac137b-8eba-4ca3-8652-3d230112e2a0") },
|
|
{ "b25d1052-dd35-4688-9a47-0507f51cfd6f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Statement), "59e83bc4-5a3a-44dc-8024-fd27f0c84a88"}, { new NonTerminator(NonTerminatorType.Variable), "95ec03de-0feb-40ef-8d99-6e17f1af43bc"}, { Terminator.IdentifierTerminator, "d5afeb9a-86c4-4038-b0cc-cddf89462d0d"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "c99a68a9-af82-472a-b1a8-40f6398859c5"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "d2c7c905-6a01-48f9-a191-8b6e18062cce"}, { new Terminator(KeywordType.If), "197d696b-bb93-47c1-a2d6-32f6f58a2966"}, { new Terminator(KeywordType.For), "954dbde8-c20c-421b-b9af-3f71898d6f01"}, { new Terminator(KeywordType.Begin), "df0cac11-1ad1-4f31-87ee-0350ca6051f4"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "b25d1052-dd35-4688-9a47-0507f51cfd6f") },
|
|
{ "c6a9f364-68dd-4012-ab77-379f7603f641", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "f179d170-3c09-41d5-a9d0-867e0528ad2e"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bbdbc5e4-202b-4d40-a862-c9092cfb6c67"}, { new NonTerminator(NonTerminatorType.Term), "fe68835b-da88-4eda-940c-19f73c430df7"}, { new NonTerminator(NonTerminatorType.Factor), "3b214e0e-230c-481b-8c15-f304cc856f86"}, { Terminator.NumberTerminator, "6aa7ef72-0153-4ddf-8f6f-32e44c4c66c4"}, { new NonTerminator(NonTerminatorType.Variable), "f56343e4-f729-4764-b769-0e133ee3a2f2"}, { new Terminator(DelimiterType.LeftParenthesis), "cdf73a03-7454-4da9-942e-e01adf2d6592"}, { Terminator.IdentifierTerminator, "38247e93-f150-453a-b02a-809c7b22ef1d"}, { new Terminator(KeywordType.Not), "16b14840-8c2b-4856-a232-ebcc29c0f7a5"}, { new Terminator(OperatorType.Minus), "f8243a3e-9016-47fc-88a3-453d416dc854"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c6a9f364-68dd-4012-ab77-379f7603f641") },
|
|
{ "49a7e4fa-6e01-4023-907b-60286af9256a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.CompoundStatement))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.CompoundStatement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.CompoundStatement))}, }, "49a7e4fa-6e01-4023-907b-60286af9256a") },
|
|
{ "bd6b6663-2cce-483e-9d19-c61be1d729f3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "2c40c3e6-9e5d-4a04-9a03-d54fa03e9fd6"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "bd6b6663-2cce-483e-9d19-c61be1d729f3") },
|
|
{ "bb7afe54-cf30-4686-bcb5-9cc28aea6f05", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "bb7afe54-cf30-4686-bcb5-9cc28aea6f05") },
|
|
{ "8c7fb4d8-adbc-4a32-88e8-fa21d0091d18", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "8c7fb4d8-adbc-4a32-88e8-fa21d0091d18") },
|
|
{ "a8bed40a-3e8e-4852-81b4-203f2c0f31f4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "3fa4a97c-ceff-4907-9619-e2b09647f276"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a8bed40a-3e8e-4852-81b4-203f2c0f31f4") },
|
|
{ "392887a7-7346-422b-842c-6b02c94db7aa", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "3ec26952-3a3b-48f0-a986-31936626b333"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "392887a7-7346-422b-842c-6b02c94db7aa") },
|
|
{ "0d952e95-ce57-44c2-846a-170950662e9a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "5f4efd42-9412-4187-a6c0-dd718cbfefa4"}, { new NonTerminator(NonTerminatorType.Factor), "41c2af4d-09f5-4b9b-9451-8cc42419d432"}, { Terminator.NumberTerminator, "2a86e144-9c92-4853-81a5-12888293d62f"}, { new NonTerminator(NonTerminatorType.Variable), "a7f71a4b-2095-4f79-a06f-6cdee4b7d59e"}, { new Terminator(DelimiterType.LeftParenthesis), "f881ba2a-5a64-4a41-beaa-22cb3030308a"}, { Terminator.IdentifierTerminator, "0f896225-a2c2-40c8-ba03-f80886ec77eb"}, { new Terminator(KeywordType.Not), "7dd7e043-b608-405e-99f9-7a031816ff16"}, { new Terminator(OperatorType.Minus), "c46d6d12-9c82-4d65-ae5a-b973857c1a90"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0d952e95-ce57-44c2-846a-170950662e9a") },
|
|
{ "7b21201b-8255-4261-9742-587d3c0a4bc6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "29fc62da-0290-4cc3-8558-905b7f27f851"}, { Terminator.NumberTerminator, "2a86e144-9c92-4853-81a5-12888293d62f"}, { new NonTerminator(NonTerminatorType.Variable), "a7f71a4b-2095-4f79-a06f-6cdee4b7d59e"}, { new Terminator(DelimiterType.LeftParenthesis), "f881ba2a-5a64-4a41-beaa-22cb3030308a"}, { Terminator.IdentifierTerminator, "0f896225-a2c2-40c8-ba03-f80886ec77eb"}, { new Terminator(KeywordType.Not), "7dd7e043-b608-405e-99f9-7a031816ff16"}, { new Terminator(OperatorType.Minus), "c46d6d12-9c82-4d65-ae5a-b973857c1a90"},}, new Dictionary<Terminator, ReduceInformation>{ }, "7b21201b-8255-4261-9742-587d3c0a4bc6") },
|
|
{ "27768c95-ff12-4fac-aa55-9c5b9e361de8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "f08a4df6-29a3-485d-aa00-b337f689ebba"},}, new Dictionary<Terminator, ReduceInformation>{ }, "27768c95-ff12-4fac-aa55-9c5b9e361de8") },
|
|
{ "e8a5d434-72a4-46e9-9bd9-d78af731ef73", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "18a38fb1-eb50-47ce-b084-ecf9b3fc680b"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e8a5d434-72a4-46e9-9bd9-d78af731ef73") },
|
|
{ "49e62c47-cb9e-44ca-9eef-b43dea6723d4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "49e62c47-cb9e-44ca-9eef-b43dea6723d4") },
|
|
{ "d46259bb-80c9-4ffd-9c24-9e7bae78add5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "d1fcd592-f6da-49a4-beaa-f3754456e3cd"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d46259bb-80c9-4ffd-9c24-9e7bae78add5") },
|
|
{ "39e72570-0e67-4611-bd9a-0470e815b89e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "39e72570-0e67-4611-bd9a-0470e815b89e") },
|
|
{ "8a01dda9-1cc2-4878-95c9-df49efe5b0b4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "8a01dda9-1cc2-4878-95c9-df49efe5b0b4") },
|
|
{ "fdbb53de-4335-4d3e-b784-3b7c1b262625", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "fdbb53de-4335-4d3e-b784-3b7c1b262625") },
|
|
{ "1491bbfe-601a-4191-b45a-1bb10d7d18d5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "1491bbfe-601a-4191-b45a-1bb10d7d18d5") },
|
|
{ "4f2176aa-ff6e-477c-a812-a0b2c3a1d64c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.Do), "956c51d3-6720-44fb-a244-38fa83a1bcd5"},}, new Dictionary<Terminator, ReduceInformation>{ }, "4f2176aa-ff6e-477c-a812-a0b2c3a1d64c") },
|
|
{ "80dcc69e-4b6c-47a7-806d-2d6042789558", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.RelationOperator), "15eac16c-c4fb-4679-a19b-ab11aaf86300"}, { new Terminator(OperatorType.Equal), "79a105dd-26a8-48e1-a1e5-574c75949b4d"}, { new Terminator(OperatorType.NotEqual), "6afacd0d-b338-4375-8311-1af9477b2050"}, { new Terminator(OperatorType.Less), "b19156b3-e778-413d-b25a-ae00fc21ab09"}, { new Terminator(OperatorType.LessEqual), "583c85b9-f1c6-454a-9d8a-9954789412ec"}, { new Terminator(OperatorType.Greater), "74e622b4-8fc3-49a2-992b-87c0d048f09a"}, { new Terminator(OperatorType.GreaterEqual), "5db54d7e-231b-43b7-be0f-bb3021e1915a"}, { new NonTerminator(NonTerminatorType.AddOperator), "70f42b09-8fc8-4b33-81c8-5436d40d0eea"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Expression))}, }, "80dcc69e-4b6c-47a7-806d-2d6042789558") },
|
|
{ "b6f0cf51-dc0f-42b9-b4be-bf32b9a5a91a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "0259badf-01fd-4c0a-b6d9-20d229f4918e"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "b6f0cf51-dc0f-42b9-b4be-bf32b9a5a91a") },
|
|
{ "fb30bf0b-a6da-4520-bbf4-f7bde327e205", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "fb30bf0b-a6da-4520-bbf4-f7bde327e205") },
|
|
{ "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927") },
|
|
{ "3e0807fb-f778-41ce-bc91-57646ba8a4fd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "3e0807fb-f778-41ce-bc91-57646ba8a4fd") },
|
|
{ "92c717a5-062e-4ee9-8b6b-83979d1b8f55", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "6d7cc5f1-5413-4e2e-a410-882f13f2629c"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "92c717a5-062e-4ee9-8b6b-83979d1b8f55") },
|
|
{ "c0348957-d7d9-46c4-8066-60e86de6a120", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "dba227ae-ef37-4eb5-b7fe-49a47039724e"}, { new NonTerminator(NonTerminatorType.IdVarPart), "3bacc071-b000-4652-81d6-81517f1563a2"}, { new Terminator(DelimiterType.LeftSquareBracket), "5fa21881-4d9b-48be-bacb-aadfba7b6bb0"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "c0348957-d7d9-46c4-8066-60e86de6a120") },
|
|
{ "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "695088aa-fb51-4354-a469-8ac6b042786e"}, { Terminator.NumberTerminator, "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927"}, { new NonTerminator(NonTerminatorType.Variable), "3e0807fb-f778-41ce-bc91-57646ba8a4fd"}, { new Terminator(DelimiterType.LeftParenthesis), "92c717a5-062e-4ee9-8b6b-83979d1b8f55"}, { Terminator.IdentifierTerminator, "c0348957-d7d9-46c4-8066-60e86de6a120"}, { new Terminator(KeywordType.Not), "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81"}, { new Terminator(OperatorType.Minus), "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81") },
|
|
{ "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "31f75e24-88f8-47d7-b464-309a3a996b2c"}, { Terminator.NumberTerminator, "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927"}, { new NonTerminator(NonTerminatorType.Variable), "3e0807fb-f778-41ce-bc91-57646ba8a4fd"}, { new Terminator(DelimiterType.LeftParenthesis), "92c717a5-062e-4ee9-8b6b-83979d1b8f55"}, { Terminator.IdentifierTerminator, "c0348957-d7d9-46c4-8066-60e86de6a120"}, { new Terminator(KeywordType.Not), "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81"}, { new Terminator(OperatorType.Minus), "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4") },
|
|
{ "5bf62974-385a-46c4-ab7f-efef13e98440", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "e063676b-3978-4bd8-afa4-50cf441c4c67"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "5bf62974-385a-46c4-ab7f-efef13e98440") },
|
|
{ "750206ac-afc5-4a52-bba3-ac748d93cf32", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "c237e1e4-fe17-4ba2-9dd8-e154792b9510"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "750206ac-afc5-4a52-bba3-ac748d93cf32") },
|
|
{ "a69cd9ad-c893-4b4f-be74-0fc3cd420ddb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "a69cd9ad-c893-4b4f-be74-0fc3cd420ddb") },
|
|
{ "c1cac270-eb37-4944-a5f2-4fbf46250915", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "c1cac270-eb37-4944-a5f2-4fbf46250915") },
|
|
{ "7f8b9161-eadb-41c1-bdf3-449db72c2486", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "7f8b9161-eadb-41c1-bdf3-449db72c2486") },
|
|
{ "8858a3cf-c573-42ff-9f6f-1c14e693c759", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "d66633be-9cf6-4037-8d2e-04d112c71de1"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8858a3cf-c573-42ff-9f6f-1c14e693c759") },
|
|
{ "8f80a93b-1f9c-4827-851d-25e5bce2798b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "06f98484-94f3-479e-a617-154c4a2358bb"}, { new NonTerminator(NonTerminatorType.IdVarPart), "298ba6c5-ccc1-47cc-89be-e599edf5f9e7"}, { new Terminator(DelimiterType.LeftSquareBracket), "50045f85-2963-418e-8847-77b49eb88ef0"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "8f80a93b-1f9c-4827-851d-25e5bce2798b") },
|
|
{ "c8e9b2a6-afee-4f2e-9646-74cbba6b2f65", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "2c0c74cc-fcc2-4e4f-939a-c01c965ac4b8"}, { Terminator.NumberTerminator, "c1cac270-eb37-4944-a5f2-4fbf46250915"}, { new NonTerminator(NonTerminatorType.Variable), "7f8b9161-eadb-41c1-bdf3-449db72c2486"}, { new Terminator(DelimiterType.LeftParenthesis), "8858a3cf-c573-42ff-9f6f-1c14e693c759"}, { Terminator.IdentifierTerminator, "8f80a93b-1f9c-4827-851d-25e5bce2798b"}, { new Terminator(KeywordType.Not), "c8e9b2a6-afee-4f2e-9646-74cbba6b2f65"}, { new Terminator(OperatorType.Minus), "b1eb6db3-a55a-4399-a287-469148a5eef3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c8e9b2a6-afee-4f2e-9646-74cbba6b2f65") },
|
|
{ "b1eb6db3-a55a-4399-a287-469148a5eef3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "c7f69d0e-8e95-4b17-bdf9-3c6917f36c94"}, { Terminator.NumberTerminator, "c1cac270-eb37-4944-a5f2-4fbf46250915"}, { new NonTerminator(NonTerminatorType.Variable), "7f8b9161-eadb-41c1-bdf3-449db72c2486"}, { new Terminator(DelimiterType.LeftParenthesis), "8858a3cf-c573-42ff-9f6f-1c14e693c759"}, { Terminator.IdentifierTerminator, "8f80a93b-1f9c-4827-851d-25e5bce2798b"}, { new Terminator(KeywordType.Not), "c8e9b2a6-afee-4f2e-9646-74cbba6b2f65"}, { new Terminator(OperatorType.Minus), "b1eb6db3-a55a-4399-a287-469148a5eef3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b1eb6db3-a55a-4399-a287-469148a5eef3") },
|
|
{ "02e11df1-da73-441a-92b5-8057844e68a3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "4415fae3-3f18-49e9-a5cd-26c4a00bcaf2"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "02e11df1-da73-441a-92b5-8057844e68a3") },
|
|
{ "7f26b8c0-ea67-477b-84e6-343ffd2923dd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "7f26b8c0-ea67-477b-84e6-343ffd2923dd") },
|
|
{ "0c17baef-2b4b-450e-b245-5cd843ef405f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "0c17baef-2b4b-450e-b245-5cd843ef405f") },
|
|
{ "77fc79a0-c31e-48df-bd18-0ea14a034ba1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "6c84079c-312e-4ec3-89cb-5b3e3a4cb05f"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "77fc79a0-c31e-48df-bd18-0ea14a034ba1") },
|
|
{ "fe879b03-0748-4f76-b081-9196cf3a5684", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "739f14c8-d204-459c-b273-59c26f16eaf0"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "fe879b03-0748-4f76-b081-9196cf3a5684") },
|
|
{ "359234a1-0f34-4d0f-b65b-e6b4b5aa7a8a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.CompoundStatement))}, }, "359234a1-0f34-4d0f-b65b-e6b4b5aa7a8a") },
|
|
{ "52d8892a-4e66-443a-90c7-e78d08bc6940", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.Semicolon), new ReduceInformation(6, new NonTerminator(NonTerminatorType.Type))}, }, "52d8892a-4e66-443a-90c7-e78d08bc6940") },
|
|
{ "5019768d-6250-47ae-a7b6-d22b6ee1fbea", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { Terminator.NumberTerminator, "6ae799f6-e200-4e1e-80a6-63012afbe11e"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5019768d-6250-47ae-a7b6-d22b6ee1fbea") },
|
|
{ "cd3c2c4c-b44a-47e2-b2af-183065962f24", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "2661dbe7-08ca-4463-8892-ffdc1673793c"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "cd3c2c4c-b44a-47e2-b2af-183065962f24") },
|
|
{ "875f3b07-14ea-4cc5-87c8-94ee7d2d0eeb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "875f3b07-14ea-4cc5-87c8-94ee7d2d0eeb") },
|
|
{ "5b4b078e-5e44-4eca-9ef0-43450b2c2277", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "5b4b078e-5e44-4eca-9ef0-43450b2c2277") },
|
|
{ "90399067-fa55-4c60-9310-0ae6d71a9cc3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "80156c31-946f-4145-b473-c08578a6884b"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "90399067-fa55-4c60-9310-0ae6d71a9cc3") },
|
|
{ "73fc5922-076e-459a-95c7-40c6e6a5eae8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "80c77bb2-d52b-4cc5-865b-badd669139bd"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "73fc5922-076e-459a-95c7-40c6e6a5eae8") },
|
|
{ "c03b755e-3abd-46b4-a7e4-29ccec010909", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "49ff32c8-99bc-4459-9f3f-33612a86266a"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "c03b755e-3abd-46b4-a7e4-29ccec010909") },
|
|
{ "375f7217-1f72-45b4-82ac-711f5615da6b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "375f7217-1f72-45b4-82ac-711f5615da6b") },
|
|
{ "a43f13cd-fa8e-44cf-99f7-6ab78917c5cc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "a43f13cd-fa8e-44cf-99f7-6ab78917c5cc") },
|
|
{ "e94fff91-a23d-4230-9b0e-2d2a5df7e56c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "439e6dd4-35cb-4aa1-9a14-c6af2d162fb5"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e94fff91-a23d-4230-9b0e-2d2a5df7e56c") },
|
|
{ "addd1a42-5cb6-4af5-9c34-75702e727c61", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "1cd0d960-86d2-4836-bc6b-ee26f9da131d"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "addd1a42-5cb6-4af5-9c34-75702e727c61") },
|
|
{ "12996497-f8f0-487e-a986-4faf26a412fc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "73a2f83c-7090-409b-92cf-40f354be432c"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "12996497-f8f0-487e-a986-4faf26a412fc") },
|
|
{ "54b6b697-8095-4963-a92e-979bdd69f240", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "54b6b697-8095-4963-a92e-979bdd69f240") },
|
|
{ "48411b02-dd58-43cd-ba96-8e43edc14d9f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "48411b02-dd58-43cd-ba96-8e43edc14d9f") },
|
|
{ "912cba3e-b8ae-44fd-8555-09649067280d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "be5fa7ea-3898-458b-8414-d15e1bd7b471"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "912cba3e-b8ae-44fd-8555-09649067280d") },
|
|
{ "4c990798-e543-4628-8c4f-e4fd5e8fac82", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "96be577d-b281-454b-b3d0-6c67389de16c"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "4c990798-e543-4628-8c4f-e4fd5e8fac82") },
|
|
{ "8b26efb2-6dc6-40ab-9dd9-69cce78e87dc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "a596c765-8c74-49d9-ab2c-21b9abf71d90"}, { new NonTerminator(NonTerminatorType.Term), "f79a770a-7f61-47b4-9021-6b76d1871e8e"}, { new NonTerminator(NonTerminatorType.Factor), "91800229-168f-43c1-b19a-dad9ccbde5f2"}, { Terminator.NumberTerminator, "0042b889-9997-4d9d-9612-85aa7438bb07"}, { new NonTerminator(NonTerminatorType.Variable), "377da628-4e39-4e40-8e5d-ee3be2ec6bee"}, { new Terminator(DelimiterType.LeftParenthesis), "18747c8a-6860-4efe-8b22-6e2f5cb71f75"}, { Terminator.IdentifierTerminator, "77bf9832-3c9c-4aa9-bef2-3c6692ff14f6"}, { new Terminator(KeywordType.Not), "2043bcb8-f4be-4ab8-8e89-987533aa517f"}, { new Terminator(OperatorType.Minus), "9171b745-205a-44c3-b56a-19a3dc3a9a13"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8b26efb2-6dc6-40ab-9dd9-69cce78e87dc") },
|
|
{ "bc175d2d-ffb8-43d8-a25d-9e7a8e8f7de4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "680a7fb3-b0cb-4527-8708-556f19d9c2cf"}, { new NonTerminator(NonTerminatorType.Factor), "5f286aeb-87b1-4cd2-8227-dacae01f5851"}, { Terminator.NumberTerminator, "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4"}, { new NonTerminator(NonTerminatorType.Variable), "d8bb860c-d107-4a56-96fb-7e7e70063536"}, { new Terminator(DelimiterType.LeftParenthesis), "33fd746c-385d-4bb0-9e51-902f3d247131"}, { Terminator.IdentifierTerminator, "152863ec-9b47-42ca-8fee-391ace4b9f5e"}, { new Terminator(KeywordType.Not), "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae"}, { new Terminator(OperatorType.Minus), "3db16667-f163-45df-a53a-a2b4c37906fb"},}, new Dictionary<Terminator, ReduceInformation>{ }, "bc175d2d-ffb8-43d8-a25d-9e7a8e8f7de4") },
|
|
{ "5049da86-b0f5-4f86-9380-7a1e03c0e85a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "795e941f-b35d-43ed-bdeb-991078802a93"}, { Terminator.NumberTerminator, "3e55b29f-4acb-4c26-b6c4-3c42f39fd6c4"}, { new NonTerminator(NonTerminatorType.Variable), "d8bb860c-d107-4a56-96fb-7e7e70063536"}, { new Terminator(DelimiterType.LeftParenthesis), "33fd746c-385d-4bb0-9e51-902f3d247131"}, { Terminator.IdentifierTerminator, "152863ec-9b47-42ca-8fee-391ace4b9f5e"}, { new Terminator(KeywordType.Not), "52cd0a52-9224-4fe6-aceb-aa63fe5ffcae"}, { new Terminator(OperatorType.Minus), "3db16667-f163-45df-a53a-a2b4c37906fb"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5049da86-b0f5-4f86-9380-7a1e03c0e85a") },
|
|
{ "a6422eec-d723-42b5-9893-d24495d886eb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "a642c2bb-461e-4644-a7cb-e7e6c7a87023"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a6422eec-d723-42b5-9893-d24495d886eb") },
|
|
{ "a61591c6-7c29-4357-8982-9368097cb288", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "45e06bac-2cdd-4be7-bf42-818701f21eea"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a61591c6-7c29-4357-8982-9368097cb288") },
|
|
{ "33a4241e-4918-4f4f-8572-aaa1dd967254", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Else), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "33a4241e-4918-4f4f-8572-aaa1dd967254") },
|
|
{ "b9a5dc96-4f6b-44d7-924e-e1a373f3bc01", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "0f9a8f5a-8ffa-4f98-9422-6ea0cc9380cd"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b9a5dc96-4f6b-44d7-924e-e1a373f3bc01") },
|
|
{ "3c9368ec-9988-4be8-a072-9e88afaea56d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "3c9368ec-9988-4be8-a072-9e88afaea56d") },
|
|
{ "3d0a1402-9860-48e4-87ea-ca3f32bbc322", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "3d0a1402-9860-48e4-87ea-ca3f32bbc322") },
|
|
{ "5ea72771-54ad-48d9-b4fa-bf8f15adb2b8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(4, new NonTerminator(NonTerminatorType.ProcedureCall))}, { new Terminator(KeywordType.Else), new ReduceInformation(4, new NonTerminator(NonTerminatorType.ProcedureCall))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(4, new NonTerminator(NonTerminatorType.ProcedureCall))}, }, "5ea72771-54ad-48d9-b4fa-bf8f15adb2b8") },
|
|
{ "59e83bc4-5a3a-44dc-8024-fd27f0c84a88", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ElsePart), "b345044b-ec06-471c-ab9c-d2a319b4bfa6"}, { new Terminator(KeywordType.Else), "febdd0bb-f3eb-401c-87ac-158688625ea8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ElsePart))}, { new Terminator(KeywordType.Else), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ElsePart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.ElsePart))}, }, "59e83bc4-5a3a-44dc-8024-fd27f0c84a88") },
|
|
{ "f179d170-3c09-41d5-a9d0-867e0528ad2e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.To), "538d6402-8d40-41a2-a3ae-0e4c4e9459b7"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f179d170-3c09-41d5-a9d0-867e0528ad2e") },
|
|
{ "3fa4a97c-ceff-4907-9619-e2b09647f276", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "3fa4a97c-ceff-4907-9619-e2b09647f276") },
|
|
{ "3ec26952-3a3b-48f0-a986-31936626b333", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Then), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "3ec26952-3a3b-48f0-a986-31936626b333") },
|
|
{ "5f4efd42-9412-4187-a6c0-dd718cbfefa4", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "7b21201b-8255-4261-9742-587d3c0a4bc6"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "5f4efd42-9412-4187-a6c0-dd718cbfefa4") },
|
|
{ "29fc62da-0290-4cc3-8558-905b7f27f851", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "29fc62da-0290-4cc3-8558-905b7f27f851") },
|
|
{ "f08a4df6-29a3-485d-aa00-b337f689ebba", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "f08a4df6-29a3-485d-aa00-b337f689ebba") },
|
|
{ "18a38fb1-eb50-47ce-b084-ecf9b3fc680b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "4f19c2ae-f60a-46de-9a3c-c3374d0f37f2"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "18a38fb1-eb50-47ce-b084-ecf9b3fc680b") },
|
|
{ "d1fcd592-f6da-49a4-beaa-f3754456e3cd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "b848f0dd-2b49-43f0-9314-04282c4b4535"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d1fcd592-f6da-49a4-beaa-f3754456e3cd") },
|
|
{ "956c51d3-6720-44fb-a244-38fa83a1bcd5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Statement), "f4f8b84e-1ded-4bdf-a5d1-1d78dc624e61"}, { new NonTerminator(NonTerminatorType.Variable), "5c5d5be7-e10a-42b4-9004-5d2bdf5e7db1"}, { Terminator.IdentifierTerminator, "2103d893-c079-4b22-81d8-4f9f358fe6ad"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "e53241b0-bb50-48d3-845a-063d9ecbbdeb"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "81235c65-a9b7-4f3f-bce2-4c97f0d9db5a"}, { new Terminator(KeywordType.If), "c9145487-1b31-498c-9722-1f68bba31eab"}, { new Terminator(KeywordType.For), "d93a638a-474b-4cbd-93db-107fb792750f"}, { new Terminator(KeywordType.Begin), "bbe1d91e-d0c2-4be2-8184-4548587afa0e"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "956c51d3-6720-44fb-a244-38fa83a1bcd5") },
|
|
{ "15eac16c-c4fb-4679-a19b-ab11aaf86300", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.SimpleExpression), "5d7444e5-c266-443a-98ae-196b25d25aa0"}, { new NonTerminator(NonTerminatorType.Term), "868bae7f-161d-45ae-adb8-e57b46542d67"}, { new NonTerminator(NonTerminatorType.Factor), "72442c47-ce68-41f2-8b8c-4ba48451f95f"}, { Terminator.NumberTerminator, "5402355f-0062-4a4a-b209-4ac1c4b37304"}, { new NonTerminator(NonTerminatorType.Variable), "5fa31e4e-9080-49ad-806e-c5a218a0fa3c"}, { new Terminator(DelimiterType.LeftParenthesis), "f574482c-fe85-4132-bdff-b68aa7dc8f7e"}, { Terminator.IdentifierTerminator, "f060aa86-c858-4f9a-96cb-70d2573dd235"}, { new Terminator(KeywordType.Not), "b280b5a1-2a5f-4b4c-ade1-359067ea8e96"}, { new Terminator(OperatorType.Minus), "4867a9b8-8fde-4539-96c3-509d02964d33"},}, new Dictionary<Terminator, ReduceInformation>{ }, "15eac16c-c4fb-4679-a19b-ab11aaf86300") },
|
|
{ "70f42b09-8fc8-4b33-81c8-5436d40d0eea", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "cd53a578-2af6-4d63-990c-b20914ac856f"}, { new NonTerminator(NonTerminatorType.Factor), "fb30bf0b-a6da-4520-bbf4-f7bde327e205"}, { Terminator.NumberTerminator, "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927"}, { new NonTerminator(NonTerminatorType.Variable), "3e0807fb-f778-41ce-bc91-57646ba8a4fd"}, { new Terminator(DelimiterType.LeftParenthesis), "92c717a5-062e-4ee9-8b6b-83979d1b8f55"}, { Terminator.IdentifierTerminator, "c0348957-d7d9-46c4-8066-60e86de6a120"}, { new Terminator(KeywordType.Not), "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81"}, { new Terminator(OperatorType.Minus), "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4"},}, new Dictionary<Terminator, ReduceInformation>{ }, "70f42b09-8fc8-4b33-81c8-5436d40d0eea") },
|
|
{ "0259badf-01fd-4c0a-b6d9-20d229f4918e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "6917d284-453a-4e6f-8dcb-c911b0d4e1e8"}, { Terminator.NumberTerminator, "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927"}, { new NonTerminator(NonTerminatorType.Variable), "3e0807fb-f778-41ce-bc91-57646ba8a4fd"}, { new Terminator(DelimiterType.LeftParenthesis), "92c717a5-062e-4ee9-8b6b-83979d1b8f55"}, { Terminator.IdentifierTerminator, "c0348957-d7d9-46c4-8066-60e86de6a120"}, { new Terminator(KeywordType.Not), "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81"}, { new Terminator(OperatorType.Minus), "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0259badf-01fd-4c0a-b6d9-20d229f4918e") },
|
|
{ "6d7cc5f1-5413-4e2e-a410-882f13f2629c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "2d89dfc5-7daa-456f-82dc-da4844037807"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6d7cc5f1-5413-4e2e-a410-882f13f2629c") },
|
|
{ "dba227ae-ef37-4eb5-b7fe-49a47039724e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "1fabe222-0acd-465e-b5e7-e8b20472a120"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "dba227ae-ef37-4eb5-b7fe-49a47039724e") },
|
|
{ "3bacc071-b000-4652-81d6-81517f1563a2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "3bacc071-b000-4652-81d6-81517f1563a2") },
|
|
{ "5fa21881-4d9b-48be-bacb-aadfba7b6bb0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "a4f28cf9-bfc2-42cc-bc35-ee88cea248ae"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5fa21881-4d9b-48be-bacb-aadfba7b6bb0") },
|
|
{ "695088aa-fb51-4354-a469-8ac6b042786e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "695088aa-fb51-4354-a469-8ac6b042786e") },
|
|
{ "31f75e24-88f8-47d7-b464-309a3a996b2c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "31f75e24-88f8-47d7-b464-309a3a996b2c") },
|
|
{ "e063676b-3978-4bd8-afa4-50cf441c4c67", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "7a050248-f085-40cc-89e6-ac6a99e2a878"}, { new NonTerminator(NonTerminatorType.Factor), "a69cd9ad-c893-4b4f-be74-0fc3cd420ddb"}, { Terminator.NumberTerminator, "c1cac270-eb37-4944-a5f2-4fbf46250915"}, { new NonTerminator(NonTerminatorType.Variable), "7f8b9161-eadb-41c1-bdf3-449db72c2486"}, { new Terminator(DelimiterType.LeftParenthesis), "8858a3cf-c573-42ff-9f6f-1c14e693c759"}, { Terminator.IdentifierTerminator, "8f80a93b-1f9c-4827-851d-25e5bce2798b"}, { new Terminator(KeywordType.Not), "c8e9b2a6-afee-4f2e-9646-74cbba6b2f65"}, { new Terminator(OperatorType.Minus), "b1eb6db3-a55a-4399-a287-469148a5eef3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e063676b-3978-4bd8-afa4-50cf441c4c67") },
|
|
{ "c237e1e4-fe17-4ba2-9dd8-e154792b9510", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "fb3fef8a-2499-4262-9d03-4346d812c7cf"}, { Terminator.NumberTerminator, "c1cac270-eb37-4944-a5f2-4fbf46250915"}, { new NonTerminator(NonTerminatorType.Variable), "7f8b9161-eadb-41c1-bdf3-449db72c2486"}, { new Terminator(DelimiterType.LeftParenthesis), "8858a3cf-c573-42ff-9f6f-1c14e693c759"}, { Terminator.IdentifierTerminator, "8f80a93b-1f9c-4827-851d-25e5bce2798b"}, { new Terminator(KeywordType.Not), "c8e9b2a6-afee-4f2e-9646-74cbba6b2f65"}, { new Terminator(OperatorType.Minus), "b1eb6db3-a55a-4399-a287-469148a5eef3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c237e1e4-fe17-4ba2-9dd8-e154792b9510") },
|
|
{ "d66633be-9cf6-4037-8d2e-04d112c71de1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "69cf6f48-4426-4f67-9446-1cabb79aac69"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d66633be-9cf6-4037-8d2e-04d112c71de1") },
|
|
{ "06f98484-94f3-479e-a617-154c4a2358bb", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "e8b6d4fe-7707-4393-8393-b0973e71c37e"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "06f98484-94f3-479e-a617-154c4a2358bb") },
|
|
{ "298ba6c5-ccc1-47cc-89be-e599edf5f9e7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "298ba6c5-ccc1-47cc-89be-e599edf5f9e7") },
|
|
{ "50045f85-2963-418e-8847-77b49eb88ef0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "e67b3d7f-17ca-4a4c-ab04-ba4342e3c8cc"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "50045f85-2963-418e-8847-77b49eb88ef0") },
|
|
{ "2c0c74cc-fcc2-4e4f-939a-c01c965ac4b8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "2c0c74cc-fcc2-4e4f-939a-c01c965ac4b8") },
|
|
{ "c7f69d0e-8e95-4b17-bdf9-3c6917f36c94", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "c7f69d0e-8e95-4b17-bdf9-3c6917f36c94") },
|
|
{ "6c84079c-312e-4ec3-89cb-5b3e3a4cb05f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "6c84079c-312e-4ec3-89cb-5b3e3a4cb05f") },
|
|
{ "739f14c8-d204-459c-b273-59c26f16eaf0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "739f14c8-d204-459c-b273-59c26f16eaf0") },
|
|
{ "6ae799f6-e200-4e1e-80a6-63012afbe11e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(5, new NonTerminator(NonTerminatorType.Period))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(5, new NonTerminator(NonTerminatorType.Period))}, }, "6ae799f6-e200-4e1e-80a6-63012afbe11e") },
|
|
{ "80156c31-946f-4145-b473-c08578a6884b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "80156c31-946f-4145-b473-c08578a6884b") },
|
|
{ "80c77bb2-d52b-4cc5-865b-badd669139bd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "80c77bb2-d52b-4cc5-865b-badd669139bd") },
|
|
{ "439e6dd4-35cb-4aa1-9a14-c6af2d162fb5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "439e6dd4-35cb-4aa1-9a14-c6af2d162fb5") },
|
|
{ "1cd0d960-86d2-4836-bc6b-ee26f9da131d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightSquareBracket), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "1cd0d960-86d2-4836-bc6b-ee26f9da131d") },
|
|
{ "be5fa7ea-3898-458b-8414-d15e1bd7b471", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "be5fa7ea-3898-458b-8414-d15e1bd7b471") },
|
|
{ "96be577d-b281-454b-b3d0-6c67389de16c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Comma), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "96be577d-b281-454b-b3d0-6c67389de16c") },
|
|
{ "a596c765-8c74-49d9-ab2c-21b9abf71d90", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "8cb31ed7-f32d-42bb-aa99-5d43c3acbf8e"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "a596c765-8c74-49d9-ab2c-21b9abf71d90") },
|
|
{ "f79a770a-7f61-47b4-9021-6b76d1871e8e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "feebebb1-2ec2-49ff-a991-e82b198c09fc"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "f79a770a-7f61-47b4-9021-6b76d1871e8e") },
|
|
{ "91800229-168f-43c1-b19a-dad9ccbde5f2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "91800229-168f-43c1-b19a-dad9ccbde5f2") },
|
|
{ "0042b889-9997-4d9d-9612-85aa7438bb07", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "0042b889-9997-4d9d-9612-85aa7438bb07") },
|
|
{ "377da628-4e39-4e40-8e5d-ee3be2ec6bee", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "377da628-4e39-4e40-8e5d-ee3be2ec6bee") },
|
|
{ "18747c8a-6860-4efe-8b22-6e2f5cb71f75", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "1deba651-7205-498b-8dd5-0bdbfacd2ee1"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "18747c8a-6860-4efe-8b22-6e2f5cb71f75") },
|
|
{ "77bf9832-3c9c-4aa9-bef2-3c6692ff14f6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "5c1b1295-6f7e-4858-9c8d-7fbbd27a62cc"}, { new NonTerminator(NonTerminatorType.IdVarPart), "52b8cef8-df59-4222-a82a-70db6253759e"}, { new Terminator(DelimiterType.LeftSquareBracket), "c10fdeaf-866f-40fb-8384-418941e16a5a"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Else), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "77bf9832-3c9c-4aa9-bef2-3c6692ff14f6") },
|
|
{ "2043bcb8-f4be-4ab8-8e89-987533aa517f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "78313254-fe02-47a9-a5d3-c100c71e49d6"}, { Terminator.NumberTerminator, "0042b889-9997-4d9d-9612-85aa7438bb07"}, { new NonTerminator(NonTerminatorType.Variable), "377da628-4e39-4e40-8e5d-ee3be2ec6bee"}, { new Terminator(DelimiterType.LeftParenthesis), "18747c8a-6860-4efe-8b22-6e2f5cb71f75"}, { Terminator.IdentifierTerminator, "77bf9832-3c9c-4aa9-bef2-3c6692ff14f6"}, { new Terminator(KeywordType.Not), "2043bcb8-f4be-4ab8-8e89-987533aa517f"}, { new Terminator(OperatorType.Minus), "9171b745-205a-44c3-b56a-19a3dc3a9a13"},}, new Dictionary<Terminator, ReduceInformation>{ }, "2043bcb8-f4be-4ab8-8e89-987533aa517f") },
|
|
{ "9171b745-205a-44c3-b56a-19a3dc3a9a13", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "836cbcc6-e775-4bf8-888f-13c5bb5c4fb0"}, { Terminator.NumberTerminator, "0042b889-9997-4d9d-9612-85aa7438bb07"}, { new NonTerminator(NonTerminatorType.Variable), "377da628-4e39-4e40-8e5d-ee3be2ec6bee"}, { new Terminator(DelimiterType.LeftParenthesis), "18747c8a-6860-4efe-8b22-6e2f5cb71f75"}, { Terminator.IdentifierTerminator, "77bf9832-3c9c-4aa9-bef2-3c6692ff14f6"}, { new Terminator(KeywordType.Not), "2043bcb8-f4be-4ab8-8e89-987533aa517f"}, { new Terminator(OperatorType.Minus), "9171b745-205a-44c3-b56a-19a3dc3a9a13"},}, new Dictionary<Terminator, ReduceInformation>{ }, "9171b745-205a-44c3-b56a-19a3dc3a9a13") },
|
|
{ "680a7fb3-b0cb-4527-8708-556f19d9c2cf", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "5049da86-b0f5-4f86-9380-7a1e03c0e85a"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "680a7fb3-b0cb-4527-8708-556f19d9c2cf") },
|
|
{ "795e941f-b35d-43ed-bdeb-991078802a93", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "795e941f-b35d-43ed-bdeb-991078802a93") },
|
|
{ "a642c2bb-461e-4644-a7cb-e7e6c7a87023", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "a642c2bb-461e-4644-a7cb-e7e6c7a87023") },
|
|
{ "45e06bac-2cdd-4be7-bf42-818701f21eea", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "f500fc21-0d4b-4f32-86e4-967f123c5a85"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "45e06bac-2cdd-4be7-bf42-818701f21eea") },
|
|
{ "0f9a8f5a-8ffa-4f98-9422-6ea0cc9380cd", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "276a89a6-1c75-4b43-b90a-312ae6ecadc2"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0f9a8f5a-8ffa-4f98-9422-6ea0cc9380cd") },
|
|
{ "b345044b-ec06-471c-ab9c-d2a319b4bfa6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(5, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(5, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(5, new NonTerminator(NonTerminatorType.Statement))}, }, "b345044b-ec06-471c-ab9c-d2a319b4bfa6") },
|
|
{ "febdd0bb-f3eb-401c-87ac-158688625ea8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Statement), "a2b29025-d892-4c57-818e-901ec87b61ed"}, { new NonTerminator(NonTerminatorType.Variable), "95ec03de-0feb-40ef-8d99-6e17f1af43bc"}, { Terminator.IdentifierTerminator, "d5afeb9a-86c4-4038-b0cc-cddf89462d0d"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "c99a68a9-af82-472a-b1a8-40f6398859c5"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "d2c7c905-6a01-48f9-a191-8b6e18062cce"}, { new Terminator(KeywordType.If), "197d696b-bb93-47c1-a2d6-32f6f58a2966"}, { new Terminator(KeywordType.For), "954dbde8-c20c-421b-b9af-3f71898d6f01"}, { new Terminator(KeywordType.Begin), "df0cac11-1ad1-4f31-87ee-0350ca6051f4"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "febdd0bb-f3eb-401c-87ac-158688625ea8") },
|
|
{ "538d6402-8d40-41a2-a3ae-0e4c4e9459b7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "bc3fc288-61cb-4a44-9d81-e201d5813729"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "80dcc69e-4b6c-47a7-806d-2d6042789558"}, { new NonTerminator(NonTerminatorType.Term), "b6f0cf51-dc0f-42b9-b4be-bf32b9a5a91a"}, { new NonTerminator(NonTerminatorType.Factor), "fb30bf0b-a6da-4520-bbf4-f7bde327e205"}, { Terminator.NumberTerminator, "6fd8ebbb-a32d-4fdd-ad27-0443de8ba927"}, { new NonTerminator(NonTerminatorType.Variable), "3e0807fb-f778-41ce-bc91-57646ba8a4fd"}, { new Terminator(DelimiterType.LeftParenthesis), "92c717a5-062e-4ee9-8b6b-83979d1b8f55"}, { Terminator.IdentifierTerminator, "c0348957-d7d9-46c4-8066-60e86de6a120"}, { new Terminator(KeywordType.Not), "d0b89d30-6b10-4cac-8ecd-0d8c8bb7bc81"}, { new Terminator(OperatorType.Minus), "1fc9d0de-5bd1-4138-9d9a-8c09c03f7bc4"},}, new Dictionary<Terminator, ReduceInformation>{ }, "538d6402-8d40-41a2-a3ae-0e4c4e9459b7") },
|
|
{ "4f19c2ae-f60a-46de-9a3c-c3374d0f37f2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "4f19c2ae-f60a-46de-9a3c-c3374d0f37f2") },
|
|
{ "b848f0dd-2b49-43f0-9314-04282c4b4535", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(DelimiterType.RightParenthesis), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "b848f0dd-2b49-43f0-9314-04282c4b4535") },
|
|
{ "f4f8b84e-1ded-4bdf-a5d1-1d78dc624e61", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(8, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(8, new NonTerminator(NonTerminatorType.Statement))}, }, "f4f8b84e-1ded-4bdf-a5d1-1d78dc624e61") },
|
|
{ "5d7444e5-c266-443a-98ae-196b25d25aa0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.AddOperator), "65c2eac7-e640-47da-9d47-b7e0b7805b92"}, { new Terminator(OperatorType.Plus), "a6970a4b-bf6b-45c3-9f5f-cd30a01aa981"}, { new Terminator(OperatorType.Minus), "521c4a92-4030-40b7-a6af-9b8ec1f4358f"}, { new Terminator(KeywordType.Or), "273a6a36-3c91-4795-b9b6-8884d837b5a8"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Expression))}, }, "5d7444e5-c266-443a-98ae-196b25d25aa0") },
|
|
{ "868bae7f-161d-45ae-adb8-e57b46542d67", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "d5c81d88-1671-437f-bca0-5940229ce244"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "868bae7f-161d-45ae-adb8-e57b46542d67") },
|
|
{ "72442c47-ce68-41f2-8b8c-4ba48451f95f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Term))}, }, "72442c47-ce68-41f2-8b8c-4ba48451f95f") },
|
|
{ "5402355f-0062-4a4a-b209-4ac1c4b37304", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "5402355f-0062-4a4a-b209-4ac1c4b37304") },
|
|
{ "5fa31e4e-9080-49ad-806e-c5a218a0fa3c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(1, new NonTerminator(NonTerminatorType.Factor))}, }, "5fa31e4e-9080-49ad-806e-c5a218a0fa3c") },
|
|
{ "f574482c-fe85-4132-bdff-b68aa7dc8f7e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Expression), "843bd08e-6cee-4a78-a09b-14f60c53dd5c"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "0f6db587-6cd7-4ecc-8651-c6d50006f10a"}, { new NonTerminator(NonTerminatorType.Term), "29ba3a00-e922-4557-afb9-6f5d3609d6ee"}, { new NonTerminator(NonTerminatorType.Factor), "b92f0307-2eb4-4bd0-afe0-ecb3463e44b2"}, { Terminator.NumberTerminator, "c7d186b5-6791-4dc0-b8ac-22ec8b5d3749"}, { new NonTerminator(NonTerminatorType.Variable), "27067d65-a3e8-4670-b353-2f4a7abd2ab7"}, { new Terminator(DelimiterType.LeftParenthesis), "cb7a9eca-2c00-4aa1-b40d-d9c025922749"}, { Terminator.IdentifierTerminator, "5022f026-8866-40ab-8bd7-91eade276b7d"}, { new Terminator(KeywordType.Not), "1ad9b6c2-2a76-4dc8-8aa9-1a43fa7fddc3"}, { new Terminator(OperatorType.Minus), "8c33c722-e4b7-43e5-b416-eac9ae0200b3"},}, new Dictionary<Terminator, ReduceInformation>{ }, "f574482c-fe85-4132-bdff-b68aa7dc8f7e") },
|
|
{ "f060aa86-c858-4f9a-96cb-70d2573dd235", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.LeftParenthesis), "0c477775-87b8-4a39-9920-8e665ee1b257"}, { new NonTerminator(NonTerminatorType.IdVarPart), "39724f7b-a0fd-4d27-8694-514cedc69469"}, { new Terminator(DelimiterType.LeftSquareBracket), "395efe1e-5d37-4418-9b27-7ab607643d82"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(0, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "f060aa86-c858-4f9a-96cb-70d2573dd235") },
|
|
{ "b280b5a1-2a5f-4b4c-ade1-359067ea8e96", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "20960e3e-f412-4cf4-845b-ce095309f68a"}, { Terminator.NumberTerminator, "5402355f-0062-4a4a-b209-4ac1c4b37304"}, { new NonTerminator(NonTerminatorType.Variable), "5fa31e4e-9080-49ad-806e-c5a218a0fa3c"}, { new Terminator(DelimiterType.LeftParenthesis), "f574482c-fe85-4132-bdff-b68aa7dc8f7e"}, { Terminator.IdentifierTerminator, "f060aa86-c858-4f9a-96cb-70d2573dd235"}, { new Terminator(KeywordType.Not), "b280b5a1-2a5f-4b4c-ade1-359067ea8e96"}, { new Terminator(OperatorType.Minus), "4867a9b8-8fde-4539-96c3-509d02964d33"},}, new Dictionary<Terminator, ReduceInformation>{ }, "b280b5a1-2a5f-4b4c-ade1-359067ea8e96") },
|
|
{ "4867a9b8-8fde-4539-96c3-509d02964d33", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "22be2db8-0124-4bfe-870e-2297903a98d7"}, { Terminator.NumberTerminator, "5402355f-0062-4a4a-b209-4ac1c4b37304"}, { new NonTerminator(NonTerminatorType.Variable), "5fa31e4e-9080-49ad-806e-c5a218a0fa3c"}, { new Terminator(DelimiterType.LeftParenthesis), "f574482c-fe85-4132-bdff-b68aa7dc8f7e"}, { Terminator.IdentifierTerminator, "f060aa86-c858-4f9a-96cb-70d2573dd235"}, { new Terminator(KeywordType.Not), "b280b5a1-2a5f-4b4c-ade1-359067ea8e96"}, { new Terminator(OperatorType.Minus), "4867a9b8-8fde-4539-96c3-509d02964d33"},}, new Dictionary<Terminator, ReduceInformation>{ }, "4867a9b8-8fde-4539-96c3-509d02964d33") },
|
|
{ "cd53a578-2af6-4d63-990c-b20914ac856f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "0259badf-01fd-4c0a-b6d9-20d229f4918e"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "cd53a578-2af6-4d63-990c-b20914ac856f") },
|
|
{ "6917d284-453a-4e6f-8dcb-c911b0d4e1e8", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "6917d284-453a-4e6f-8dcb-c911b0d4e1e8") },
|
|
{ "2d89dfc5-7daa-456f-82dc-da4844037807", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "2d89dfc5-7daa-456f-82dc-da4844037807") },
|
|
{ "1fabe222-0acd-465e-b5e7-e8b20472a120", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "84616e6a-fa1a-4aa5-9803-d966753a721b"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1fabe222-0acd-465e-b5e7-e8b20472a120") },
|
|
{ "a4f28cf9-bfc2-42cc-bc35-ee88cea248ae", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "e5103668-8d7a-41fa-9a43-9ea53229f97d"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "a4f28cf9-bfc2-42cc-bc35-ee88cea248ae") },
|
|
{ "7a050248-f085-40cc-89e6-ac6a99e2a878", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "c237e1e4-fe17-4ba2-9dd8-e154792b9510"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "7a050248-f085-40cc-89e6-ac6a99e2a878") },
|
|
{ "fb3fef8a-2499-4262-9d03-4346d812c7cf", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "fb3fef8a-2499-4262-9d03-4346d812c7cf") },
|
|
{ "69cf6f48-4426-4f67-9446-1cabb79aac69", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "69cf6f48-4426-4f67-9446-1cabb79aac69") },
|
|
{ "e8b6d4fe-7707-4393-8393-b0973e71c37e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "b5b770b9-8aeb-4495-923e-4eea24336d3d"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e8b6d4fe-7707-4393-8393-b0973e71c37e") },
|
|
{ "e67b3d7f-17ca-4a4c-ab04-ba4342e3c8cc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "8e5bddab-acd8-4ee5-82d3-4ee611b25c4f"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "e67b3d7f-17ca-4a4c-ab04-ba4342e3c8cc") },
|
|
{ "8cb31ed7-f32d-42bb-aa99-5d43c3acbf8e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "773de252-3083-4110-9d6d-0bf87068818e"}, { new NonTerminator(NonTerminatorType.Factor), "91800229-168f-43c1-b19a-dad9ccbde5f2"}, { Terminator.NumberTerminator, "0042b889-9997-4d9d-9612-85aa7438bb07"}, { new NonTerminator(NonTerminatorType.Variable), "377da628-4e39-4e40-8e5d-ee3be2ec6bee"}, { new Terminator(DelimiterType.LeftParenthesis), "18747c8a-6860-4efe-8b22-6e2f5cb71f75"}, { Terminator.IdentifierTerminator, "77bf9832-3c9c-4aa9-bef2-3c6692ff14f6"}, { new Terminator(KeywordType.Not), "2043bcb8-f4be-4ab8-8e89-987533aa517f"}, { new Terminator(OperatorType.Minus), "9171b745-205a-44c3-b56a-19a3dc3a9a13"},}, new Dictionary<Terminator, ReduceInformation>{ }, "8cb31ed7-f32d-42bb-aa99-5d43c3acbf8e") },
|
|
{ "feebebb1-2ec2-49ff-a991-e82b198c09fc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "b8576b6c-5a11-40f6-ab41-5448a891a573"}, { Terminator.NumberTerminator, "0042b889-9997-4d9d-9612-85aa7438bb07"}, { new NonTerminator(NonTerminatorType.Variable), "377da628-4e39-4e40-8e5d-ee3be2ec6bee"}, { new Terminator(DelimiterType.LeftParenthesis), "18747c8a-6860-4efe-8b22-6e2f5cb71f75"}, { Terminator.IdentifierTerminator, "77bf9832-3c9c-4aa9-bef2-3c6692ff14f6"}, { new Terminator(KeywordType.Not), "2043bcb8-f4be-4ab8-8e89-987533aa517f"}, { new Terminator(OperatorType.Minus), "9171b745-205a-44c3-b56a-19a3dc3a9a13"},}, new Dictionary<Terminator, ReduceInformation>{ }, "feebebb1-2ec2-49ff-a991-e82b198c09fc") },
|
|
{ "1deba651-7205-498b-8dd5-0bdbfacd2ee1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "0b301ba7-2381-4a95-97e5-b0dd2fb64c4c"},}, new Dictionary<Terminator, ReduceInformation>{ }, "1deba651-7205-498b-8dd5-0bdbfacd2ee1") },
|
|
{ "5c1b1295-6f7e-4858-9c8d-7fbbd27a62cc", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "70506fe8-041e-44d7-acac-2ed008323385"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "5c1b1295-6f7e-4858-9c8d-7fbbd27a62cc") },
|
|
{ "52b8cef8-df59-4222-a82a-70db6253759e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Else), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "52b8cef8-df59-4222-a82a-70db6253759e") },
|
|
{ "c10fdeaf-866f-40fb-8384-418941e16a5a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "80926d4a-2f53-4b70-a1aa-9fa74311e1a1"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "c10fdeaf-866f-40fb-8384-418941e16a5a") },
|
|
{ "78313254-fe02-47a9-a5d3-c100c71e49d6", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "78313254-fe02-47a9-a5d3-c100c71e49d6") },
|
|
{ "836cbcc6-e775-4bf8-888f-13c5bb5c4fb0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "836cbcc6-e775-4bf8-888f-13c5bb5c4fb0") },
|
|
{ "f500fc21-0d4b-4f32-86e4-967f123c5a85", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "f500fc21-0d4b-4f32-86e4-967f123c5a85") },
|
|
{ "276a89a6-1c75-4b43-b90a-312ae6ecadc2", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "276a89a6-1c75-4b43-b90a-312ae6ecadc2") },
|
|
{ "a2b29025-d892-4c57-818e-901ec87b61ed", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ElsePart))}, { new Terminator(KeywordType.Else), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ElsePart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(2, new NonTerminator(NonTerminatorType.ElsePart))}, }, "a2b29025-d892-4c57-818e-901ec87b61ed") },
|
|
{ "bc3fc288-61cb-4a44-9d81-e201d5813729", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(KeywordType.Do), "8708f553-f203-442a-98ea-0ccf53f8f941"},}, new Dictionary<Terminator, ReduceInformation>{ }, "bc3fc288-61cb-4a44-9d81-e201d5813729") },
|
|
{ "65c2eac7-e640-47da-9d47-b7e0b7805b92", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Term), "49d63827-c947-4011-81de-d0247de67522"}, { new NonTerminator(NonTerminatorType.Factor), "72442c47-ce68-41f2-8b8c-4ba48451f95f"}, { Terminator.NumberTerminator, "5402355f-0062-4a4a-b209-4ac1c4b37304"}, { new NonTerminator(NonTerminatorType.Variable), "5fa31e4e-9080-49ad-806e-c5a218a0fa3c"}, { new Terminator(DelimiterType.LeftParenthesis), "f574482c-fe85-4132-bdff-b68aa7dc8f7e"}, { Terminator.IdentifierTerminator, "f060aa86-c858-4f9a-96cb-70d2573dd235"}, { new Terminator(KeywordType.Not), "b280b5a1-2a5f-4b4c-ade1-359067ea8e96"}, { new Terminator(OperatorType.Minus), "4867a9b8-8fde-4539-96c3-509d02964d33"},}, new Dictionary<Terminator, ReduceInformation>{ }, "65c2eac7-e640-47da-9d47-b7e0b7805b92") },
|
|
{ "d5c81d88-1671-437f-bca0-5940229ce244", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Factor), "8b33be24-8cf8-454a-b78f-d3dc413d58d0"}, { Terminator.NumberTerminator, "5402355f-0062-4a4a-b209-4ac1c4b37304"}, { new NonTerminator(NonTerminatorType.Variable), "5fa31e4e-9080-49ad-806e-c5a218a0fa3c"}, { new Terminator(DelimiterType.LeftParenthesis), "f574482c-fe85-4132-bdff-b68aa7dc8f7e"}, { Terminator.IdentifierTerminator, "f060aa86-c858-4f9a-96cb-70d2573dd235"}, { new Terminator(KeywordType.Not), "b280b5a1-2a5f-4b4c-ade1-359067ea8e96"}, { new Terminator(OperatorType.Minus), "4867a9b8-8fde-4539-96c3-509d02964d33"},}, new Dictionary<Terminator, ReduceInformation>{ }, "d5c81d88-1671-437f-bca0-5940229ce244") },
|
|
{ "843bd08e-6cee-4a78-a09b-14f60c53dd5c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "87301840-f178-4134-b060-8608f0d3c323"},}, new Dictionary<Terminator, ReduceInformation>{ }, "843bd08e-6cee-4a78-a09b-14f60c53dd5c") },
|
|
{ "0c477775-87b8-4a39-9920-8e665ee1b257", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "96089d98-8cf6-4c8c-b7b3-1c87ea44d8e3"}, { new NonTerminator(NonTerminatorType.Expression), "09556f9b-3c83-477b-8464-0cab6d8e1bee"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "bfa871d2-bd57-4be4-bafb-8e5755cfd8ba"}, { new NonTerminator(NonTerminatorType.Term), "f2a35504-1720-4849-9ae3-8ec0c5b49854"}, { new NonTerminator(NonTerminatorType.Factor), "d2d61ae7-84e4-4609-96e1-336bc474312f"}, { Terminator.NumberTerminator, "aa92ed9e-dde5-4295-84e5-f047a7118d25"}, { new NonTerminator(NonTerminatorType.Variable), "372bc844-539e-4c2f-b103-bedf27599ff9"}, { new Terminator(DelimiterType.LeftParenthesis), "b8fcd8cc-351f-4a87-a689-54fa9ada9f5b"}, { Terminator.IdentifierTerminator, "2fda7058-df05-491d-a157-6b562f943743"}, { new Terminator(KeywordType.Not), "cfda5c15-06e0-47a7-a1db-8a5062493970"}, { new Terminator(OperatorType.Minus), "b39e46cf-fb12-40fa-84eb-da91399dd049"},}, new Dictionary<Terminator, ReduceInformation>{ }, "0c477775-87b8-4a39-9920-8e665ee1b257") },
|
|
{ "39724f7b-a0fd-4d27-8694-514cedc69469", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Variable))}, }, "39724f7b-a0fd-4d27-8694-514cedc69469") },
|
|
{ "395efe1e-5d37-4418-9b27-7ab607643d82", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.ExpressionList), "6430c8a4-e428-42a8-aaea-215c9f7c99a5"}, { new NonTerminator(NonTerminatorType.Expression), "443eb0a5-40d9-4b8e-b206-363e91ed8aae"}, { new NonTerminator(NonTerminatorType.SimpleExpression), "af315387-70e8-4940-9a8f-7f894721bee7"}, { new NonTerminator(NonTerminatorType.Term), "b2337dbe-963f-4559-a9a7-901b2f1496dc"}, { new NonTerminator(NonTerminatorType.Factor), "972ac3b8-6dc3-4503-8923-1acd997078da"}, { Terminator.NumberTerminator, "13afc88b-d09a-42c0-be5b-926f3299342e"}, { new NonTerminator(NonTerminatorType.Variable), "e990e4c7-637f-4348-8ca7-ae6641c98f5f"}, { new Terminator(DelimiterType.LeftParenthesis), "bf0093f2-da22-4e47-9df5-3502975ed106"}, { Terminator.IdentifierTerminator, "55349752-e804-4542-b1e6-db73c260479d"}, { new Terminator(KeywordType.Not), "f01f63f7-64d9-4726-8917-634575d1bd27"}, { new Terminator(OperatorType.Minus), "d0b41fc4-34c3-4b9e-a939-477279006d43"},}, new Dictionary<Terminator, ReduceInformation>{ }, "395efe1e-5d37-4418-9b27-7ab607643d82") },
|
|
{ "20960e3e-f412-4cf4-845b-ce095309f68a", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "20960e3e-f412-4cf4-845b-ce095309f68a") },
|
|
{ "22be2db8-0124-4bfe-870e-2297903a98d7", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(2, new NonTerminator(NonTerminatorType.Factor))}, }, "22be2db8-0124-4bfe-870e-2297903a98d7") },
|
|
{ "84616e6a-fa1a-4aa5-9803-d966753a721b", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Equal), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Less), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Greater), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "84616e6a-fa1a-4aa5-9803-d966753a721b") },
|
|
{ "e5103668-8d7a-41fa-9a43-9ea53229f97d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Equal), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.NotEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Less), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.LessEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Greater), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.GreaterEqual), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "e5103668-8d7a-41fa-9a43-9ea53229f97d") },
|
|
{ "b5b770b9-8aeb-4495-923e-4eea24336d3d", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "b5b770b9-8aeb-4495-923e-4eea24336d3d") },
|
|
{ "8e5bddab-acd8-4ee5-82d3-4ee611b25c4f", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.To), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "8e5bddab-acd8-4ee5-82d3-4ee611b25c4f") },
|
|
{ "773de252-3083-4110-9d6d-0bf87068818e", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "feebebb1-2ec2-49ff-a991-e82b198c09fc"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "773de252-3083-4110-9d6d-0bf87068818e") },
|
|
{ "b8576b6c-5a11-40f6-ab41-5448a891a573", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "b8576b6c-5a11-40f6-ab41-5448a891a573") },
|
|
{ "0b301ba7-2381-4a95-97e5-b0dd2fb64c4c", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "0b301ba7-2381-4a95-97e5-b0dd2fb64c4c") },
|
|
{ "70506fe8-041e-44d7-acac-2ed008323385", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "a2c2c1c2-61f4-4ee5-a4fb-d9bed4ecece5"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "70506fe8-041e-44d7-acac-2ed008323385") },
|
|
{ "80926d4a-2f53-4b70-a1aa-9fa74311e1a1", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "7d3c379a-21f2-4ddf-9ddb-58d8da0ab332"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "80926d4a-2f53-4b70-a1aa-9fa74311e1a1") },
|
|
{ "8708f553-f203-442a-98ea-0ccf53f8f941", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.Statement), "db17823b-0b6a-4a4b-bd03-b4186c18a983"}, { new NonTerminator(NonTerminatorType.Variable), "95ec03de-0feb-40ef-8d99-6e17f1af43bc"}, { Terminator.IdentifierTerminator, "d5afeb9a-86c4-4038-b0cc-cddf89462d0d"}, { new NonTerminator(NonTerminatorType.ProcedureCall), "c99a68a9-af82-472a-b1a8-40f6398859c5"}, { new NonTerminator(NonTerminatorType.CompoundStatement), "d2c7c905-6a01-48f9-a191-8b6e18062cce"}, { new Terminator(KeywordType.If), "197d696b-bb93-47c1-a2d6-32f6f58a2966"}, { new Terminator(KeywordType.For), "954dbde8-c20c-421b-b9af-3f71898d6f01"}, { new Terminator(KeywordType.Begin), "df0cac11-1ad1-4f31-87ee-0350ca6051f4"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(0, new NonTerminator(NonTerminatorType.Statement))}, }, "8708f553-f203-442a-98ea-0ccf53f8f941") },
|
|
{ "49d63827-c947-4011-81de-d0247de67522", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new NonTerminator(NonTerminatorType.MultiplyOperator), "d5c81d88-1671-437f-bca0-5940229ce244"}, { new Terminator(OperatorType.Multiply), "7896e50c-802f-4079-bc51-521da71c5940"}, { new Terminator(OperatorType.Divide), "97fc410f-cbd5-4c22-9d28-0d6d17f6f1d4"}, { new Terminator(KeywordType.Divide), "8c25ace4-2cdf-4493-8fc3-24c8110c9e53"}, { new Terminator(KeywordType.Mod), "9a9ed0c3-0d0b-4eb1-b014-252c1656ee28"}, { new Terminator(KeywordType.And), "19e2b148-c63e-4b68-9299-549db9054923"},}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.SimpleExpression))}, }, "49d63827-c947-4011-81de-d0247de67522") },
|
|
{ "8b33be24-8cf8-454a-b78f-d3dc413d58d0", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Term))}, }, "8b33be24-8cf8-454a-b78f-d3dc413d58d0") },
|
|
{ "87301840-f178-4134-b060-8608f0d3c323", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.Factor))}, }, "87301840-f178-4134-b060-8608f0d3c323") },
|
|
{ "96089d98-8cf6-4c8c-b7b3-1c87ea44d8e3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightParenthesis), "ddeae42c-0021-47aa-82a7-2fbe3a0eb142"}, { new Terminator(DelimiterType.Comma), "d1a7a2c6-9986-4552-a1a0-f0a6aed90aef"},}, new Dictionary<Terminator, ReduceInformation>{ }, "96089d98-8cf6-4c8c-b7b3-1c87ea44d8e3") },
|
|
{ "6430c8a4-e428-42a8-aaea-215c9f7c99a5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{ { new Terminator(DelimiterType.RightSquareBracket), "750ea419-69a2-490e-b1f3-528ed6d2c9d3"}, { new Terminator(DelimiterType.Comma), "b9ff9839-3a26-4a8c-9679-3f5636b81d03"},}, new Dictionary<Terminator, ReduceInformation>{ }, "6430c8a4-e428-42a8-aaea-215c9f7c99a5") },
|
|
{ "a2c2c1c2-61f4-4ee5-a4fb-d9bed4ecece5", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Else), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "a2c2c1c2-61f4-4ee5-a4fb-d9bed4ecece5") },
|
|
{ "7d3c379a-21f2-4ddf-9ddb-58d8da0ab332", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Else), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "7d3c379a-21f2-4ddf-9ddb-58d8da0ab332") },
|
|
{ "db17823b-0b6a-4a4b-bd03-b4186c18a983", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.End), new ReduceInformation(8, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(KeywordType.Else), new ReduceInformation(8, new NonTerminator(NonTerminatorType.Statement))}, { new Terminator(DelimiterType.Semicolon), new ReduceInformation(8, new NonTerminator(NonTerminatorType.Statement))}, }, "db17823b-0b6a-4a4b-bd03-b4186c18a983") },
|
|
{ "ddeae42c-0021-47aa-82a7-2fbe3a0eb142", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Divide), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Mod), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.And), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Plus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(OperatorType.Minus), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, { new Terminator(KeywordType.Or), new ReduceInformation(4, new NonTerminator(NonTerminatorType.Factor))}, }, "ddeae42c-0021-47aa-82a7-2fbe3a0eb142") },
|
|
{ "750ea419-69a2-490e-b1f3-528ed6d2c9d3", new GeneratedTransformer(new Dictionary<TerminatorBase, string>{}, new Dictionary<Terminator, ReduceInformation>{ { new Terminator(KeywordType.Do), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Multiply), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Divide), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Mod), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.And), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Plus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(OperatorType.Minus), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, { new Terminator(KeywordType.Or), new ReduceInformation(3, new NonTerminator(NonTerminatorType.IdVarPart))}, }, "750ea419-69a2-490e-b1f3-528ed6d2c9d3") },
|
|
};
|
|
|
|
private GeneratedGrammarParser()
|
|
{
|
|
foreach(GeneratedTransformer transformer in s_transformers.Values)
|
|
{
|
|
transformer.ConstructShiftTable(s_transformers);
|
|
}
|
|
}
|
|
|
|
private static GeneratedGrammarParser s_instance = new GeneratedGrammarParser();
|
|
|
|
public static GeneratedGrammarParser Instance => s_instance;
|
|
|
|
public ITransformer BeginTransformer => s_transformers["0ec30c00-e066-4e5e-a8b5-d0b86ce475ff"];
|
|
public NonTerminator Begin => new NonTerminator(NonTerminatorType.StartNonTerminator);
|
|
}
|